PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
DATA ABSTRACTION:
Object-Oriented Programming incorporates a key feature known as Data Abstraction. This concept involves presenting only the essential information to the external world while concealing the underlying details, essentially providing the necessary information without revealing intricacies.
Abstraction selectively reveals pertinent details while hiding internal complexities from users. For instance, when using a laptop, we interact with its functionalities without needing to understand its inner workings. Similarly, when the laptop is locked, personal information remains inaccessible until the correct password is entered, showcasing abstraction in action.
Let's explore another real-life example to grasp Data Abstraction better. Consider a laptop: we can turn it on/off, browse the internet, watch movies, add external components, adjust volume, and even code on it. Despite utilizing these functionalities, we typically don't delve into the laptop's internal mechanisms or how it establishes internet connections. This encapsulation of internal workings from users exemplifies Data Abstraction in action.
In our Array Tutorial, we utilized the Sort()
function without concerning ourselves with the underlying sorting algorithm it employs. This abstraction shields users from implementation details, allowing them to utilize the function without being affected by potential changes in the underlying implementation.
In C++, classes facilitate robust Data Abstraction by offering a public interface for interacting with objects while concealing internal implementations.
In C++, classes enable the creation of abstract data types (ADTs). For instance, we can use the cout
object from the Ostream
class to stream data to the standard output:
#include<iostream>
using namespace std;
int main()
{
cout << "Info Brother!" << endl;
return 0;
}
Here, we focus solely on the public interface of cout
without needing to comprehend its internal mechanisms, allowing for potential changes in its implementation without affecting its usage.
In C++, access labels define the abstract interface of a class. A class may include zero or more access labels:
Members labeled as public are accessible to all parts of the program, defining the data-abstraction view of the type.
Members labeled as private or protected are inaccessible to external code, concealing implementation details.
There are no limitations on the frequency of access labels, and each label specifies the access level of subsequent member definitions until encountering another access label or the closing brace of the class body.
Let's illustrate how abstraction works with an example demonstrating data hiding within a class.
#include<iostream>
using namespace std;
class Hide
{
private:
int x, y;
public:
Hide()
{
x = 10;
y = 20;
}
void Show()
{
cout << "The value of x is: " << x << endl;
cout << "The value of y is: " << y;
}
};
int main()
{
Hide obj;
obj.Show();
return 0;
}
The presence of the keyword public
, private
or protected
before member data or functions dictates the accessibility of subsequent members. If these access types differ, explicit mention of access type is necessary for each member.
Removing the public
keyword from the previous example results in errors since attempting to access private members outside the class is prohibited, reflecting the enforcement of abstraction principles.
- Reduces the need for writing low-level code.
- Eliminates the requirement to specify register-level instructions or hardware details.
- Prevents code duplication, enhancing efficiency.
- Facilitates seamless changes in internal implementations without impacting users.
Abstraction segregates code into interface and implementation, ensuring that modifications in implementation don't affect the interface. This allows programs using these interfaces to remain unaffected, merely requiring recompilation with the latest implementation.
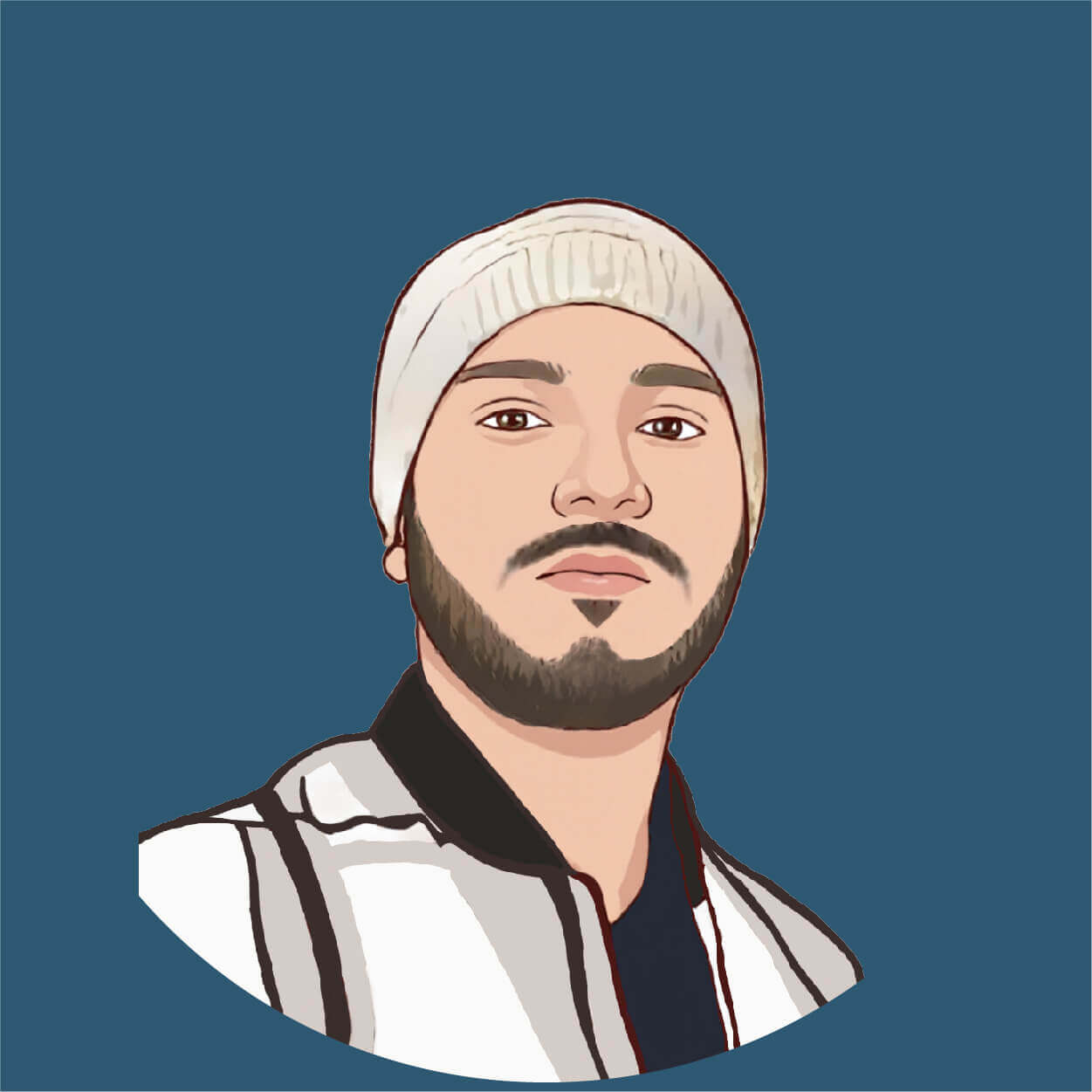
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.