PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
MULTIDIMENSIONAL ARRAYS:
Multidimensional arrays are used to represent data in multiple dimensions, such as a chess board or a tic-tac-toe board. Essentially, multidimensional data refers to having more than one index for the data. The simplest form of a multidimensional array is the "Two Dimensional Array."
A two-dimensional array is essentially a list of one-dimensional arrays. When we declare a one-dimensional array, we use a set of square brackets[ ]
after the array identifier. To declare a two-dimensional array, we need to use two sets of brackets after the array identifier. Similarly, for a three-dimensional array, three sets of brackets are required.
In C++, multidimensional arrays can be defined with any number of dimensions. The ANSI Standard specifies a minimum of 256 dimensions, but the actual limit depends on the available memory.
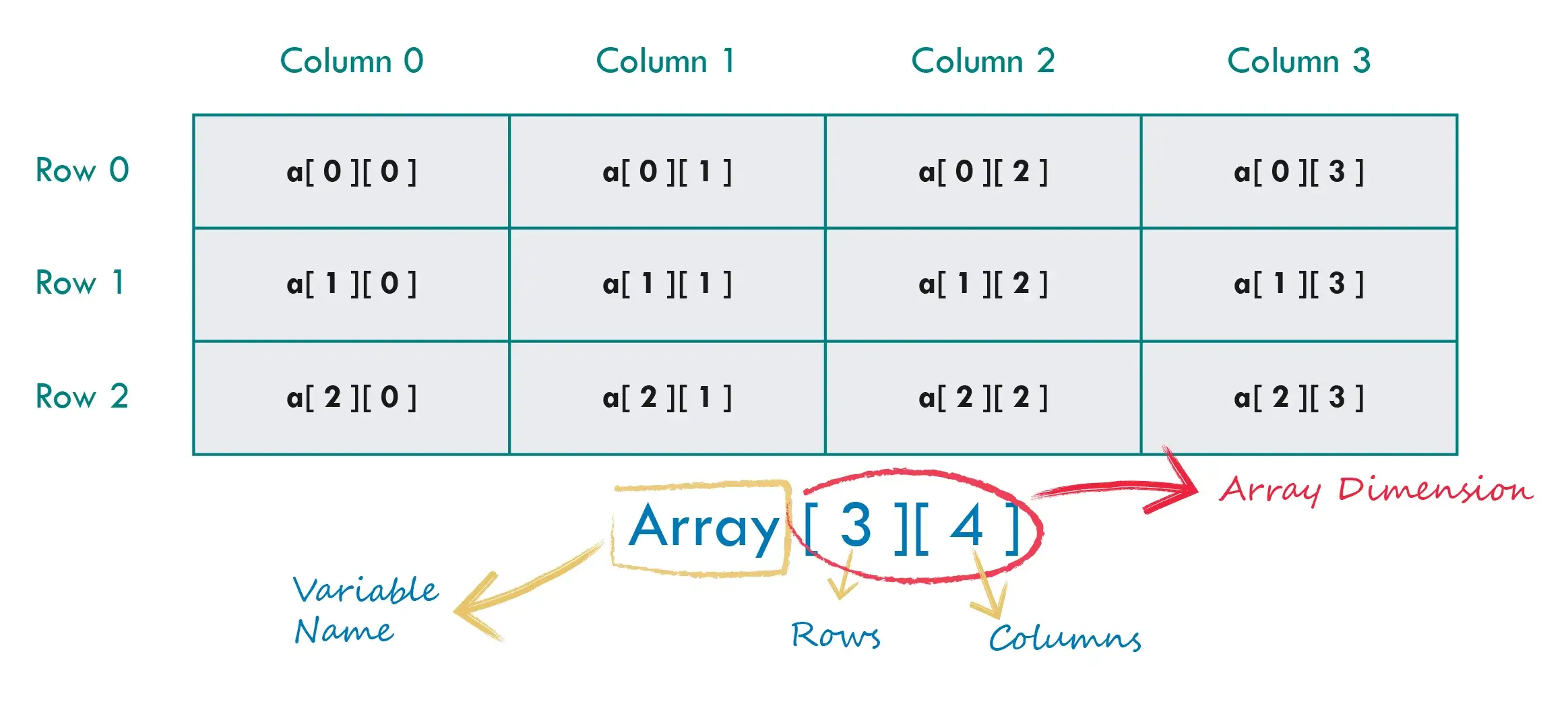
Since a two-dimensional array is rectangular, it requires two indices to access its elements: one for the row and one for the column. The first value indicates the row, and the second indicates the column.
a[row][column]
To declare a two-dimensional integer array of size x by y, we would write something like this:
Data_Type Array_Name[x][y];
The array size must be an integer constant greater than zero, and the type can be any valid C++ data type. For example, to declare a two-dimensional array for a "3 x 4" (three rows and four columns) chessboard with the variable name "Chess" and data type "int," we use the following statement:
int Chess[3][4];
In C++, there's no need for special syntax to define multidimensional arrays. An n-dimensional array is essentially an array with only one dimension whose elements are (n-1)-dimensional arrays. Multidimensional arrays can be initialized by specifying bracketed values for each row. For instance:
int Chess[3][4] = {
{0, 1, 2, 3},
{4, 5, 6, 7},
{8, 9, 10, 11}
};
The nested braces indicating rows are optional. The following initialization is equivalent to the previous example:
int Chess[3][4] = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11};
Once an array is filled with values, individual array elements can be accessed and used similar to accessing any single variable of the same type. Elements are accessed by indexing the array name using subscripts (row index and column index). For example:.
int Item = chess[2][3];
In the above statement, the assignment operator =
assigns the value of the fourth element from the third row of the array to the variable "Item."
