PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
String:
A string, like "InfoBrother", is represented in C++ as a sequence of characters stored in memory. Essentially, a string is a character array and can be manipulated accordingly.
C++ offers two main representations for strings:
The C-style character string, originating from C, is still supported in C++. This string is essentially a one-dimensional array of characters terminated by a null character \0
Thus, a null-terminated string contains the characters of the string followed by a null.
Note:
The null character is represented either by \0
or by using the constant NULL
available in the "iostream" library.
If we declare a string named "Name" and initialize it to "infobrother", any of the following statements will yield the same result:
char Name[] = "Infobrother";
char Name[] = { "Infobrother" };
char Name[12] = "Infobrother";
char Name[12] = { "Infobrother" };
char Name[12] = { 'I', 'n', 'f', 'o', 'b', 'r', 'o', 't', 'h', 'e', 'r', '\0' };
Each statement reserves 12 character positions and stores 'I' at Name[0], 'n' at Name[1], 'f' at Name[2], and so on.
Curly braces are optional when storing a string, but they must be included when storing individual characters.
The null character \0
is automatically placed at the end of a string constant by the C++ compiler when it initializes the array. Let's consider a simple example to print the aforementioned string:
#include <iostream>
using namespace std;
main() {
char Name[12] = {'i', 'n', 'f', 'b', 'r', 'o', 't', 'h', 'e', 'r'};
cout << Name;
return 0;
}
The String class provided by the Standard C++ Library defines many functions to manipulate C++ strings. Here are some functions that manipulate null-terminated strings:
Function Name | Syntax | Purpose |
---|---|---|
String Copy | strcpy(S1, S2); | Copy string S2 to string S1. |
String Concatenate | strcat(S1, S2); | Concatenate string S2 at the end of string S1. |
String Length | strlen(S1); | Returns the length of string S1. |
String Compare | strcmp(S1, S2); | Compares S1 and S2. Returns 0 if both strings are equal, -1 if S1 < S2, and +1 if S1 >S2. |
String to Uppercase | strupr(S1); | Converts the entire string to uppercase. |
String to Lowercase | strlwr(S1); | Converts the entire string to lowercase. |
String Character | strchr(S1, 'b'); | Finds the first occurrence of the given character ('b') in a string and prints out the string starting from that character. |
String Reverse | strrev(S1); | Reverses the entire string. |
String Character | strchr(S1, ch); | Returns a pointer to the first occurrence of character ch in string S1. |
String String | strstr(S1, S2); | Returns a pointer to the first occurrence of string S2 in string S1. |
Let's consider a comprehensive example to understand all the string functions discussed above:
#include <iostream>
#include <string.h> // Required to use string functions.
using namespace std;
main() {
char S1[15] = "Welcome to "; // string S1
char S2[15] = "InfoBrother: "; // string S2
char S3[20];
// Copy string S1 to S3:
strcpy(S3, S1);
cout << "1): Strcpy(S3, S1): Value of S3 is: " << S3 << endl;
// Concatenate S1 and S2:
strcat(S1, S2);
cout << "\n2): strcat(S1, S2): After Concatenation of S1: " << S1 << endl;
// Total length of S1 after concatenation:
cout << "\n3): Strlen(S1): Total Length of String is: " << strlen(S1) << endl;
// Compare string S1 and S2:
int compare = strcmp(S1, S2);
cout << "\n4): Strcmp(S1, S2): After Compare, The Result is: " << compare << endl;
// Convert string S1 to uppercase:
strupr(S1);
cout << "\n5) Strupr(S1): S1 to Uppercase: " << S1 << endl;
// Convert string S1 back to lowercase:
strlwr(S1);
cout << "\n6) Strlwr(S1): S1 to Lowercase: " << S1 << endl;
// Find out the first occurrence of 'b' and print out the string after 'b':
cout << "\n7): String After first occurrence of 'b': " << strchr(S1, 'b') << endl;
// Reverse string S1.
strrev(S1);
cout << "\n8): Reverse String S1: " << S1;
return 0;
}
While we use cin
to get strings with the extraction operator as we do with fundamental data type variables, cin stops reading as soon as it encounters any blank space character. Thus, using cin, we cannot obtain an entire line, such as a complete user name. If a user enters both their first and last names, cin only gets the first name and stops reading at the blank space character. This behavior may not be desirable. To obtain entire lines, including spaces, we can use the
getline()
function, which is a more recommended way to get user input alongside cin.
