PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Keywords in C++
In C++, keywords are predefined reserved identifiers that hold specific meanings and cannot be utilized as identifiers in our program. C++ reserves a set of keywords for its own use.
C++ allocates a collection of words for specialized purposes, often referred to as reserved words. In the table below, certain keywords are outlined, emphasizing that they cannot be employed in a program as variables or identifiers. If any attempt is made to use these reserved words mistakenly, the compiler will generate an error.
KEYWORDS | DESCRIPTION |
---|---|
asm: | Allows insertion of assembly language commands directly into code. |
auto: | Directs the compiler to deduce the type of a declared variable from its initialization expression. |
bool: | Represents a variable with two values: True and False. |
break: | Ends the execution of the nearest enclosing loop or conditional statement. |
case: | Used in a switch statement to test a variable against a list of values. |
catch: | Indicates the catching of an exception in a program. |
char: | Represents a data type for single characters. |
class: | A blueprint for a user-defined data type. |
const: | Declares a constant in C++. |
const_cast: | Used to cast away the constness of variables. |
continue: | Skips the code in between and forces the next iteration of a loop to take place. |
default: | Optional case in a switch statement for tasks when none of the cases is true. |
delete: | Used to delete dynamically allocated memory. |
double: | Allocates memory for floating-point numbers. |
do: | Represents a do...while loop that tests the condition at the bottom of the loop. |
dynamic_cast: | Converts pointers from parent to child types. |
else: | Optional clause in an if statement executed when the Boolean expression is false. |
enum: | Creates enumerations for a new, simple type. |
explicit: | Specifies whether user-defined type conversions are explicit or implicit. |
export: | Marks a template definition as exported. |
Note:
In C++ programming, it's essential to recognize that keywords are case-sensitive. This means that the compiler distinguishes between uppercase and lowercase letters when interpreting the language's reserved words. For instance, "if," "If," and "IF" are treated as distinct keywords.
KEYWORDS | DESCRIPTION |
---|---|
extern: | Gives a reference of a global variable visible to all program files. |
false: | Represents one of the two values for a bool type. |
float: | Stores real numbers or floating-point numbers. |
for: | Repetition control structure for efficiently writing loops. |
friend: | A function that can access non-public members of a class. |
goto: | Allows the program's execution flow to jump to a specified location within the function. |
if: | A conditional statement with an optional else clause. |
inline: | Creates an inline function. |
int: | Stores integer values. |
long: | Represents a data type that may occupy more storage than int. |
mutable: | Allows a member of an object to override const member functions. |
namespace: | Mechanism for grouping features in a program. |
new: | Allocates memory dynamically for any data type. |
operator: | A symbol that tells the compiler to perform specific manipulations. |
private: | A member variable or function inaccessible from outside the class. |
protected: | Similar to private but can be accessed in derived classes. |
public: | Accessible from anywhere outside the class within a program. |
register: | Defines local variables stored in a register instead of RAM. |
Note:
In C++ programming, the case sensitivity applies not only to keywords but also to identifiers—names given to variables, functions, classes, and other user-defined entities. Therefore, "MyVariable" and "myvariable" would be considered two separate identifiers in C++.
KEYWORDS | DESCRIPTION |
---|---|
reinterpret_cast: | Converts a pointer to a new designated type. |
return: | Used to return values from a program or function. |
short: | Represents integers that may occupy fewer bytes of memory than int. |
signed: | A data type that can be int or char, with positive, negative, or zero values. |
sizeof: | Computes the amount of memory in bytes. |
static: | Keeps a local variable in existence during the program's lifetime. |
static_cast: | Performs narrowing conversion and silences compiler warnings. |
struct: | Similar to class but with default public access to members. |
switch: | Allows testing a variable for equality against a list of values. |
template: | Enables defining a family of functions or classes operating on different types. |
this: | A pointer giving every object access to its own address. |
throw: | Used to throw exceptions when a problem occurs. |
true: | Represents one of the two values for a bool type. |
try: | Identifies a block of code for which exceptions will be caught. |
typedef: | Creates a new name for an existing type. |
typeid: | Returns information about an object's data type. |
typename: | Replaces class in a template definition in some C++ compilers. |
union: | Defines a single location with many different field names. |
unsigned: | A data type that can be int or char, always positive or zero. |
using: | Informs the compiler that the subsequent code is using names in the specified namespace. |
virtual: | Used to create virtual functions in a base class. |
void: | Represents the absence of any data type, returning no value to the program. |
volatile: | Informs the compiler that a variable's value may change in unspecified ways. |
wchar_t: | Represents wide characters. |
while: | Represents a do...while loop that tests the condition at the bottom of the loop. |
A C++ identifier serves as a designation for a variable, function, class, module, or any other user-defined element. It commences with a letter from A to Z or a to z, or an underscore (_), followed by zero or more letters, underscores, and digits (0 to 9).
In C++, the use of punctuation characters such as @, $, and % within identifiers is not permitted.
While mathematicians may find single-letter names like x sufficient for their variables, programmers are encouraged to adopt longer, more descriptive variable names. For instance, names such as altitude, sum, and User_name are preferable to the more concise but less informative options like a, s, and u. A variable's name should convey its purpose within the program, contributing to enhanced readability for human understanding.
