PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
C++ Overview
C++ is a powerful general-purpose programming language that is widely used for developing operating systems, game engines, high-performance and resource-intensive applications, and many other software projects. It was created in the early 1980s by Bjarne Stroustrup and is an extension of the C programming language. C++ introduces object-oriented programming (OOP) features, such as classes and inheritance, while still maintaining the low-level programming capabilities of C.
In this tutorial, we will provide an overview of the C++ programming language, covering its key features, syntax, and concepts.
- Every C++ program begins with a main function, which serves as the entry point of the program.
- C++ programs are composed of statements, which are instructions that perform specific actions.
- Statements are terminated with a semicolon (;) and can be organized into blocks using curly brackets ({}).
- Variables are used to store and manipulate data.
- C++ provides several built-in data types, including integers
int
, floating-point numbersfloat
,double
, characterschar
, and booleansbool
, among others. - Variables must be declared before they can be used, specifying their type and name.
- C++ supports a wide range of operators for performing various operations such as arithmetic, assignment, comparison, logical, and bitwise operations.
- Arithmetic operators (+, -, *, /, %) allow you to perform mathematical computations.
- Comparison operators (==, !=, <, >, >=, >=) are used to compare values and return boolean results.
- Control flow statements enable you to alter the flow of program execution based on specific conditions.
- Conditional statements, such as
if
,else if
, andelse
, are used to execute different blocks of code depending on certain conditions. - Looping statements, such as
for
,while
, anddo-while
, allow you to repeat a block of code multiple times until a specified condition is met.
- Functions in C++ allow you to divide your code into smaller, reusable blocks that perform specific tasks.
- Functions have a return type, a name, and a body containing the code to be executed.
- You can pass arguments to functions to provide input data, and functions can return values as a result.
- Arrays are used to store multiple values of the same type in contiguous memory locations.
- Pointers are variables that store the memory address of another variable.
- C++ provides powerful features for manipulating arrays and pointers, allowing efficient memory management and array access.
- C++ supports object-oriented programming, which allows you to create classes to represent entities and define their properties (data members) and behaviors (member functions).
