PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Comments in C++
In C++, comments serve as annotations within the code that the compiler disregards. They provide a means for developers to include notes in the relevant sections of the source code. Two main types of comments exist:
- Single line Comments
- Multi-line Comments
Single line comments are initiated with the double slash //
symbol, signaling the compiler to disregard everything from that point until the end of the line. Typically, these comments are employed to provide brief annotations about a single line of code.
#include<iostream> using namespace std; main() { //This is a single line comment and will be ignored by the compiler. }
Multi-line comments begin with , indicating to the compiler to overlook everything between these symbols. Programmers often utilize multi-line comments not only to annotate code but also to enhance the visual appeal of their programs.
#include<iostream> using namespace std; main() { /* This is a multi-line comment and will be ignored by the compiler. */ }
It is recommended to use comments for libraries, functions, or classes to describe their purpose and functionality. A well-crafted comment provides a logical overview of the program's objectives without delving into the code details. This practice becomes particularly crucial when collaborating within a team, where not all team members may be familiar with the entire codebase.
At the statement level, comments should explain why the code is performing a specific action rather than detailing what the code is doing. If a statement requires a comment to elucidate its purpose, consider refactoring the statement instead.
// This is a for loop. for (int i = 0; i < 10; ++i) { // code... }
The comment placed in front of the for loop, stating "this is a for loop," is redundant and unnecessary. Since it is evident from the code structure that a for loop is being used, such comments are considered poor programming practice and do not contribute meaningfully to the code's clarity.
// This loop will repeat the statement 10 times. for (int i = 0; i < 10; ++i) { // code... }
However, in this context, the comment holds value as it explains the purpose of the loop. Therefore, incorporating comments that provide insights into the functionality is considered good programming practice in this scenario.
Commenting is an art that involves describing the program's functionality. Adopting this good programming practice ensures that anyone reviewing the code, including yourself in the future, can easily comprehend its purpose. In the long run, well-documented code with thoughtful comments becomes invaluable when reusing or maintaining code. Failure to include comments may lead to difficulties in understanding and reusing the code. Hence, incorporating comments is an essential aspect of creating clean and comprehensible code.
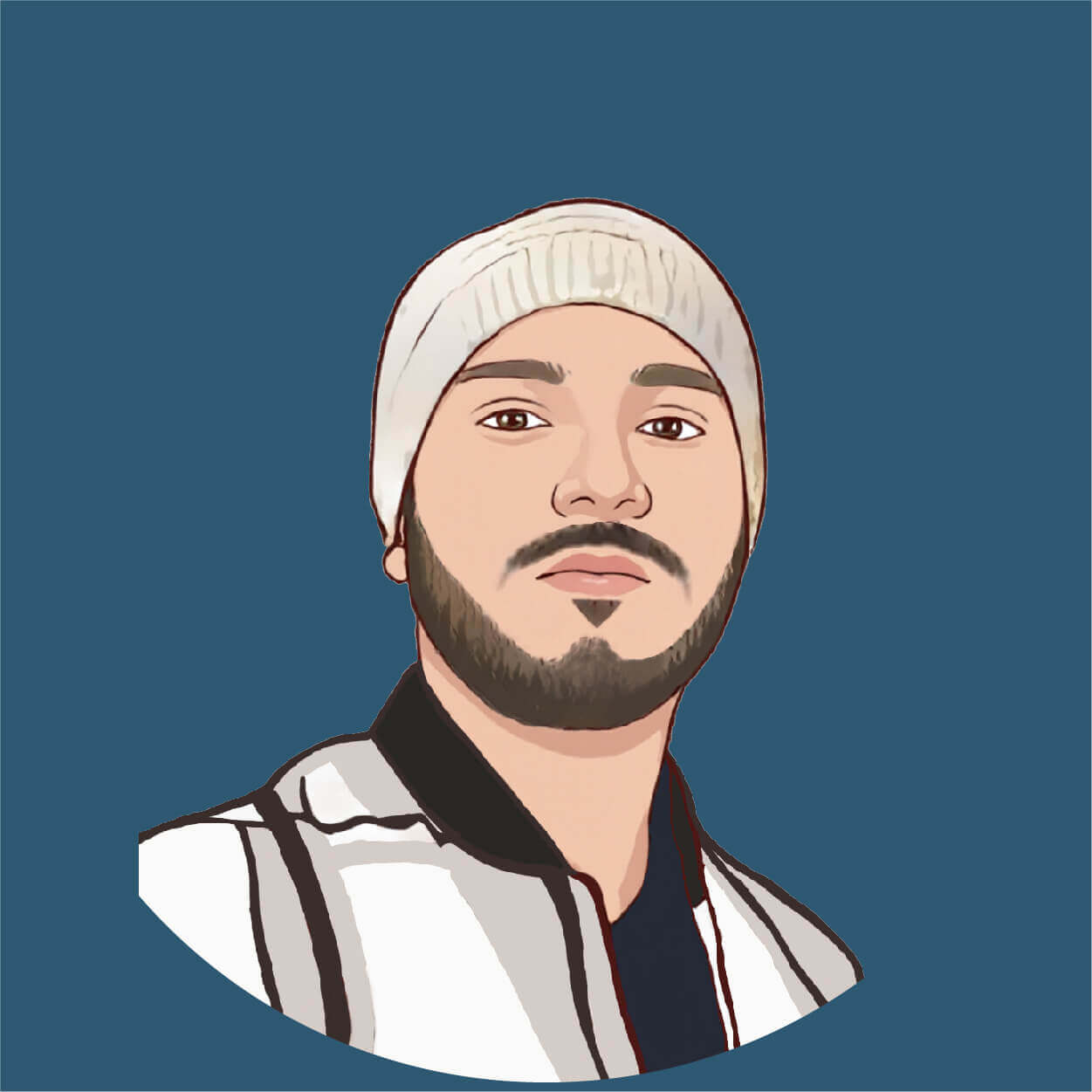
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.