As mentioned earlier, within C++ programming, identifiers, which are the names assigned to variables, functions, classes, and other user-defined entities, exhibit case sensitivity. Consequently, in C++, "MyVariable" and "myvariable" are recognized as distinct identifiers.
C++ has some important rules for naming variables. It's essential to understand these basic rules before you create any variable in C++ programming.
- An identifier must contain at least one character.
- The first character must be an alphabetic letter (upper or lower case) or an underscore.
- Subsequent characters may be alphabetic characters (upper or lower case), underscores, or digits (0 to 9).
- No other characters, including spaces, are permitted in identifiers.
- Reserved words cannot be used as identifiers.
- C++ is case-sensitive; therefore, "Temp" and "temp" are distinct identifiers.
Programmers are advised to use descriptive and meaningful variable names to enhance code readability. Following these rules ensures the proper creation of identifiers in C++ programming.
Valid Names:
AddNumbers , sum , Omar , Move_left , a_001 , totalAge, _temp, goto_number14
Invalid Names:
Sum of Numbers ( contains whitespace ), 13UserName ( starting with number ), goto ( using keywords ),
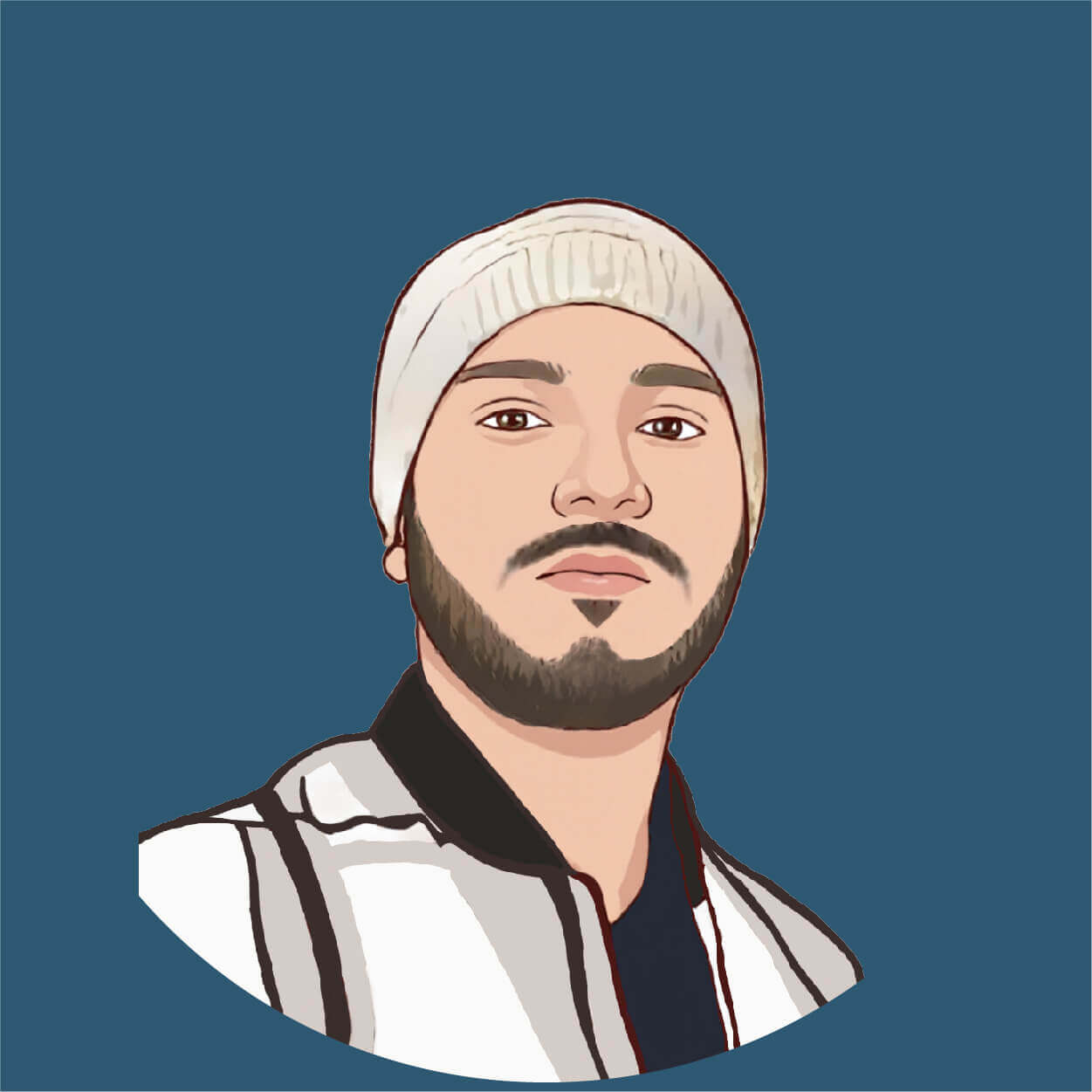
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.