PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
STREAM MANIPULATIONS:
Stream manipulators in C++ are operators utilized for formatting output. They empower programmers to control the presentation of data according to their requirements. Until now, the formatting of input and output information has been governed by the default settings of the C++ Input/Output system.
However, there are two ways to precisely control data formatting: using member functions of the ios class or employing special functions known as manipulators. Let's delve into formatting using the ios
member functions.
C++ provides built-in manipulator functions within various libraries. These libraries can be included in our programs to leverage these functions:
Provides fundamental functionalities for stream Input/Output in C++.
Contains utilities for performing formatted Input/Output with parameterized stream manipulation.
Deals with user-controlled file processing operations.
Facilitates in-memory formatting similar to file processing, but operations are performed on character arrays instead of files.
For programs that combine C and C++ styles of Input/Output.
These manipulators are applicable to both input and output streams, although some may only affect either output or input streams.
boolalphaAlphanumeric bool Values:- This sets the
boolalpha
format flag for the stream. When activated, bool values are represented as either "true" or "false" instead of integral values. - This flag can be disabled with the
noboolalpha
manipulator.
showbaseShow Numerical Base Prefixes:
- Activates the
showbase
format flag for the stream. It prefixes numerical integer values with appropriate base indicators like "0x" for hexadecimal values and "0" for octal values. - This can be turned off with the
noshowbase
manipulator.
showpointShow Decimal Point:
- Sets the
showpoint
format flag for the stream. It ensures that the decimal point is always written for floating-point values, even if the decimal part is zero. - The
noshowpoint
manipulator disables this behavior.
showposShow Positive Signs:
- Enables the
showpos
format flag, which adds a plus sign (+) before every non-negative numerical value. noshowpos
manipulator turns off this behavior.
skipwsSkip White Spaces:
- This flag, when set, causes leading whitespace characters to be ignored during input operations.
noskipws
manipulator disables this behavior.
unitbufFlush Buffer After Insertions:
- Activates the
unitbuf
flag, which flushes the buffer after each insertion operation. nounitbuf
manipulator disables this, preventing flushes after every insertion.
uppercaseGenerate Upper-Case Letters:
- When set, this flag instructs the stream to use uppercase letters instead of lowercase in representations like hexadecimal values.
nouppercase
manipulator reverses this behavior.
Let's explore each of these manipulators with examples:
#include <iostream>
#include <sstream>
#include <ios>
#include <fstream>
using namespace std;
int main() {
// boolalpha & noboolalpha
bool b = true;
cout << "boolalpha: " << boolalpha << b << endl;
cout << "noboolalpha: " << noboolalpha << b << endl;
// showbase & noshowbase
int x = 15;
cout << hex << "\nshowbase: " << showbase << x << endl;
cout << hex << "noshowbase: " << noshowbase << x << endl;
// showpoint & noshowpoint
double a = 30, s = 10000.0, pi = 3.1416;
cout.precision(5);
cout << "\nShowpoint: " << showpoint << a << '\t' << s << '\t' << pi << '\n';
cout << "noshowpoint: " << noshowpoint << a << '\t' << s << '\t' << pi << '\n';
// showpos & noshowpos
int p = 1, z = 0, c = -1;
cout << "\nshowpos: " << showpos << p << '\t' << z << '\t' << c << endl;
cout << "noshowpos: " << noshowpos << p << '\t' << z << '\t' << c << endl;
// skipws & noskipws
char wa, wb, wc;
istringstream iss(" 123");
iss >> skipws >> wa >> wb >> wc;
cout << "\nSkipws: " << wa << wb << wc << '\n';
iss.seekg(0);
iss >> noskipws >> wa >> wb >> wc;
cout << "noskipws: " << wa << wb << wc << '\n';
// unitbuf & nounitbuf
ofstream outfile("test.txt");
outfile << unitbuf << "Test " << "file" << '\n'; // flushed three times
outfile.close();
// uppercase & nouppercase
cout << showbase << hex;
cout << "\nuppercase: " << uppercase << 77 << '\n';
cout << "nouppercase: " << nouppercase << 77 << '\n';
return 0;
}
These flags determine how integer values are represented in the stream: in decimal, hexadecimal, or octal form.
- decUse Decimal Base.
- hexUse Hexadecimal Base.
- octUse Octal Base.
#include <iostream>
using namespace std;
int main() {
int n = 70;
cout << "dec: " << dec << n << '\n'; // dec
cout << "hex: " << hex << n << '\n'; // hex
cout << "oct: " << oct << n << '\n'; // oct
return 0;
}
These flags control how floating-point values are represented in the stream: in fixed or scientific notation.
- fixedUse Fixed Floating-Point Notation.
- scientificUse Scientific Floating-Point Notation.
#include <iostream>
using namespace std;
int main() {
double a = 3.1415926534, b = 2006.0, c = 1.0e-10;
cout.precision(5);
cout << "default:\n";
cout << a << '\n' << b << '\n' << c << '\n';
cout << "\nfixed:\n" << fixed;
cout << a << '\n' << b << '\n' << c << '\n';
cout << "\nscientific:\n" << scientific;
cout << a << '\n' << b << '\n' << c << '\n';
return 0;
}
- Extracts whitespace characters until a non-whitespace character is encountered.
#include <iostream>
#include <sstream>
using namespace std;
int main() {
char a[10], b[10];
istringstream iss("one \n \t two");
iss >> noskipws;
iss >> a >> ws >> b;
cout << a << ", " << b << '\n';
return 0;
}
- endlInsert Newline and Flush.
- endsInsert Null Character.
- flushFlush Stream Buffer.
