PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
C++ Basic Input & Output:
The C++ Standard Libraries offer a robust set of Input/Output (I/O) capabilities, which will be explored in subsequent tutorials. This tutorial focuses on fundamental Common Input/Output operations crucial for C++ Programming.
C++ I/O revolves around streams, sequences of bytes:
- If bytes flow from a device (keyboard, disk drive, network connection) to main memory, it's an input operation.
- If bytes flow from main memory to a device (display screen, printer, disk drive, network connection), it's an output operation.
Several header files are integral to C++ programs, especially those dealing with basic input and output operations. Detailed discussions about libraries will be covered in the Library Tutorial.
Used for standard input stream, standard output stream, unbuffered standard error stream, and buffered standard error stream.
Declares functionality for performing formatted I/O with parameterized stream manipulators like setw and setprecision (covered in C++ ManipulatorsTutorial).
Declares services for user-controlled file processing (covered in Files OperationTutorial).
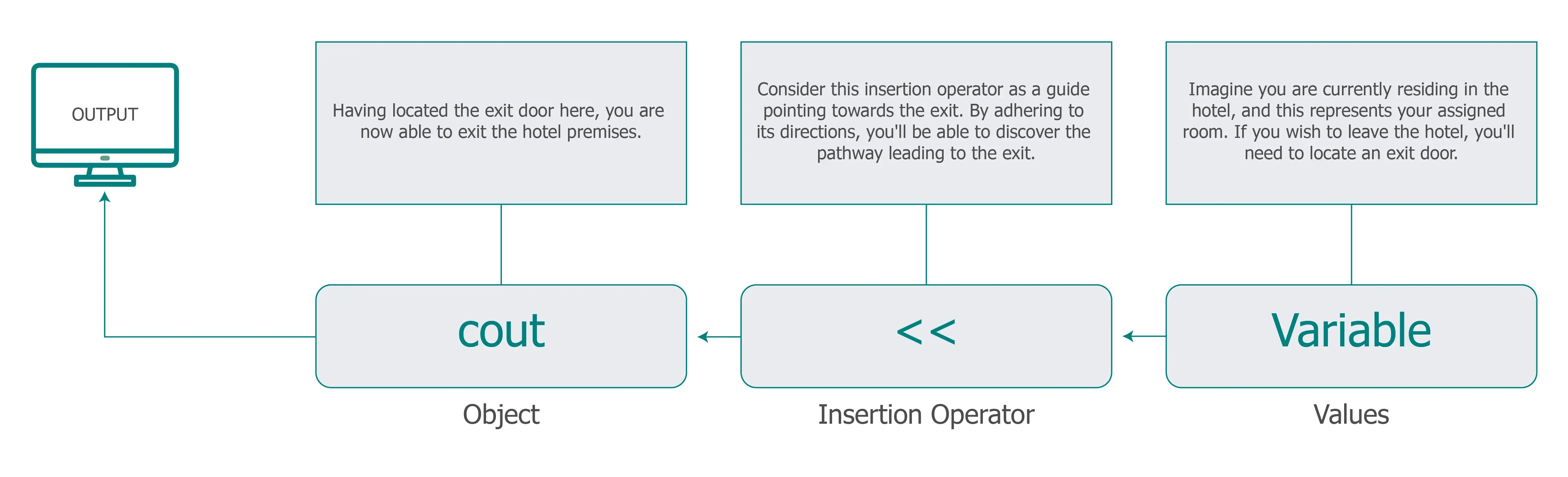
The predefined object cout is an instance of the ostream class, connected to the standard output device (typically the display screen). It is used with the stream insertion operator <<
, which inserts data into the preceding stream. For example:
cout<<"Welcome to InfoBrother";
The <<
operator incorporates the subsequent data into the stream that precedes it. In the example above, it integrated the string "Welcome to infoobrother" into the standard output stream, cout. It's noteworthy that the statement preceding the literal string is enclosed in double quotes "
since it represents a string.
Here's the thing about quotes: when you put text inside them, it appears exactly as you typed it. However, if there are no quotes, the computer assumes it's a special name for something you want to use. Check out the example below for a clearer picture.
