PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Constructor Overloading:
In our previous tutorial on Operator Overloading, we discussed the concept of overloading and specifically focused on Operator Overloading. In this tutorial, we will delve into Constructor Overloading in C++ Programming.
A constructor is a special method of a class that is automatically invoked whenever an object of the class is created. It's responsible for initializing the object and allocating memory for its class. If a class is created without a constructor, the compiler will automatically generate a default constructor for it.
Constructor Overloading is a technique used to create multiple constructors with different arguments. It allows us to use a single class in various ways, enabling the class to behave differently based on the constructor being overloaded. Let's explore an example to understand how constructor overloading works and why we need it.
For deeper understanding, read constructors in C++ programming.
#include<iostream>
using namespace std;
class Overloading {
private:
string name;
string Day;
int temp;
public:
// Default constructor:
Overloading() {
name = "Abbottabad";
temp = 29;
cout << name << " Yesterday's Temperature: " << temp << "°" << endl;
}
// Constructor Overloading using parameters.
Overloading(string nam, int tem) {
name = nam;
temp = tem;
cout << name << " Today's Temperature: " << temp << "°" << endl;
}
// Constructor Overloading with more parameters.
Overloading(string nam, string day, int tem) {
name = nam;
temp = tem;
Day = day;
cout << name << " " << Day << " Temperature: " << temp << "°" << endl;
}
};
int main() {
// Object Creation:
// 1 - Default constructor called
Overloading obj1;
// 2 - Overloaded constructor with parameters
Overloading obj2("Abbottabad", 28);
// 3 - Overloaded constructor with different parameters
Overloading obj("Abbottabad", "Monday", 26);
return 0;
}
In the example above, we have created three constructors:
- The first one is the default constructor with no parameters.
- The second one is an overloaded constructor with some parameters.
- And lastly, we overload the constructor again with a different number of parameters.
Creating an overloaded constructor is straightforward, similar to overloading functions. We can overload constructors as many times as necessary, but each constructor must have a different argument list.
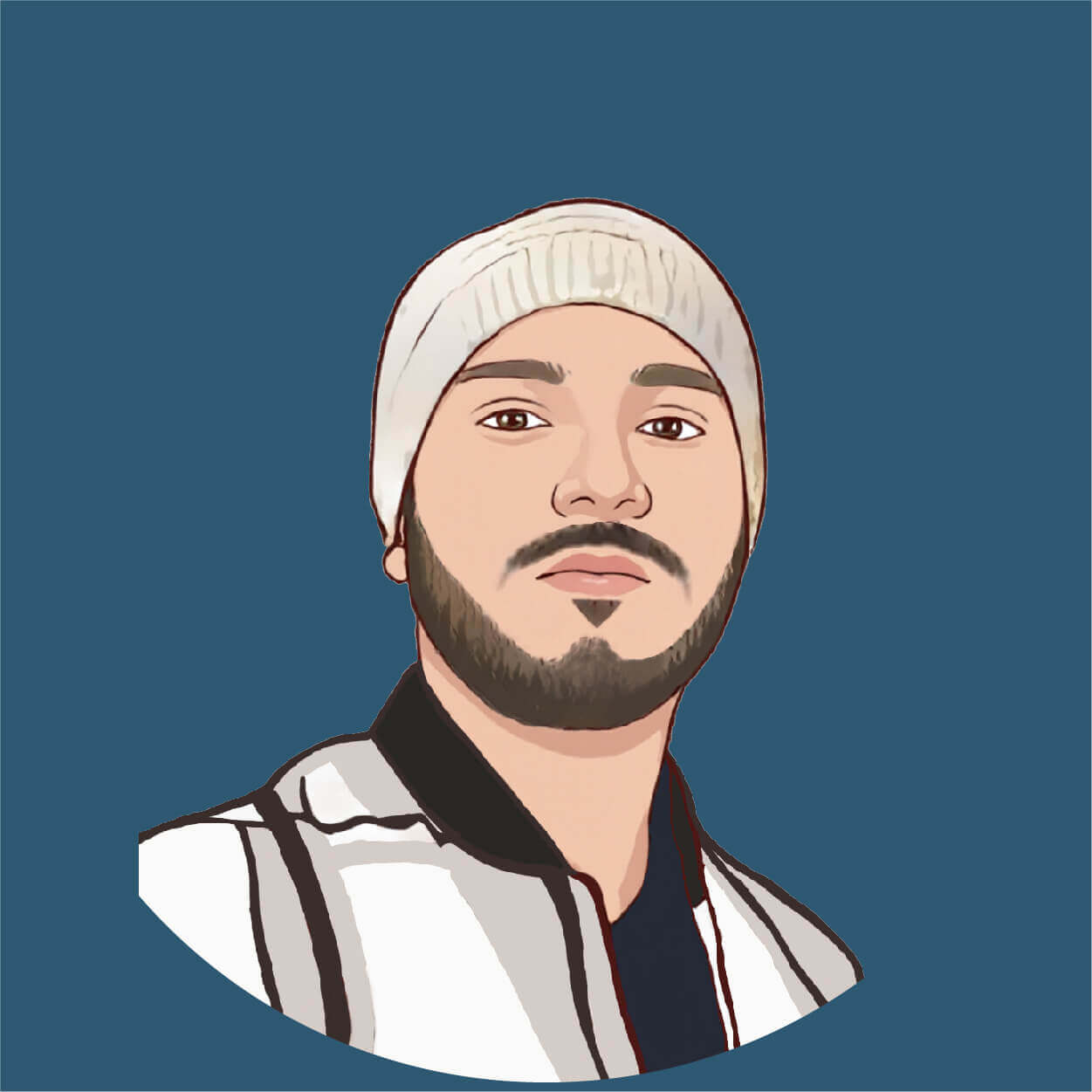
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.