PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Programming
A function in C++ is a collection of statements designed to perform a specific task. It receives input, processes it, and produces an output. Functions are pivotal in breaking down complex computing tasks into smaller, more manageable units, fostering code reuse and modular programming practices. Every C++ program comprises at least one function, typically the main()
function, with the option to define additional functions.
Imagine you're running a bakery, and your goal is to efficiently produce different types of pastries. Each pastry consists of common elements like dough, filling, and toppings, but they vary in specific ingredients and preparation methods.
- Creating Pastries: Your primary task is to create various pastries, such as croissants, muffins, and danishes. Each pastry type requires a unique recipe and preparation process.
- Modular Approach: To streamline operations, you break down the baking process into smaller, modular tasks. For example, you have separate functions for preparing dough, making fillings, and adding toppings.
- Functionality and Reusability: Functions represent these modular tasks. You can reuse functions across different pastry recipes, promoting code efficiency and consistency. For instance, the function to knead dough remains the same for all pastries that require dough preparation.
- Function Invocation: When assembling a pastry, you call specific functions to execute the necessary steps. For instance, when making a croissant, you invoke functions for rolling out dough, adding filling, and shaping.
- Flexibility and Adaptability: Functions allow you to adapt to changing requirements easily. If you introduce a new pastry type, you can create additional functions or modify existing ones without rewriting the entire baking process.
In C++, you would represent each baking task as a function. For example:
// Function to prepare dough
void prepareDough() {
// Code to mix ingredients and knead dough
}
// Function to make filling
void makeFilling() {
// Code to prepare filling ingredients
}
// Function to add toppings
void addToppings() {
// Code to sprinkle toppings on pastries
}
// Function to make croissants
void makeCroissants() {
prepareDough();
makeFilling();
addToppings();
// Code specific to croissant preparation
}
// Function to make muffins
void makeMuffins() {
prepareDough();
makeFilling();
// Code specific to muffin preparation
}
In this example, each function represents a specific task in the baking process. By organizing code into functions, you can easily manage and reuse individual steps across different pastry recipes. This modular approach enhances code readability, maintainability, and scalability, mirroring the efficiency and adaptability of a well-organized bakery.
C++ functions can be categorized into two types:
- Functions that return a value.
- Functions that do not return a value (void functions).
For instance, a function might calculate the sum of two numbers and return the result, while another function might simply display information without returning any value.
To create a function in C++, we follow three steps:
A function declaration in C++ provides the compiler with information regarding the function's name, return type, and parameters, while the implementation of the function's body can be specified elsewhere. In C++, each function typically consists of a header, or function declaration, comprising three essential components:
return-type function-name (parameter-list);
A function declaration includes three essential elements:
- Return Type: Indicates the type of value returned by the function. Use void if the function does not return a value.
- Function Name: The identifier for the function, which, along with the parameter list, forms the function's signature.
- Parameter List: Specifies the types and names of variables passed to the function. Parameters are optional.
Presented below is a function declaration aimed at calculating the sum of two numbers and returning the result.
int sum(int num1 , int num2);
In function declarations, the names of parameters hold no significance; only their types are necessary. Hence, the subsequent declaration is equally valid.
int sum(int, int);
Alternatively, we may have a function designed solely for displaying text. Since it does not return any value, its return type would be void, and it would require no parameters, as exemplified in the provided example.
void message( );
A function definition consists of a function header and a function body. The header reiterates the function's signature, while the body contains the statements defining the function's behavior.
void message( ); {
//function body
}
To call a function, simply use its name followed by parentheses. If the function requires parameters, provide them within the parentheses. Upon invocation, the function executes its defined tasks.
Let's explore a straightforward example to create and utilize functions in C++. In this example, we'll develop a simple program to display a message. Since this program doesn't need to return any value, we'll use the "void" keyword to indicate that.
Note:
If we define a function after the main() method, it's essential to include a function prototype or declaration inside the main() method before calling it, as illustrated in the following example.
#include <iostream>
using namespace std;
main() {
// function prototype Or Declaration
void message();
cout << "Let's call our message function here." << endl;
message(); //calling function.
return 0;
}
//Function Definition:
void message() {
cout << "Greetings! " << endl;
cout << "I'm a function. My purpose is to showcase messages for you. " << endl;
}
If we define a function before the main()
method, there is no need to include a function prototype or declaration inside the main() method before calling it.
Here's another helpful example of a function. In this case, we'll define the function before the main()
function, eliminating the need for a function declaration or prototype. This function will take two integers as parameters and return their sum, as demonstrated below.
#include <iostream>
using namespace std;
// function
int sum(int a, int b)
{
int result = 0;
result = a + b;
}
main()
{
int a = 150;
int b = 14;
// Invoking function with arguments.
cout << " The sum of a and b is: " << sum(a, b) << endl;
return 0;
}
We can also directly pass values as arguments instead of variable names, as demonstrated in the example below.