#include using namespace std; main() { string name = "InfoBrother"; //Variable Declared cout << "welcome to: "; cout << name; return 0; }
In above example, the computer finds the variable called "name" and prints its content, which is "InfoBrother." So, instead of showing "Name," it prints "InfoBrother."
Multiple Insertion Operation (<<)
The insertion operator <<
can be used multiple times in a single statement, allowing for concise output statements. For Example:
cout << "welcome to: " << name;
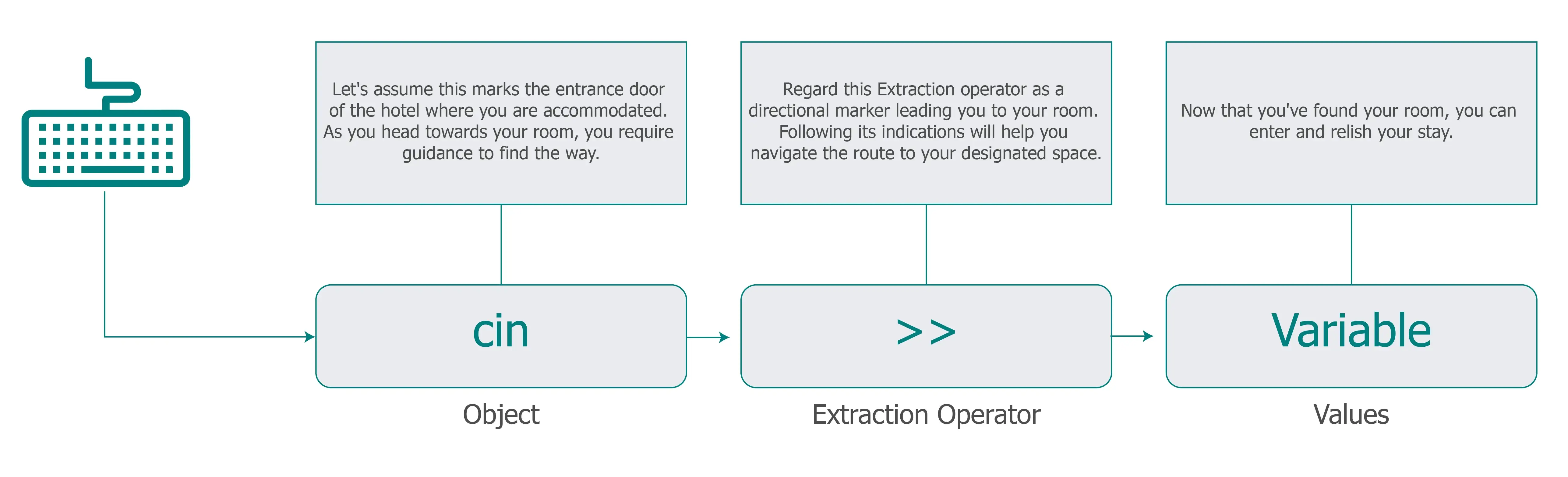
The predefined object cin is an instance of the istream class, connected to the standard input device (usually the keyboard). It is used with the stream extraction operator . For example:
int age; cout << "Enter your age: "; cin >> age;
In the example above, the first statement creates a variable of type int. The second line serves as a prompt, asking the user to input their age. Subsequently, the third line permits the user to enter their age, storing the input in the variable called "age" using cin
. This operation causes the program to pause, waiting for input from cin. Typically, this implies that the program is anticipating the user to type something using the keyboard.
Multiple Extraction Operation (>)
The extraction operator can be used multiple times in a single statement, simplifying input statements. For Example:
int a; int b; cin >> a >> b;
The predefined object cerr
is an instance of the ostream
class, connected to the standard error device (also a display screen). It is unbuffered and used with the stream insertion operator <<
. For example:
#include<iostream> using namespace std; main() { char str[] = "sorry for inconvenience..."; cerr << "Error : " << str << endl; return 0; }
The predefined object clog
is an instance of the ostream
class, connected to the standard error device (also a display screen). Unlike cerr, clog is buffered, holding its output until the buffer is full or flushed. It is used with the stream insertion operator <<
. For example:
#include<iostream> using namespace std; main() { // Declare variables int a; int b; int sum; // Prompt user for input cout << "Enter the first number: "; cin >> a; cout << "Enter the second number: "; cin >> b; // Calculate the sum sum = a + b; // Log the result using clog clog << "The sum of " << a << " and " << b << " is: " << sum << endl; return 0; }
Pro-Tip:
Although "clog" and "cerr" may not exhibit significant distinctions, it is advisable to employ "cerr" for error messages and "clog" for other log messages, particularly in more extensive programs.