Here's a comprehensive example demonstrating the declaration, initialization, and accessing of elements in a two-dimensional array:
#include <iostream>
using namespace std;
int main() {
// Declaration and initialization of a 3x4 chessboard
int chess[3][4] = {
{0, 1, 2, 3},
{4, 5, 6, 7},
{8, 9, 10, 11}
};
// Output each array element's value
for (int row = 0; row < 3; row++) {
for (int col = 0; col < 4; col++) {
cout << "chess[" << row << "][" << col << "]: ";
cout << chess[row][col] << endl;
}
}
// Accessing a specific element
int item = chess[2][3]; // Fourth element from the third row
cout << "\n\n The item stored in chess[2][3] is: " << item;
return 0;
}
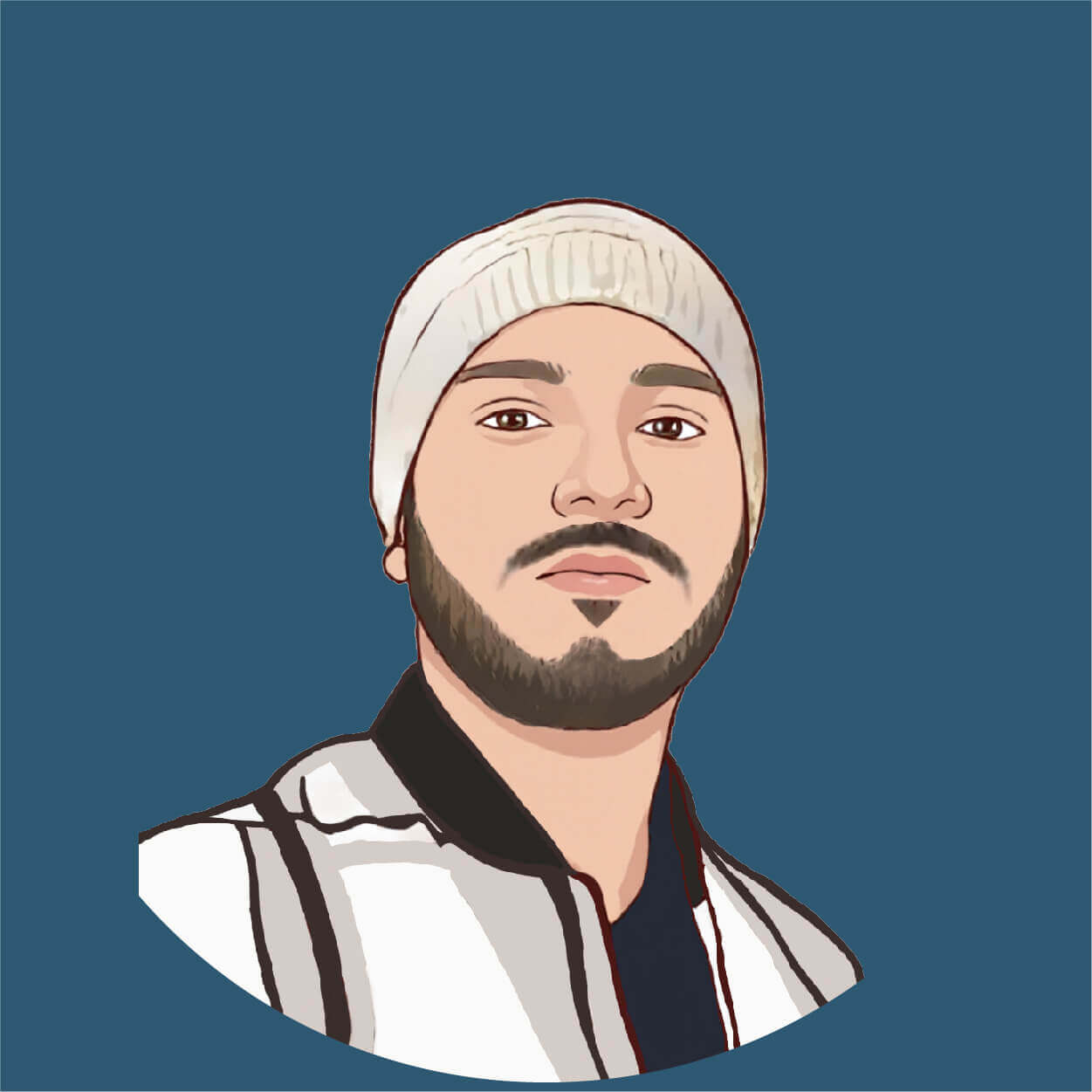
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.