PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Pointer to Function:
Utilizing pointer variables extends to passing arguments to functions. Pointers enable the transmission of a local variable's address into a function, allowing for modification of said variable within the function's scope. Understanding the significance of pointers in functions necessitates grasping how arguments are conveyed, which can be done in two ways:
The default parameter passing mechanism in C++ is known as Pass by Value or call by value. This approach involves copying the value of the actual parameter into the formal parameter for executing the function's code. As the function operates on a copy of the actual parameter, it cannot directly modify the value of the actual parameter possessed by the caller.
Let's illustrate this concept with a straightforward example where arguments are passed by value to a function. In this example, we'll create a function aiming to modify the value of the actual variable.
#include <iostream>
using namespace std;
// Function to change the value of the actual variable:
void increment(int x) {
++x; // Increment the value
}
int main() {
int x = 10; // Actual variable
cout << "Before increment: " << x << endl;
increment(x); // Function call (call by value)
cout << "After increment: " << x << endl;
return 0;
}
Passing by Pointer, also referred to as call by Pointer, involves copying the address of an argument into the formal parameter. Within the function, this address is used to access the actual argument used in the call. Consequently, modifications made to the parameter affect the passed argument. To employ passing by pointer, argument pointers are passed to functions akin to any other value. Thus, function parameters need to be declared as pointer types, facilitating the exchange of values between two integer variables pointed to by its arguments.
Let's revisit the previous example of "passing by value," but this time, we'll pass the argument by pointer.
#include <iostream>
using namespace std;
// Function to change the value of the actual variable:
void increment(int *x) {
++*x; // Increment the value
}
int main() {
int x = 10; // Actual variable
cout << "Before increment: " << x << endl;
increment(&x); // Function call (call by pointer: pass address)
cout << "After increment: " << x << endl;
return 0;
}
Let's examine a simple example to comprehend the value of pointers in functions. In this scenario, we'll create two swap functions attempting to interchange the values stored in two variables. The first function will take two simple arguments, and in the main function, we'll attempt to call the function by values. Conversely, the second function will accept two pointers as arguments, and in the main function, we'll call the function by reference.
#include <iostream>
using namespace std;
// Swap function without pointers:
void swap1(int left, int right) {
int temp;
temp = left;
left = right;
right = temp;
}
// Swap function using pointers:
void swap2(int *p_left, int *p_right) {
int temp = *p_left;
*p_left = *p_right;
*p_right = temp;
}
int main() {
int x = 10, y = 20; // Two values
swap1(x, y); // Calling function by value
cout << "Swap Without Pointer: x = " << x << " | y = " << y << '\n';
swap2(&x, &y); // Calling function by reference
cout << "Swap with pointer: x = " << x << " | y = " << y << '\n';
return 0;
}
Pro-Tip:
If you are seeking a job as an accountant, you do not need to highlight your web design skills.
This function merely exchanges the values of two variables local to the swap function. It can't modify the values passed into it since they merely hold copies of the original values (the values stored in variables x and y) passed into them. Visually, the function call copies the values of x and y into the variables left and right.
Function Swap2():More intriguingly, this function accepts the addresses of the local variables x and y. The variables p_left and p_right now point to x and y. Consequently, the swap2() function gains access to the memory backing those two variables. Therefore, when it performs the swap, it writes into the memory of variables x and y.
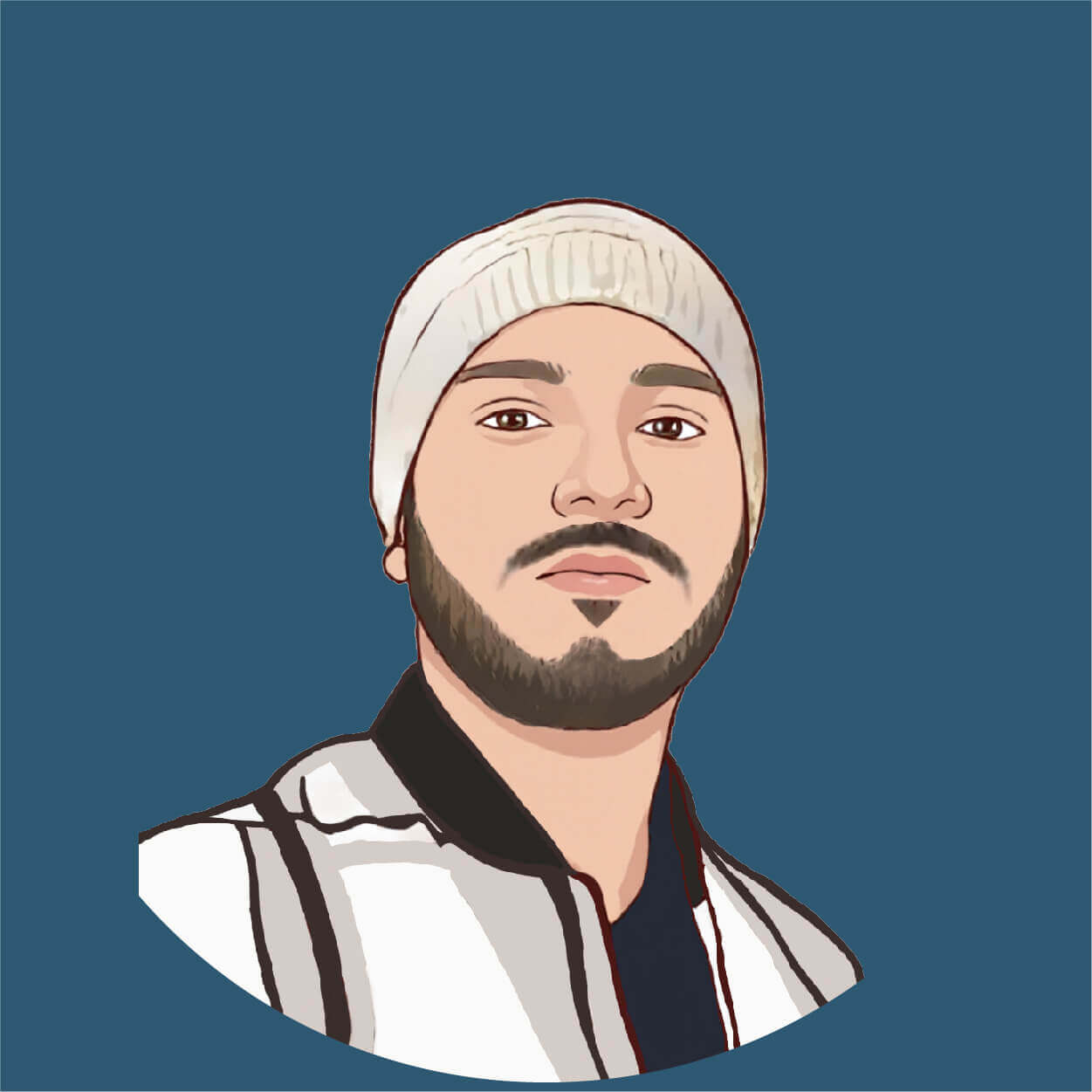
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.