PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
WHAT IS A VARIABLE?
In programming, think of a variable as a special space in your computer's memory. It's like a labeled cubbyhole where you can put in and take out values whenever you need.
Imagine the computer's memory as a series of numbered cubbyholes, each representing a memory address. Variables, then, are like labeled containers that make it easier to access information without having to remember specific memory addresses.
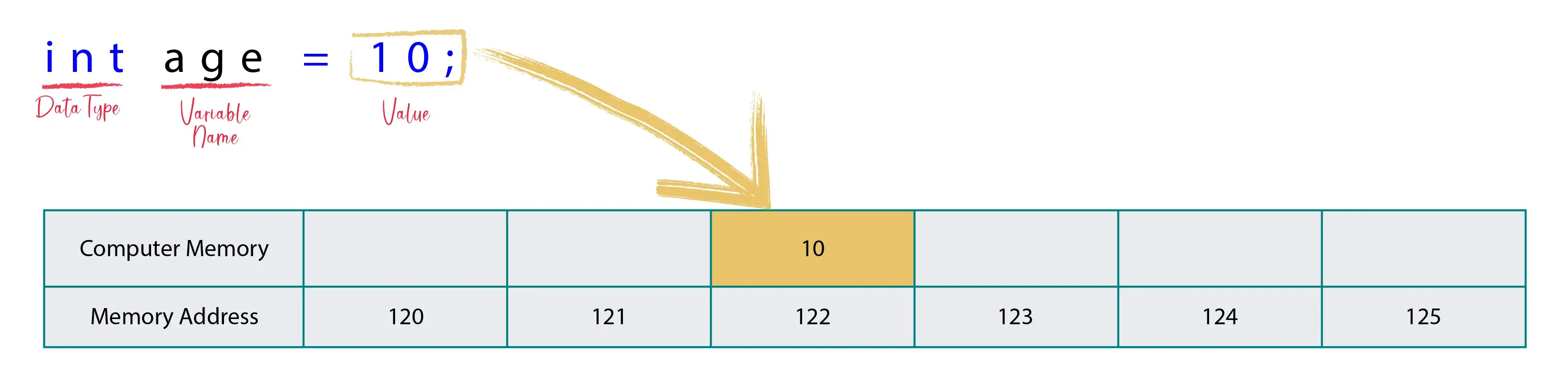
Variables come with names that describe their purpose. In C++, a variable name must start with:
- A letter (A to Z or a to z).
- An underscore (_) followed by letters.
- Digits (0 to 9).
- Punctuation characters such as @, $, and % are not allowed.
Moreover, C++ is case-sensitive, meaning "InfoBrother" and "infobrother" are treated as distinct variables.
While mathematicians might be content with single-letter variable names like x, programmers are encouraged to use more descriptive names. This enhances code readability for humans. For instance, using variable names like altitude, sum, and user_name is preferable to generic alternatives like a, s, and u.
C++ imposes strict rules for variable names, and it is essential to be aware of these before creating any variable in C++ programming:
- Identifiers must contain at least one character.
- The first character must be an alphabetic letter (upper or lower case) or an underscore.
- The remaining characters can be alphabetic (upper or lower case), underscore, or a digit.
- No other characters, including spaces, are allowed.
- Reserved words cannot be used as identifiers.
Here are examples of acceptable identifiers:
int studentAge; int _gotoValueX; int V0024; float averageScore;
Variables defined inside functions are known as local variables. They exist in memory only when within scope, freeing up memory when the program execution leaves that scope. Local variables can share names across different functions without conflict since the compiler associates each variable with its function declaration.
A local variable has an undefined value after declaration until properly initialized. It is essential not to use its value until that point.
Conversely, a variable defined outside any function is a global variable. It is not tied to a specific function and can be accessed or modified by any function declared after it in the source code. Global variables do not need explicit initialization; numeric types are automatically set to zero.
Before using a variable, it needs to be declared to inform the compiler. The syntax for declaring a variable is:
int age; // Declaration of an integer variable named 'age'
Values can be assigned to variables either at the time of declaration or later in the program. Multiple variables of the same type can be declared in a single line using the comma ,
operator.
int x, y, z;
Variables in a C++ program can be defined either within functions or outside them, leading to two types of scope:
- A variable defined within a function is localand is limited to that specific function.
- A variable defined outside of each function is globaland can be used by all functions.
Initialization involves assigning a value to a variable during its declaration. This can be achieved by using an equals sign =
followed by the initial value.
int age; // Integer variable named 'age' age = 10; //Assigning the value 10 to the variable 'age'. int number = 7; //Initializing values during declaration.
