PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
ARRAYS:
An array is a variable with a single name capable of storing multiple values, each indexed by a number. Think of an array as a numbered list where elements are accessible by their respective numbers. Arrays are data structures capable of storing a fixed-size sequential collection of elements of the same type.
Arrays store fixed numbers of elements of the same data type sequentially in memory. An integer array holds a number of integers, a character array holds characters, and so forth. The size of an array is referred to as its dimension.
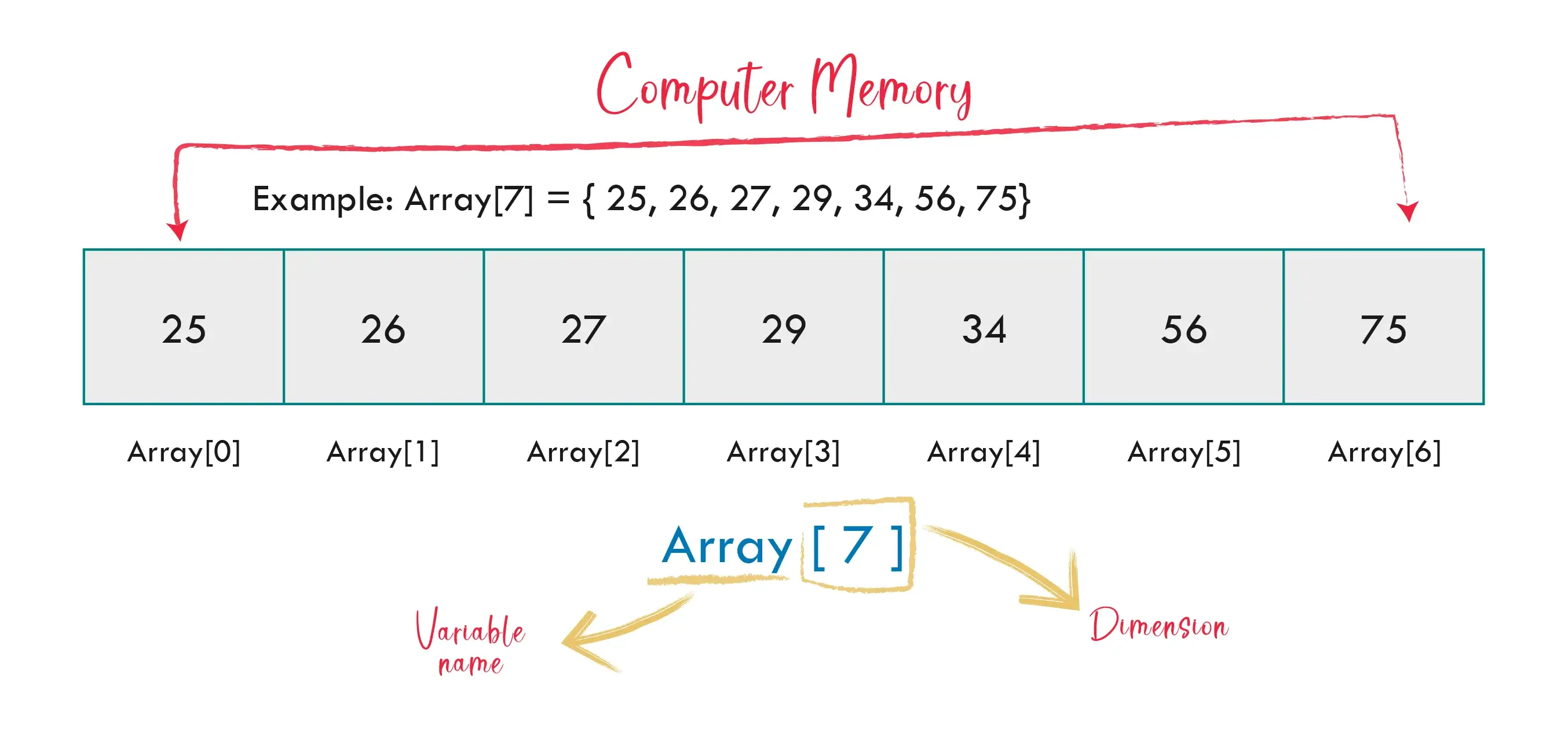
To illustrate arrays, let's consider an example where we store the results of 10 different students and display them using functions. We'll utilize two functions: getData()
to collect data from the user and showData()
to display the entered data.
#include <iostream>
#include <Windows.h> // For system("CLS") method
using namespace std;
void getData(); // Function prototype
void showData(); // Function prototype
// Global variables to store student data
int student0, student1, student2, student3, student4, student5,
student6, student7, student8, student9;
int main() {
getData(); // Call to get data from user
return 0;
}
// Function to get data from user
void getData() {
cout << "Enter Student Number: ";
cin >> student0;
cout << "Enter Student Number: ";
cin >> student1;
cout << "Enter Student Number: ";
cin >> student2;
cout << "Enter Student Number: ";
cin >> student3;
cout << "Enter Student Number: ";
cin >> student4;
cout << "Enter Student Number: ";
cin >> student5;
cout << "Enter Student Number: ";
cin >> student6;
cout << "Enter Student Number: ";
cin >> student7;
cout << "Enter Student Number: ";
cin >> student8;
cout << "Enter Student Number: ";
cin >> student9;
showData(); // Call to display entered data
}
// Function to display entered data
void showData() {
system("CLS"); // Clear the screen
// Display data for all students
cout << "Student 0) = " << student0 << endl;
cout << "Student 1) = " << student1 << endl;
cout << "Student 2) = " << student2 << endl;
cout << "Student 3) = " << student3 << endl;
cout << "Student 4) = " << student4 << endl;
cout << "Student 5) = " << student5 << endl;
cout << "Student 6) = " << student6 << endl;
cout << "Student 7) = " << student7 << endl;
cout << "Student 8) = " << student8 << endl;
cout << "Student 9) = " << student9 << endl;
}
system("CLS") is a built-in function known as "Clear-Screen" function used to clear the previous screen. It's defined in the "Window.h" header file.