The getline()
function requires two arguments: the first being cin, and the other being a variable to store the value. The syntax for the getline() function is as follows:
getline(cin, Variable-Name);
Let's consider an example to understand the concept of the getline() function in C++:
#include <iostream>
#include <string.h> // Required to use string functions.
using namespace std;
main() {
string name; // Name having type string.
cout << "Enter Your Full Name: ";
getline(cin, name); // Getline function.
cout << "Nice To Meet you: " << name;
return 0;
}
While the String class simplifies string handling compared to using character arrays, a disadvantage of using the String Class is that errors occur when assigning a long value to a string that previously held a shorter value. Thus, it is a reasonable option to choose never to bother with character arrays for storing string values and always use the String Class instead.
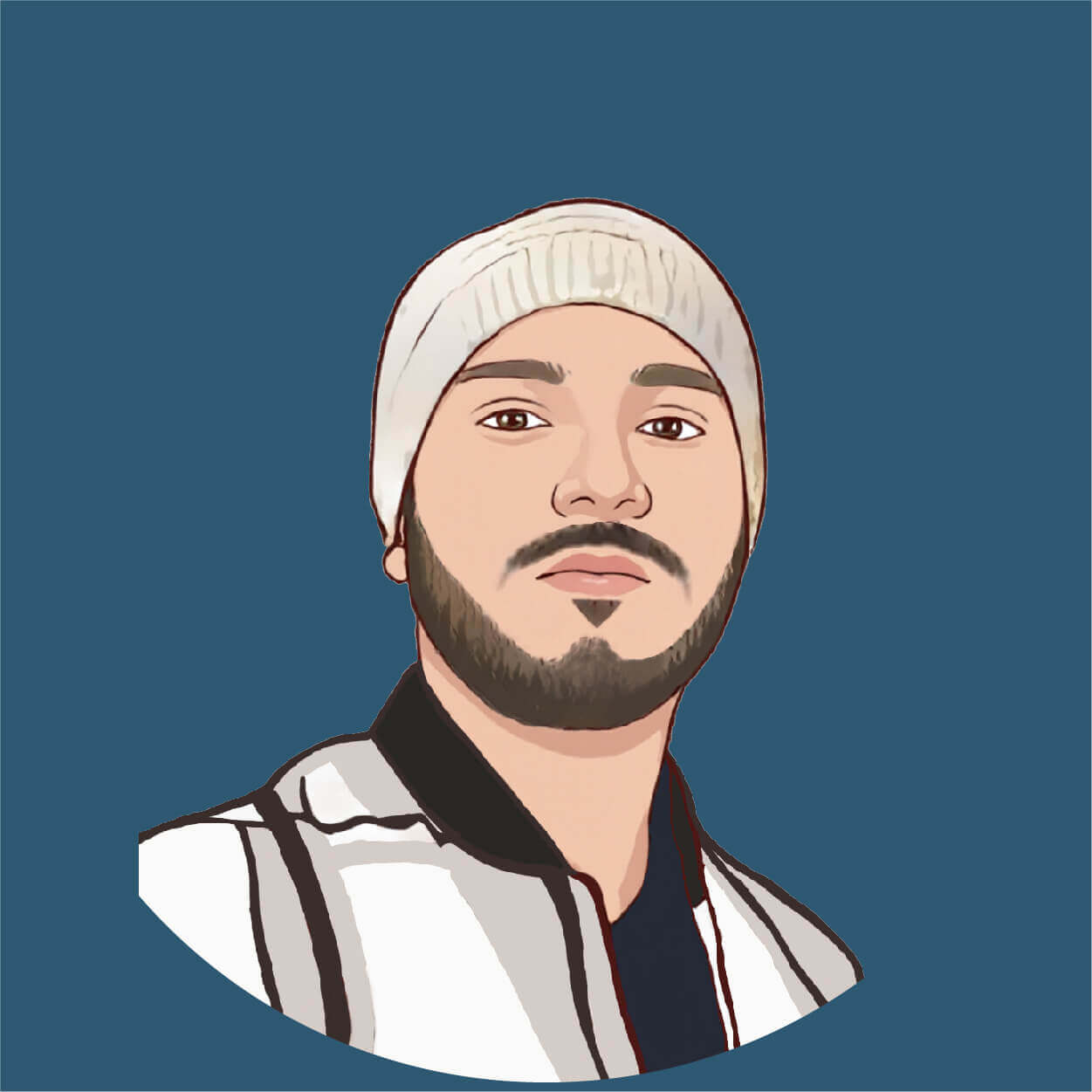
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.