Variables can also be initialized using round brackets containing the initial value.
float pi(3.14); // Assigning the value 3.14 to the variable 'pi'.
//Example Program Demonstrating Local and Global Variables: #include <iostream> using namespace std; const double pi = 3.141593; //Global variable. main() { double area, circuit, radius = 1.5; //local variable. area = pi * radius * radius; circuit = 2 * pi * radius; cout << "To Evaluate a Circle\n" << endl; cout << "Radius: " << radius << endl << "Circumference: " << circuit << endl << "Area: " << area << endl; return 0; }
Note:
Local variables must be initialized manually, while global variables are automatically initialized to zero.
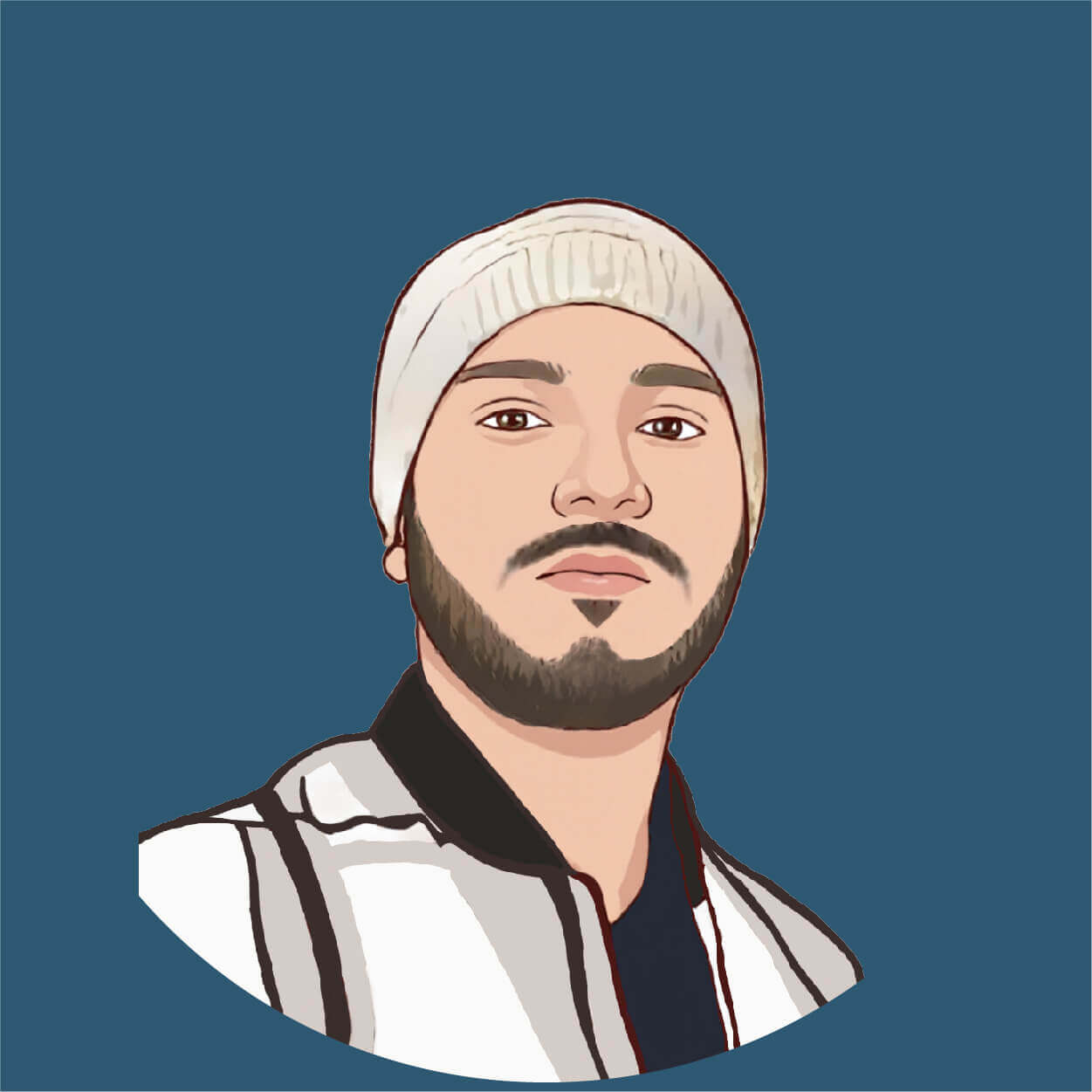
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.