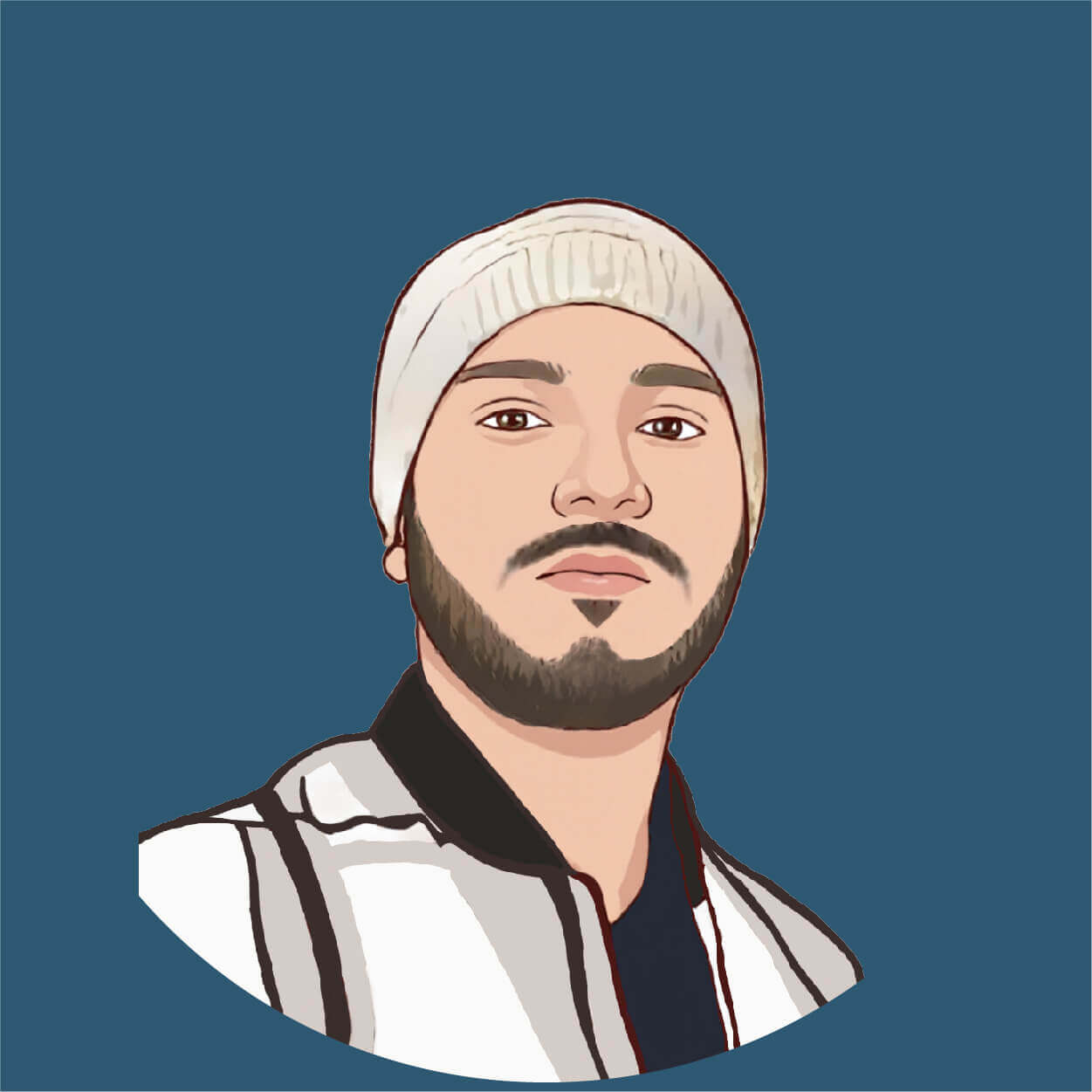
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.