PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
New Operator in C++:
The new
operator in C++ is used to allocate memory for a variable or an object at runtime. When the new
operator is used, the variables or objects are treated as pointers to the memory location allocated to them.
To allocate memory for a single element of a specified data type (which can be a built-in data type, structure, or class), the new
operator is used in the following manner:
// Request memory allocation for variable Variable_Name
Data_type* Variable_Name = new Data_type;
// Or
// Request memory allocation for variable Variable_Name and assign 10 to it
Data_type* Variable_Name = new Data_type(10);
To allocate memory for an array, the new
operator is used as follows:
Data_type* Variable_Name = new Data_type[Size_of_Array];
Here is a simple example demonstrating how to use the new
operator to allocate dynamic memory in C++:
#include <iostream>
using namespace std;
int main() {
int* ptr; // Pointer to int
int num; // To store the total number of elements
cout << "Enter the total number of elements to input: " << endl;
cout << "Total Number: ";
cin >> num;
// Dynamic memory allocation for an array of size num
ptr = new int[num];
cout << "\nEnter your " << num << " elements:" << endl;
for (int i = 0; i < num; i++) {
cout << "Element #" << i + 1 << ": ";
cin >> ptr[i];
}
cout << "\nYour entered elements are:" << endl;
for (int i = 0; i < num; i++) {
cout << ptr[i] << " | ";
}
// Releasing the allocated memory
delete[] ptr;
return 0;
}
To allocate dynamic memory using the new
operator, a pointer is required. This pointer will hold the address of the allocated memory. In the example above, we declare a pointer to int
named ptr
and allocate enough memory to store integers. If the requested memory exceeds the available heap space, an error may occur. Therefore, it is advisable to check for errors during memory allocation.
Here's an improved version of the above example with error checking:
#include <iostream>
using namespace std;
int main() {
int* ptr; // Pointer to int
int num; // To store the total number of elements
cout << "Enter the total number of elements to input: " << endl;
cout << "Total Number: ";
cin >> num;
// Dynamic memory allocation for an array of size num
ptr = new (nothrow) int[num];
if (ptr != nullptr) { // Error checker
cout << "\nEnter your " << num << " elements:" << endl;
for (int i = 0; i < num; i++) {
cout << "Element #" << i + 1 << ": ";
cin >> ptr[i];
}
cout << "\nYour entered elements are:" << endl;
for (int i = 0; i < num; i++) {
cout << ptr[i] << " | ";
}
// Releasing the allocated memory
delete[] ptr;
} else { // If an error occurs while allocating memory
cout << "An error occurred while allocating memory." << endl;
}
return 0;
}
In the improved example, the nothrow
keyword ensures that if memory allocation fails, nullptr
is returned instead of throwing an exception. The program checks if ptr
is nullptr
and handles the error appropriately.
The delete
operator is used to free the allocated memory when it is no longer needed. It is the programmer's responsibility to release the allocated memory. Here's how to use the delete
operator:
// Allocate memory for a single element
Data_type* Variable_Name = new Data_type;
delete Variable_Name; // Release memory
// Allocate memory for an array
Data_type* Variable_Name = new Data_type[Size_of_Array];
delete[] Variable_Name; // Release memory
Here is another example that demonstrates dynamic memory allocation using the new
operator in a simple marks calculator program:
#include <iostream>
#include <cstdlib> // exit() function
using namespace std;
int main() {
cout << "\t\t\tMARKS CALCULATOR" << endl;
int no, i, ans; // no = total number of subjects, i = loop counter
// Pointer to store subject marks, sum to calculate total, percentage
float *subject, sum = 0, percentage;
cout << "\n\nEnter the total number of subjects: ";
cin >> no;
// Allocate memory for subjects using new operator
subject = new (nothrow) float[no];
if (subject != nullptr) { // Error checker
cout << "\n\nEnter subjects marks:" << endl;
for (i = 0; i < no; i++) { // Loop to get data
cout << "Subject No " << (i + 1) << ": ";
cin >> subject[i];
sum += subject[i];
}
percentage = (sum / (100 * no)) * 100;
// Display data
cout << "\n\n\t\tPress 1 to Display:" << endl;
cout << "\t\tPress 2 to Exit:" << endl;
cout << "\nYour Ans: ";
cin >> ans;
cout << "\n\n";
if (ans == 1) { // Answer checker
cout << "\tSubject: \tMax:" << endl;
cout << "\n";
for (i = 0; i < no; i++) { // Loop to read and print data
cout << "\tSubject No " << (i + 1) << "\t" << subject[i] << endl;
}
cout << "\t==================" << endl;
cout << "\n\tThe Sum is: \t" << sum << endl;
cout << "\n\tPercentage is: \t" << percentage << " %" << endl;
} else {
cout << "Thank you...!!" << endl;
exit(0);
}
// Release the allocated memory
delete[] subject;
} else {
cout << "Sorry...!! Memory not allocated." << endl;
cout << "Check your code again." << endl;
exit(0);
}
return 0;
}
In the example above, the program allocates memory dynamically to store subject marks, calculates the sum and percentage, and displays the results. The memory is released using the delete[]
operator after it is no longer needed. If memory allocation fails, the program handles the error gracefully.
