PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
POINTERS AND ARRAYS:
In C++, there exists a close connection between pointers and arrays, often making them interchangeable. The identifier of an array corresponds to the address of its first element, mirroring how a pointer points to the address of the first element it refers to. Essentially, they represent the same concept.
Before diving into this tutorial, it's highly recommended to go through the C++ Pointers Tutorial to grasp the concepts discussed here effectively. Understanding pointers is crucial to comprehend the relationship between pointers and arrays.
Visualize an array as a city block where each element of the array resembles a house on that block. The elements of the array are indexed based on the number of houses from the starting point of the block (usually the street corner).
For instance, if the house at the street corner is labeled as 220 Main Street, the subsequent houses would be 221 Main Street, 222 Main Street, and so forth. In array terminology, we denote cityBlock[0]
as 220 Main Street, cityBlock[1] as 221 Main Street, and so forth.
Translating this analogy to computer memory, consider an array named Name consisting of 32 1-byte characters. If the first byte of this array resides at address 0x110, the array spans addresses 0x110 through 0x12F. Accordingly, Name[0]
is located at address 0x110, Name[1]
at 0x111, Name[2]
at 0x112, and so on.
Extending this analogy to pointer variables, consider the expression:
ptr = &Name[0];
Here's a simple example:
double *ptr;
double balance[10];
ptr = balance;
In this example, "balance" acts as a pointer to &balance[0]
, which is the address of the first element of the array "balance". Thus, the program assigns ptrthe address of the first element of "balance".
It's valid to use array names as constant pointers and vice versa. Hence, *(balance + 4)
is a legitimate way to access the data at balance[4]
. Once we store the address of the first element in ptr, we can access array elements using *ptr
, b*(ptr + 1)
, *(ptr + 2)
, and so forth.
Below is an example illustrating all the discussed concepts:
#include <iostream>
using namespace std;
int main() {
int balance[5] = {10, 20, 30, 40, 50}; // an array with 5 elements.
int *ptr; // declaring a pointer.
ptr = balance; // assign the address of the first element of the array to ptr
// output each array element's value
cout << "Array values using pointer " << endl;
for (int i = 0; i < 5; i++) {
cout << "*(ptr + " << i << ") : ";
cout << *(ptr + i) << endl;
}
cout << "\n\n Array values using balance as address " << endl;
for (int i = 0; i < 5; i++) {
cout << "*(balance + " << i << ") : ";
cout << *(balance + i) << endl;
}
return 0;
}
In the above example, ptr
is a pointer to an integer, meaning it can store the address of a variable of type int
. Once we have the address stored in ptr
, *ptr
retrieves the value available at that address, as demonstrated in the example above.
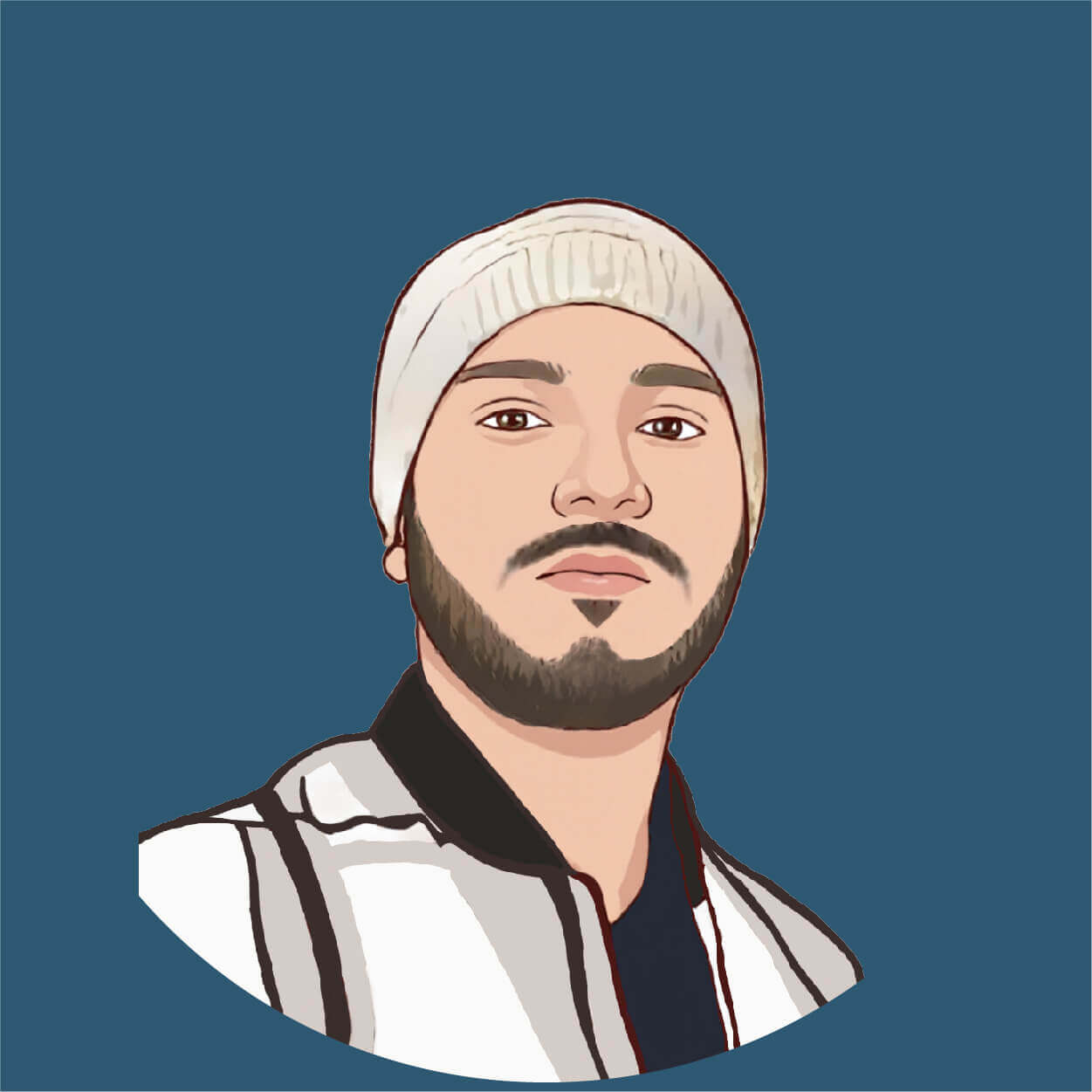
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.