PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
POINTERS:
Pointers represent a specialized type of variable capable of storing the memory address of another variable. They hold memory addresses rather than the values of variables. In C++, pointers play a crucial role in programming, facilitating certain tasks more efficiently. Understanding pointers is key, and we can illustrate their concept through the following example.
Imagine we ask someone to deliver a parcel to Omar's house. Initially, our reference point is Omar's name. However, if we provide the person with the house number and street address, we're referencing by the address.
When we declare a variable in C++, it reserves space in the computer's memory, each location possessing a unique address accessible using the ampersand &
operator. Let's consider the following example, which demonstrates printing the address of defined variables.
#include <iostream>
using namespace std;
int main() {
int a, b;
double c;
float d;
cout << "The Address of a is: " << &a << endl;
cout << "The Address of b is: " << &b << endl;
cout << "The address of c is: " << &c << endl;
cout << "The address of d is: " << &d << endl;
return 0;
}
In C++, declaring a pointer involves prefixing its name with an asterisk *
. Additionally, we specify the pointer's type, indicating the type of data stored at the address it will hold. For instance:
int* ptr;
int *ptr;
int*ptr;
int * ptr;
Pro-Tip:
The spacing around the asterisk is insignificant; each statement above declares an integer pointer.
In these declarations, ptr
denotes our variable's name, while the asterisk *
signals the compiler to allocate memory for storing an address. int
signifies our intent to use the pointer to store the address of an integer variable, making it point to an integer.
After declaring a pointer variable, such as ptr
, and a variable, let's say "x," we often need to initialize the pointer. To accomplish this, we use the address operator &
to assign the address of "x" to ptr
, like so:.
ptr = &x;
The address operator &
retrieves the memory address of "x" and assigns it to the pointer ptr
, effectively making ptr
point to "x."
Experienced C++ programmers leverage pointers for various purposes, including substituting array names. Since both array names and pointers hold memory addresses, a pointer can effectively hold an array name as long as their types match.
Consider the following example, where we declare two variables, assign values, and attempt to modify their values using pointers:
#include <iostream>
using namespace std;
int main() {
int x = 5, y = 15;
int *ptr;
cout << "Before: x = " << x << " | y = " << y << endl;
ptr = &x;
*ptr = 10;
ptr = &y;
*ptr = 20;
cout << "After: x = " << x << " | y = " << y << endl;
return 0;
}
In C++, "NULL" serves as a constant value indicating null pointers, typically defined in various C++ libraries, including <iostream>. Should it not be defined, we can define it as zero ourselves.
Before a pointer receives an assignment, it contains an arbitrary value. Attempting to use such an uninitialized pointer could lead to program crashes. To mitigate this risk, C++ programmers conventionally assign the null value to unused pointers, ensuring they don't inadvertently point to anything. Initializing pointers to null helps prevent unintended use.
To check for a null pointer, we utilize if-statements:
if (ptr == NULL);
if (!ptr);
if (ptr != NULL);
if (ptr);
Assigning NULL to a pointer variable during declaration is a good practice when the exact address is unknown, as demonstrated below:
#include <iostream>
using namespace std;
int main() {
int *ptr = NULL;
cout << "The value of Ptr is : " << ptr << endl;
return 0;
}
Pointers are indispensable for C/C++ programmers, not only for their flexibility but also for the speed they add to programs. Understanding pointers is fundamental, as they enable tasks that would be challenging or impossible otherwise, such as dynamic memory allocation and advanced functions.
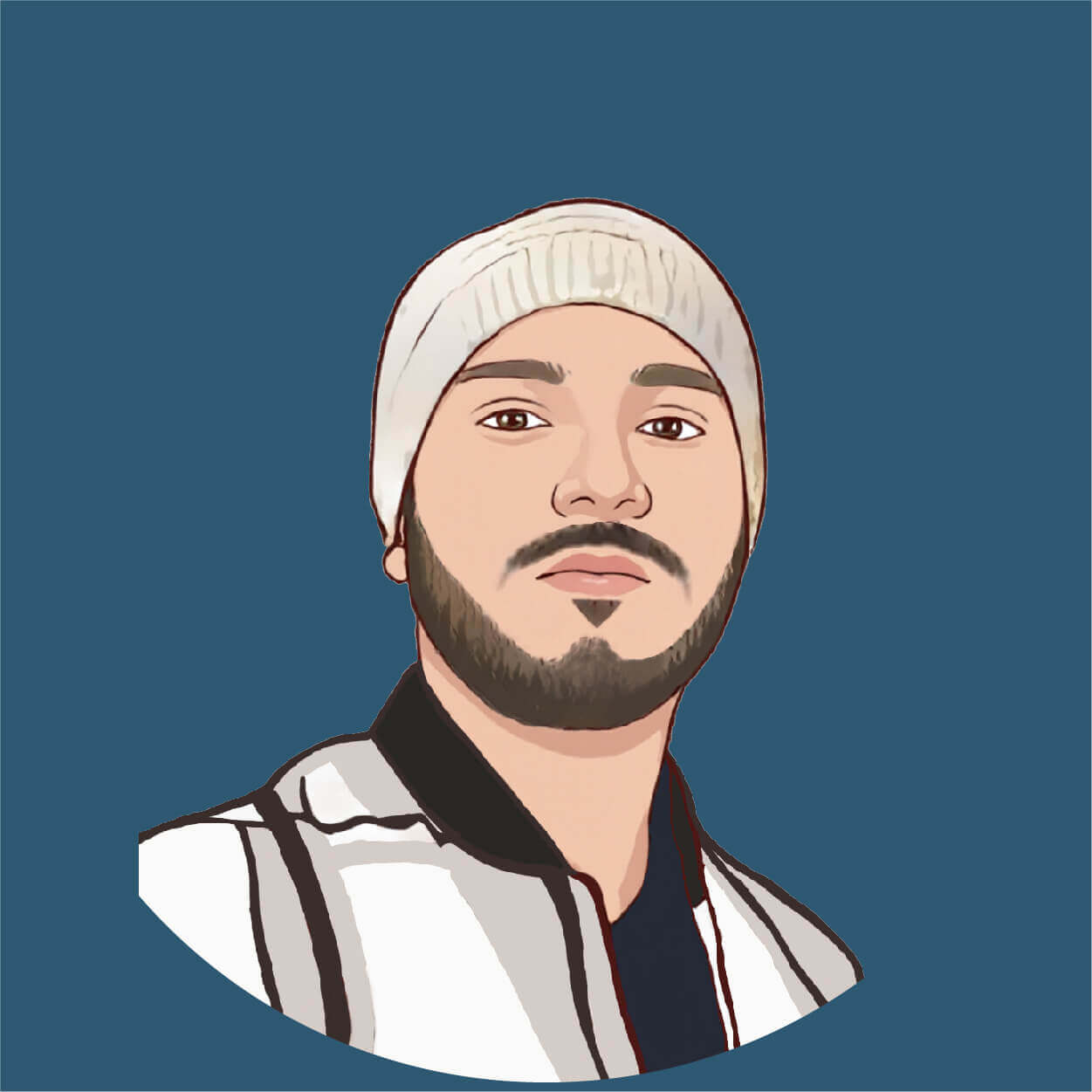
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.