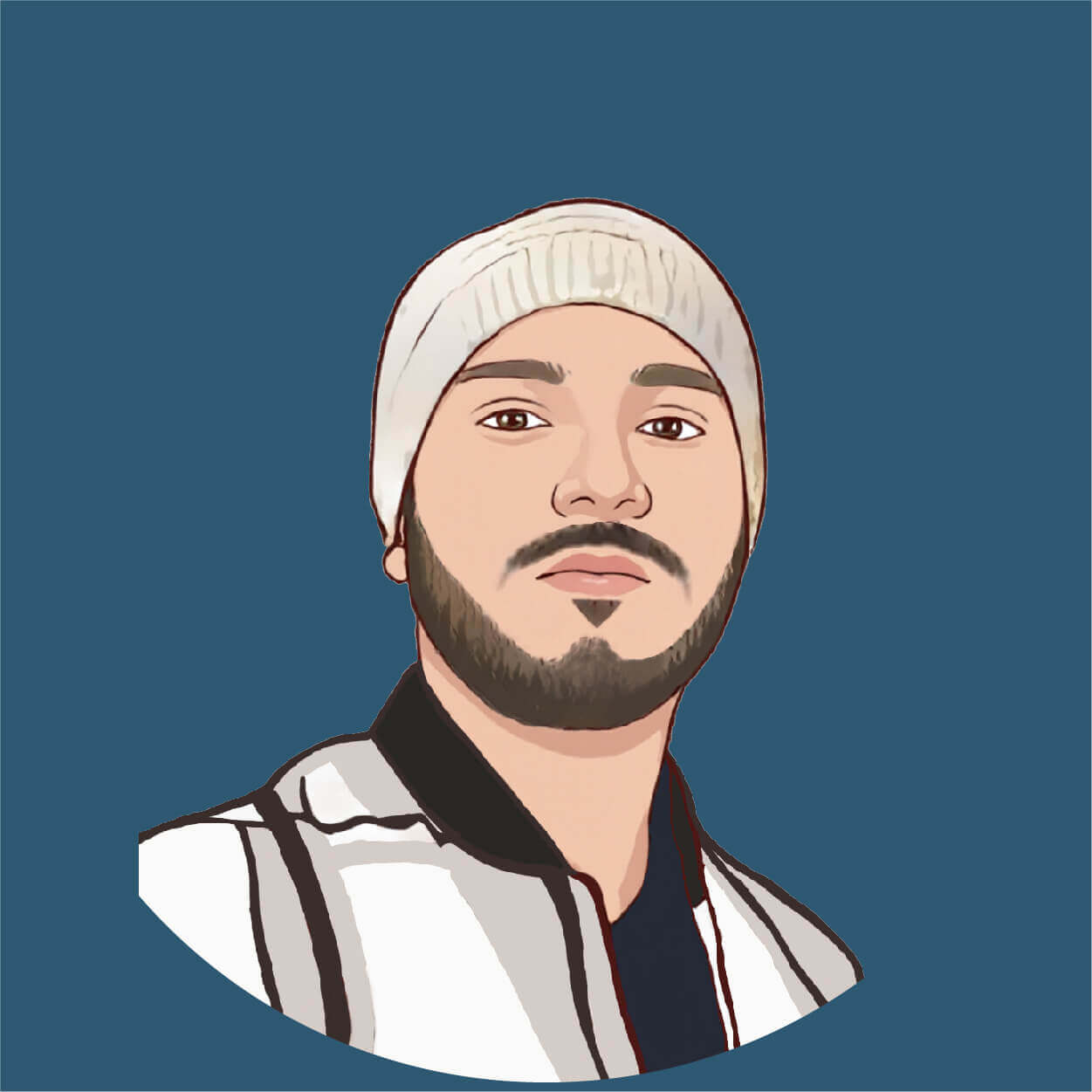
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.