PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Character Array Strings:
In C++, a character variable can hold a single character value, such as 'A' or '#'. Single character values are always expressed within single quotation marks ' '
. Whenever we declare a variable for a character with the type char
, it will hold enough memory for one character. If we want to express multiple character values as a constant, such as your name or any program title, we use double quotation marks " "
. A C++ value expressed within double quotation marks is commonly called a string, or a literal string.
In all programs seen until now, we have used only numerical variables to express numbers exclusively. However, in addition to numerical variables, there also exist strings of characters that allow us to represent successions of characters like words, sentences, names, texts, etc. Until now, we have only used them as constants, but we have never considered variables able to contain them.
In C++, there is no specific elemental variable type to store strings of characters. To fulfill this feature, we can use arrays of type char
, which are successions of "char" elements. Remember that this data type (char) is the one used to store a single character; for that reason, arrays of them are generally used to make strings of single characters. The basic syntax to use a character array is:
char Name[12];
In the above example, the array "Name" can store 12 characters long.
In C++, an array of characters and a string can be declared in the same way. We might create an array of characters if we need to store any name or grades, etc. We can store individual characters of a string in an array. However, we do not refer to an array of characters as a string unless the last usable character in the string is the "NULL character":
The Null Character is represented by the combination "\0" (backslash and zero), or by using the constant "NULL" that is available in the <iostream>library.
If we want to declare a string named Name and initialize it to "infobrother", each of the following statements provides the same result:
char Name[] = "InfoBrother";
char Name[] = {"InfoBrother"};
char Name[12] = "InfoBrother";
char Name[12] = {"InfoBrother"};
char Name[12] = {'I', 'n', 'f', 'o', 'B', 'r', '0', 't', 'h', 'e', 'r', '\0'};
Each statement reserves 12 character positions and stores an 'I' at Name[0], an 'n' at Name[1], an 'f' at Name[2], and so on.
Pro-Tip:
When we store a string, the curly braces are optional, but when we store individual characters, we must include them.
Actually, we do not place the Null character at the end of a string constant. The C++ compiler automatically places the \0
at the end of the string when it initializes the array. Let's have a simple example to print the above-mentioned string:
/* String: InfoBrother */
#include <iostream>
using namespace std;
int main ()
{
char Name[12] = {'i', 'n', 'f', 'b', 'r', 'o', 't', 'h', 'e', 'r'};
cout << Name;
return 0;
}
Since the value of an assignment can only be an element of an array and not the entire array, it would be valid to assign a string of characters to an array of Char using a method like this:
Name[0] = 'I';
Name[1] = 'n';
Name[2] = 'f';
Name[3] = 'o';
...
...
...
Name[10] = 'r';
But as we know, this does not seem to be a very practical method. Generally, for assigning values to an array, and more specifically to a string of characters, a series of functions like strcpy
(string copy) is defined in the <cstring>
(<string.h>) library and can be called the following way:
strcpy(String1, String2);
This copies the content of String2 into String1. String2 can be either an array, a pointer, or a constant string, so the following line of code would be a valid way to assign the constant string "InfoBrother" to Name as shown in the given example:
strcpy(Name, "InfoBrother");
Let's have a simple example by using strcpy()
function to clear this concept:
Note:
We need to include
/* Assigning Values to String Array: InfoBrother */
#include <iostream>
#include <string.h>
using namespace std;
int main ()
{
char name[12];
strcpy(name, "Infobrother"); // string copy function
cout << name;
return 0;
}
The standard C++ Library provides a string class that supports multiple string functions like the "strcpy" function. We discussed more about strings in our String tutorial.
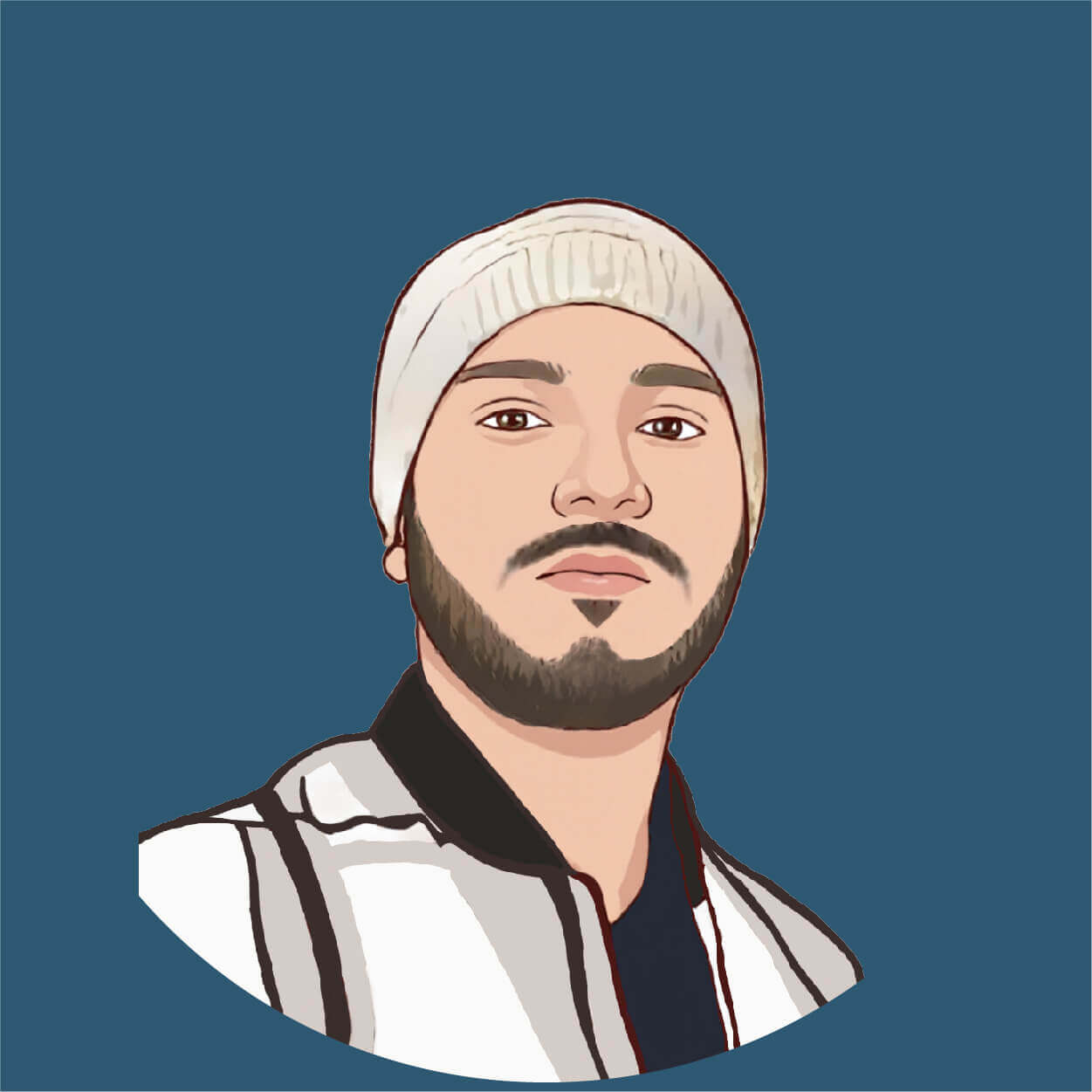
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.