#include <iostream>
#include <fstream>
using namespace std;
int main() {
// endl
int a = 100;
double b = 3.14;
cout << "endl: " << endl;
cout << a;
cout << endl; // Manipulator inserted alone
cout << b << endl << a * b; // Manipulator in concatenated insertion
endl(cout); // endl called as a regular function
// Flush test.txt 100 times
ofstream outfile("test.txt");
for (int n = 0; n < 100; n++)
outfile << n << flush;
outfile.close();
return 0;
}
- setiosflagsSet Format Flags.
- resetiosflagsReset Format Flags.
- setbaseSet Basefield Flag.
- setfillSet Fill Character.
- setprecisionSet Decimal Precision.
- setwSet Field Width.
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
// setiosflags
cout << "setiosflags: " << endl;
cout << hex;
cout << setiosflags(ios::showbase | ios::uppercase);
cout << 100 << endl;
// resetiosflags
cout << "\nresetiosflags: " << endl;
cout << hex << setiosflags(ios::showbase);
cout << 100 << endl;
cout << resetiosflags(ios::showbase) << 100 << endl;
// setbase
cout << "\nsetbase: " << endl;
cout << setbase(16);
cout << 110 << endl;
// setfill
cout << "\nsetfill: " << endl;
cout << setfill('x') << setw(10);
cout << 77 << endl;
// setprecision
cout << "\nsetprecision: " << endl;
double f = 3.14159;
cout << setprecision(9) << f << '\n';
cout << fixed;
cout << setprecision(9) << f << '\n';
// setw
cout << "\nsetw: " << endl;
cout << setw(10);
cout << 77 << endl;
return 0;
}
This tutorial covers multiple stream manipulations in C++, including basic flags, numerical base flags, floating-point flags, input and output manipulators, and parameterized manipulators. Try running each example yourself to understand how these manipulations operate in C++.
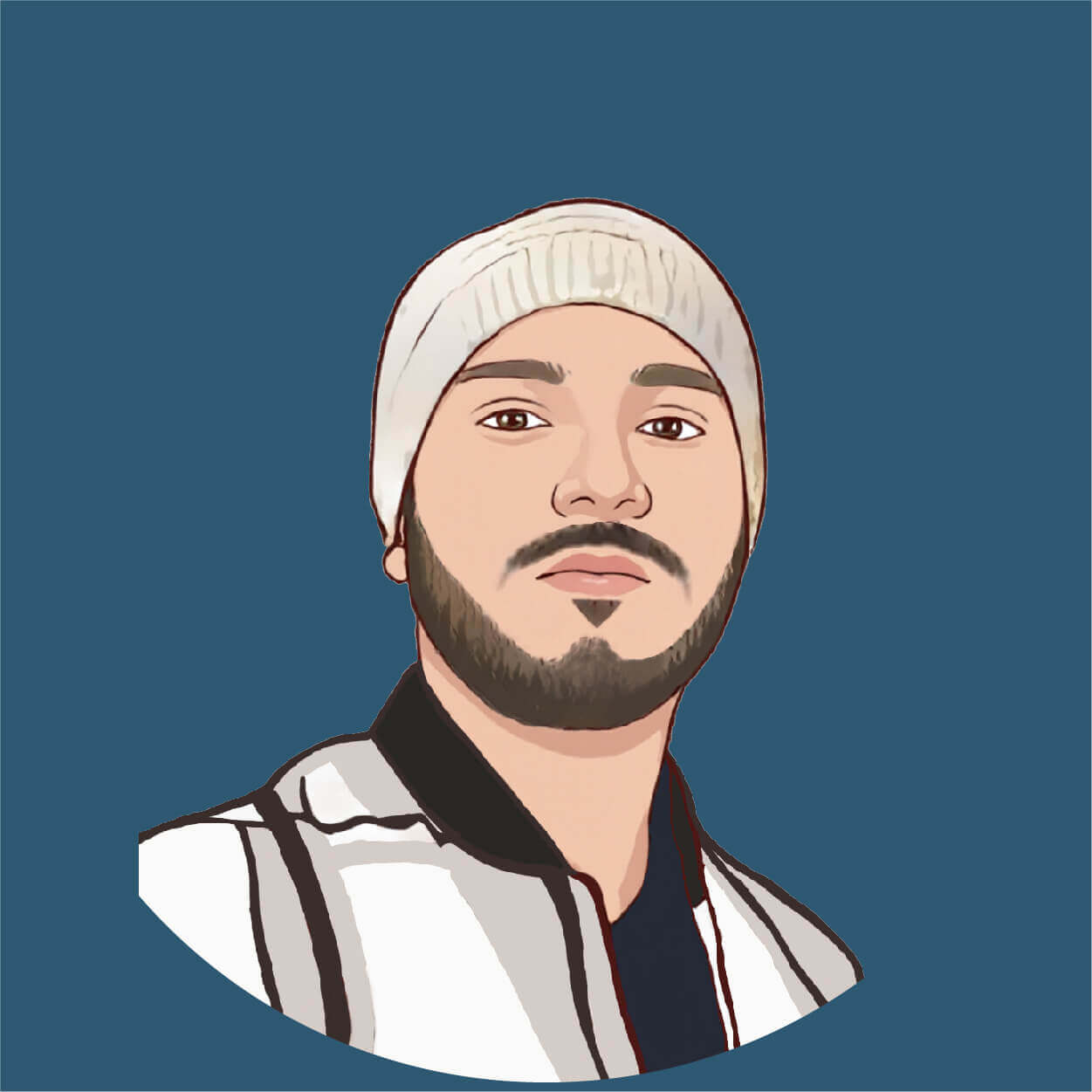
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.