This approach works but is repetitive and tedious, especially for a large number of students. Arrays offer a more efficient solution.
Let's rewrite the previous program using arrays.
#include <iostream>
#include <Windows.h>
using namespace std;
void getData(); // Function prototype
void showData(); // Function prototype
int student[10]; // Array declaration to store student marks
int main() {
getData(); // Call to get data from user
return 0;
}
// Function to get data from user
void getData() {
for(int i = 0; i < 10; i++) {
cout << "Enter Student Number: ";
cin >> student[i];
}
showData(); // Call to display entered data
}
// Function to display entered data
void showData() {
system("CLS"); // Clear the screen
// Display data for all students using loop
for(int i = 0; i < 10; i++) {
cout << "Student " << i << ") = " << student[i] << endl;
}
}
This approach using arrays is more concise and scalable, eliminating the need for individual variables for each student.
To declare an array, specify the data type, variable name, and size of the array. For example:
int element[10];
This declares an array named element capable of storing 10 integers.
Arrays can be initialized when defined. For example:
int element[10] = {10, 20, 30, 40, 50, 60, 70, 80, 90, 100};
We can also initialize elements one by one or using a loop.
Array elements are accessed by indexing the array name. For example:
int item = element[7];
This assigns the value of the 7th element of the array element to the variable item.
Arrays provide a convenient way to store and manipulate data of the same type. Remember that array indexing starts from 0, not 1, and always allocate memory with a buffer for expected usage. Arrays streamline programming tasks, especially when dealing with large datasets.
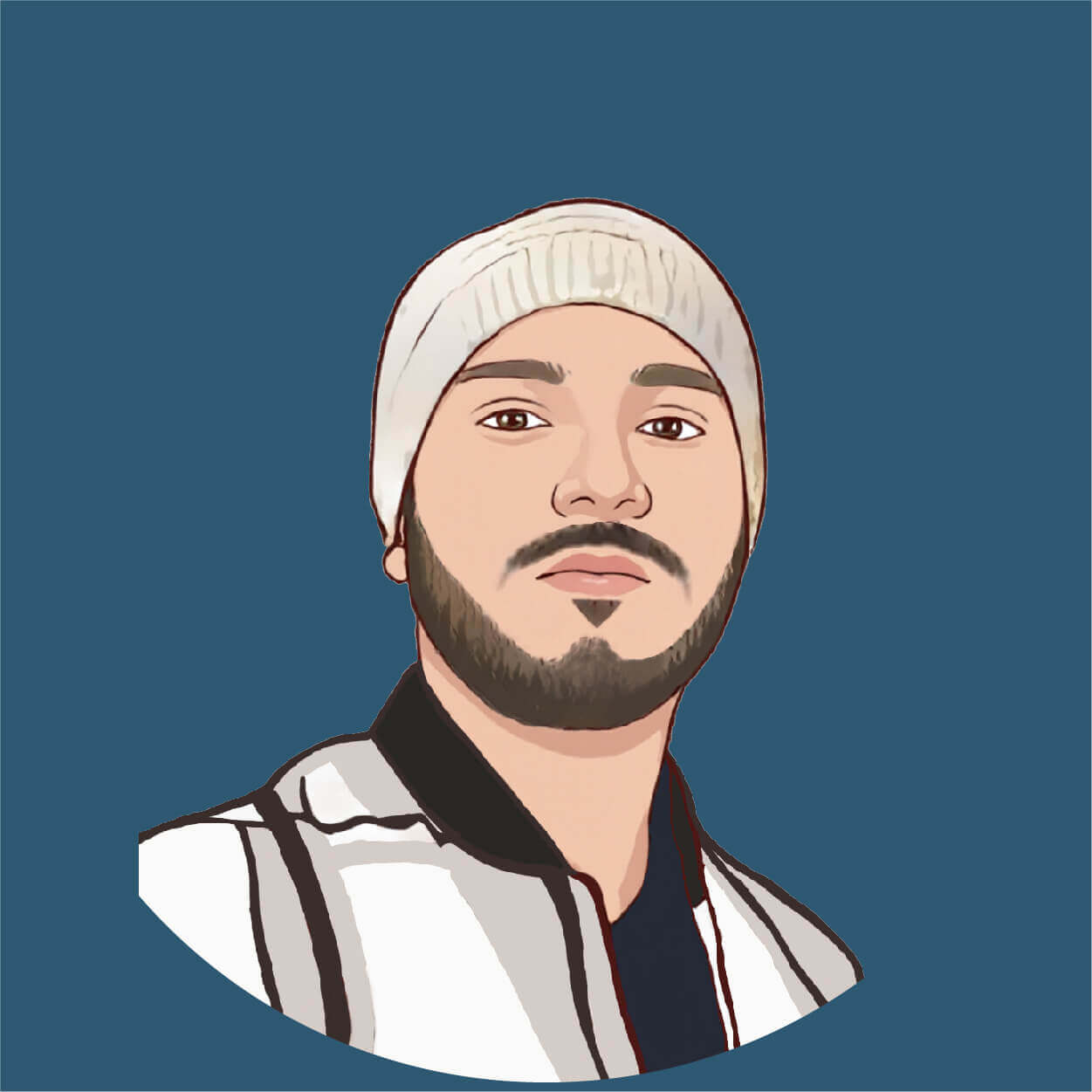
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.