PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Pointers vs. Arrays:
Pointers and arraysshare a close relationship, often being interchangeable in various scenarios. For instance, a pointer referencing the start of an array can navigate through the array using either pointer arithmetic or array-style indexing. Below is a program illustrating this concept:
#include <iostream>
using namespace std;
const int MAX = 3;
int main() {
int var[MAX] = {10, 100, 200};
int *ptr; // Pointer to int.
// Assigning array address to pointer.
ptr = var;
for (int i = 0; i < MAX; i++) {
cout << "Address of var[" << i << "] = ";
cout << ptr << endl;
cout << "Value of var[" << i << "] = ";
cout << *ptr << endl;
// Move to the next location
ptr++;
}
return 0;
}
However, despite their interchangeability, pointers and arrays aren't entirely equivalent. Consider the following program:
#include <iostream>
using namespace std;
const int MAX = 3;
int main() {
int var[MAX] = {10, 100, 200};
for (int i = 0; i < MAX; i++) {
*var = i; // This is correct syntax
var++; // This is incorrect.
}
return 0;
}
While applying the pointer operator *
to var
is acceptable, modifying its value directly is illegal. This is because var
is a constant pointing to the start of an array and cannot be altered. Although an array name can function as a pointer constant, it can still participate in pointer-style expressions as long as it remains unaltered. For instance, the statement below assigns the value 500 to var[2]
:
*(var + 2) = 500;
This statement is valid since var
remains unchanged.
In most cases, accesses using pointers and arrays can be treated similarly, except for a few exceptions:
1: The sizeof() Operator:- sizeof(array): Returns the memory used by all elements in the array.
- sizeof(pointer): Returns the memory used by the pointer variable itself.
2: The & Operator:
- &array: Alias for &
array[0]
, returning the address of the first element in the array. - &pointer: Returns the address of the pointer.
3: Initialization of a character array with string literals:
- char array[]="abc": Sets elements in the array to 'a', 'b', 'c', and '\0'.
- char *pointer="abc": Sets pointer to the address of the "abc" string, possibly stored in read-only memory.
4: Pointer variables can be assigned values, unlike array variables:
int a[10];
int *p;
p = a; // Legal
a = p; // Illegal
int a[10];
int *p;
p++; // Legal
a++; // Illegal
Let's illustrate these concepts with a simple example:
#include <iostream>
using namespace std;
int main() {
float arr[5]; // Array
float *ptr; // Pointer
cout << "Displaying addresses using arrays:" << endl;
for (int i = 0; i < 5; ++i) {
cout << "&arr[" << i << "] = " << &arr[i] << endl;
}
// ptr = &arr[0]
ptr = arr;
cout << "\nDisplaying addresses using pointers:" << endl;
for (int i = 0; i < 5; ++i) {
cout << "ptr + " << i << " = " << ptr + i << endl;
}
return 0;
}
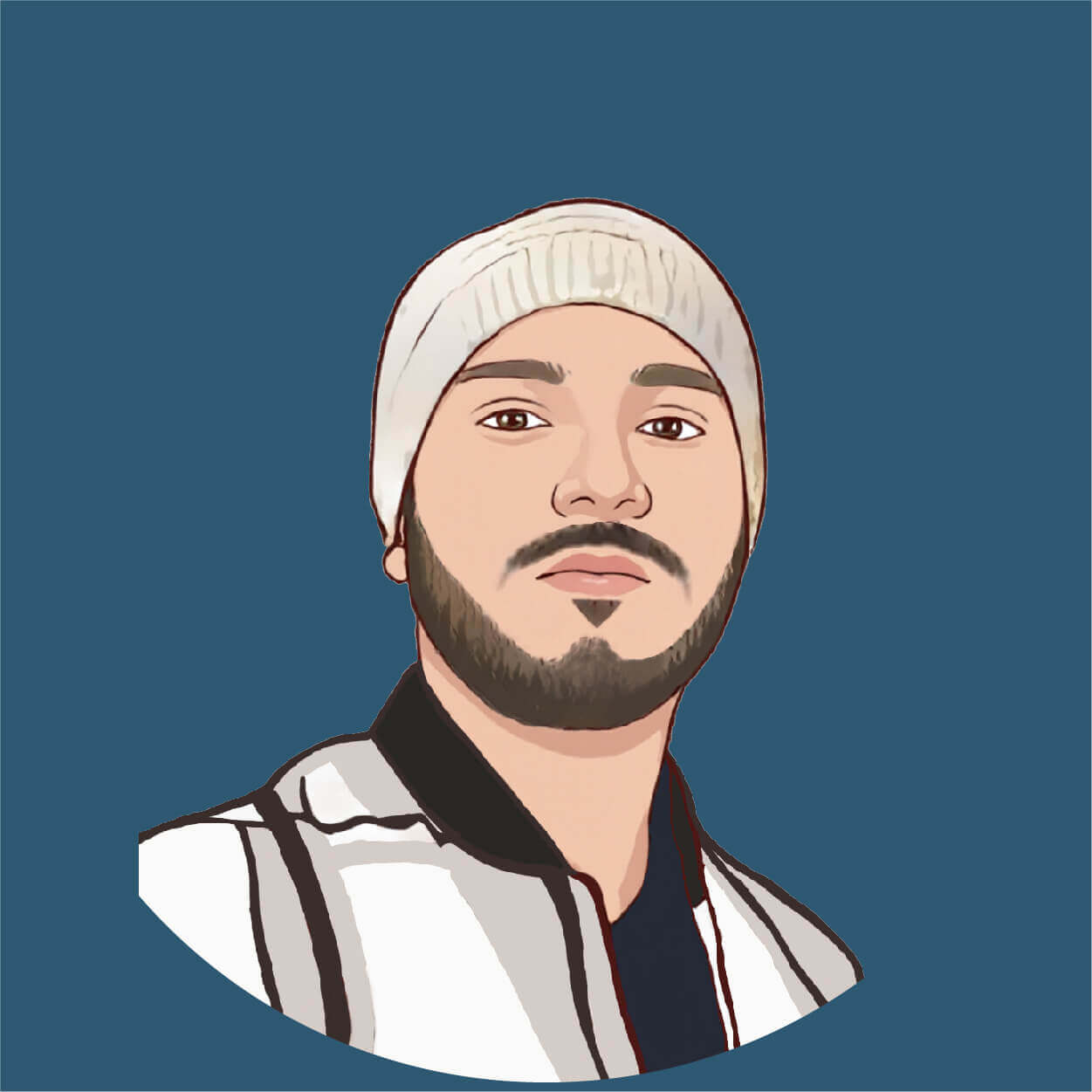
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.