#include <iostream>
using namespace std;
// function
int sum(int a, int b)
{
int result = 0;
result = a + b;
}
main()
{
// Invoking function with arguments.
cout << " The sum of a and b is: " << sum(150, 14) << endl;
return 0;
}
When a function needs to work with arguments, it has to declare variables to hold these argument values. These variables are known as formal parameters of the function. They act like any other local variables within the function and are created when the function starts and destroyed when it finishes.
When we call a function, there are three different ways we can provide arguments to that function.
- Call by Value: Arguments are passed by value, creating temporary copies within the function.
- Call by Pointer: Arguments' addresses are passed, allowing modifications to affect the original values. (Read More...)
- Call by Reference:References to arguments are passed, enabling direct access and modification. (Read More...)
When writing a program, it's crucial to consider the possibility of reusing it in the future and to structure it in a manner that allows it to solve related problems. Functions play a pivotal role in achieving reusability. By creating advanced functions, we can incorporate them into other programs. Functions enable us to break down our code into smaller, more manageable pieces, making it both more readable and efficient.
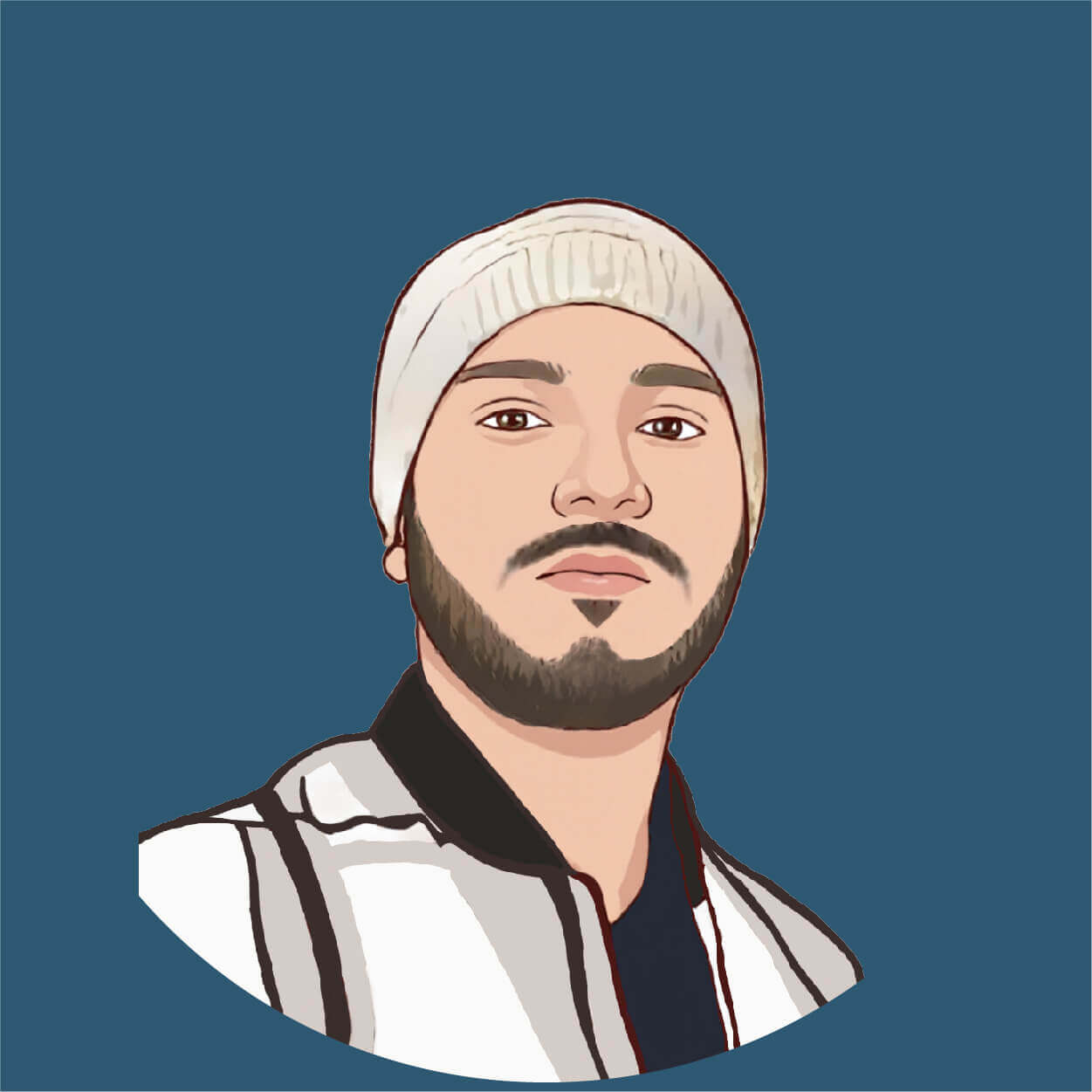
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.