PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
FOR LOOP:
The for
loop is a repetition control structure in C++ that efficiently executes a specific number of iterations. It encloses one or more statements forming the body of the loop, which repeats continuously for a specified number of times.
for (Initialize; condition; incrementation)
{
/* Body of the for Loop */
}
Within the parentheses ()
of the for statement, semicolons ;
separate three expressions: Initialize, condition, and incrementation:
This step is executed first and only once. It allows us to declare and initialize any loop control variables. We are not required to put a statement here, as long as a semicolon appears.
Next, the condition is evaluated. If it is true, the body of the loop is executed; if it's false, the body of the loop does not execute, and control jumps to the next statement after the for-loop (if any).
After the body of the for-loop executes, control jumps back up to the incrementation. This statement allows us to update any loop control variables. This statement can be left blank, as long as a semicolon appears after the condition.
The condition is then evaluated again. If it is true, the loop executes, and the process repeats itself (body of the loop, then increment step, and then again condition). After the condition becomes false, the for loop terminates.
Let's illustrate this concept with a simple example: printing the first 10 digits (1-10).
#include <iostream>
using namespace std;
int main()
{
int i; // Declare variable i of type int.
/* Initialize; Condition; Incrementation */
/* Note that these three statements must be separated by semicolons (;) */
for(i = 1; i <= 10; i++)
{
cout << i << endl;
}
/* endl is used to insert a newline at the end of each line: */
return 0;
}
In a for-loop, all three statements must be separated by semicolons (;).
We can initialize and declare a variable within the parentheses of the for-loop as shown below. Note that there will be no more variable (i.e., "i") outside the body of the for-loop, as it is declared only for the for-loop.
#include <iostream>
using namespace std;
int main()
{
/* Initialize; Condition; Incrementation. */
for(int i = 1; i <= 10; i++)
{
cout << i << endl;
}
return 0;
}
Let's consider another useful example demonstrating the uses of a for-loop: creating math multiplication tables. This program can generate a table for any number up to any given maximum limit. It prompts the user for a number to create the table for and the maximum limit for the table, starting from 1 and ending at the specified maximum limit.
#include<iostream>
using namespace std;
int main()
{
int Number, counter, MaxLimit;
cout << "For what number do you need to make a table? ";
cin >> Number;
cout << "Enter the maximum limit for the table: ";
cin >> MaxLimit;
/* Loop will start from 1 and end at MaxLimit */
/* initialization; condition; incrementation */
for(counter = 1; counter <= MaxLimit; counter = counter + 1)
{
cout << Number << " X " << counter << " = " << Number * counter << "\n";
/* Number multiplied by counter equals the result */
}
return 0;
}
The for-loop is most frequently used as a count-controlled loop, or one whose execution is controlled by counting the number of repetitions. Although the initialize portion of a for loop can contain multiple statements, some programmers believe the best style is to use only the loop control variable in the for statement and to insert other statements before the loop begins.
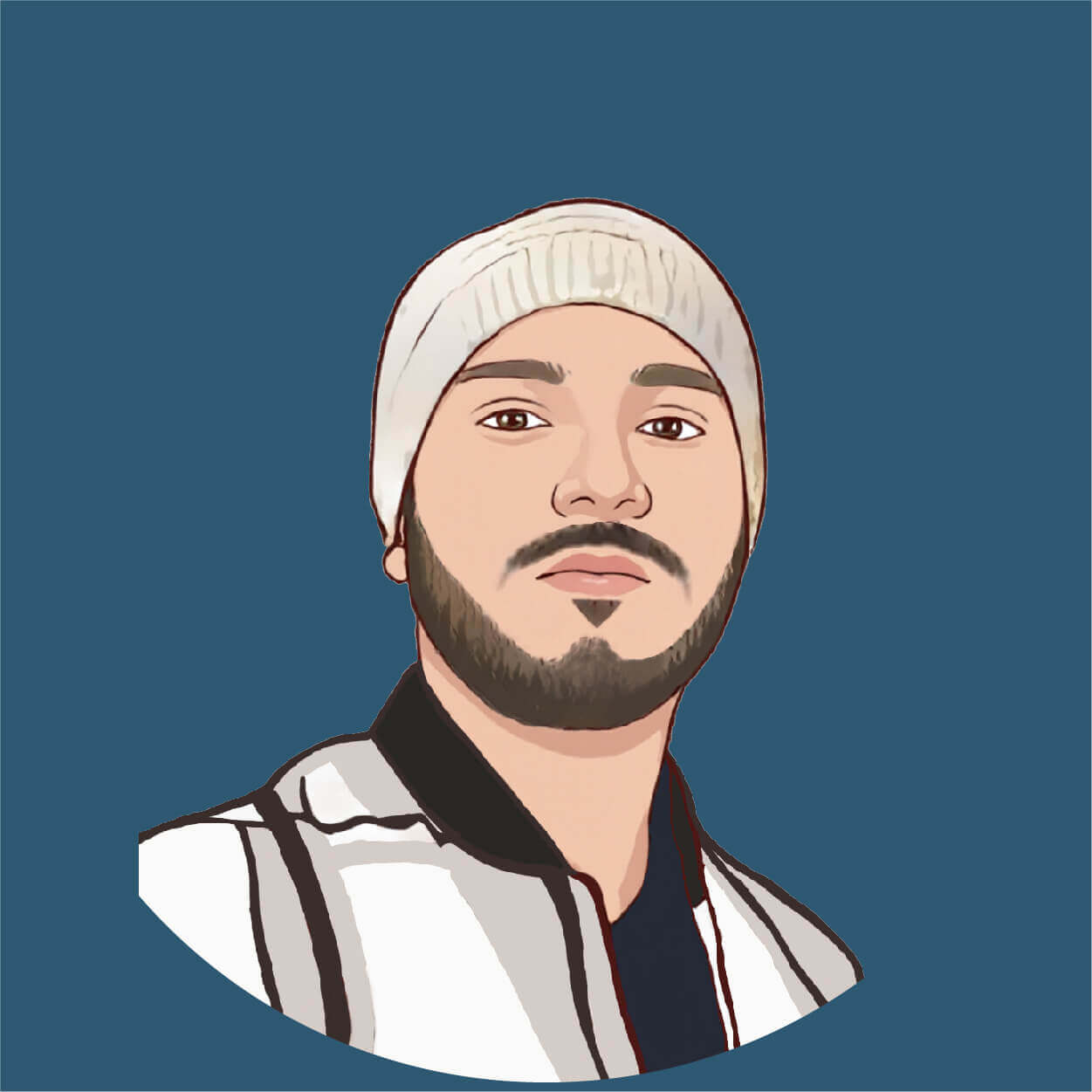
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.