- Objects are instances of classes and can be created to utilize the defined properties and behaviors.
- OOP concepts like encapsulation, inheritance, and polymorphism are extensively used in C++.
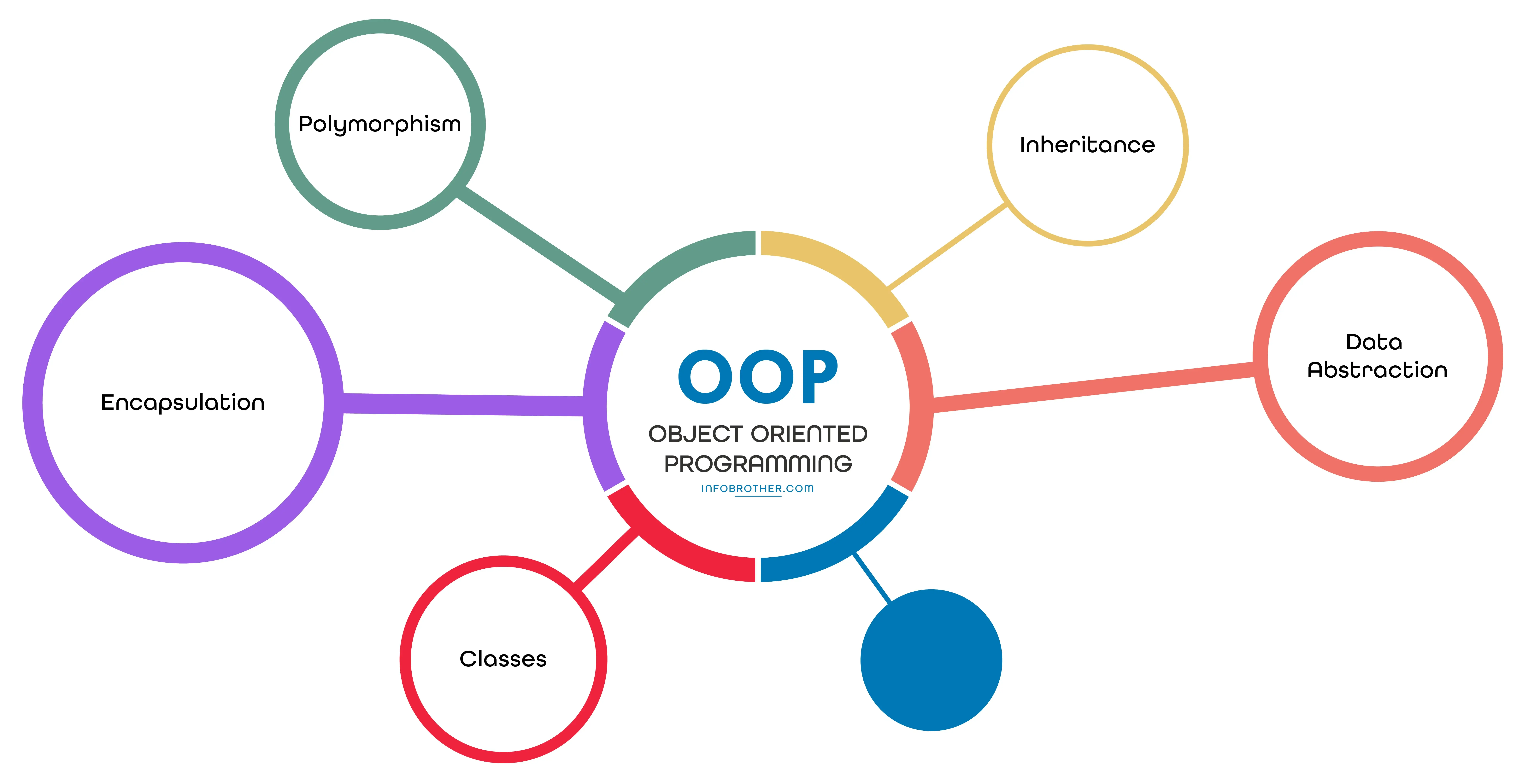
Object-Oriented Programming (OOP) is a programming paradigm that focuses on the concept of objects. Objects are instances of classes, which are user-defined data types that encapsulate both data (properties) and functionality (methods or member functions) into a single entity.
Encapsulation:Encapsulation is the practice of bundling data and its related operations (methods) within a class. It allows the class to hide its implementation details and only expose the necessary features through a well-defined interface. The idea is to achieve data abstraction, where the internal representation of an object is hidden from the outside world.
Inheritance:Inheritance is a mechanism where one class inherits the properties and behaviors of another class. The class being inherited from is called the base class or super class, and the class inheriting from it is called the derived class or sub class. Inheritance allows for code reuse and promotes the concept of hierarchy, where more specialized classes build upon generalized ones.
Polymorphism:Polymorphism means the ability to take many forms. In the context of OOP, polymorphism allows objects of different classes to be treated as instances of a common base class. Polymorphism is achieved through two main mechanisms: function overloading and function overriding. Function overloading enables a class to have multiple functions with the same name but different parameters, while function overriding allows a derived class to provide its own implementation of a method inherited from a base class.
Data Abstraction:Abstraction is a fundamental concept in OOP that involves simplifying complex systems by breaking them down into smaller, more manageable components. In C++, abstract classes and interfaces are used to define common attributes and behaviors that derived classes must implement. Abstract classes cannot be instantiated but serve as blueprints for creating derived classes.
Classes:Classes refers to the concept of building complex objects by combining simpler objects. In C++, classes can have member variables that are objects of other classes. This allows for the creation of more complex relationships between objects, enabling code organization and enhancing code reusability.
These OOP concepts in C++ promote code organization, modularity, reusability, and maintainability. They allow for building complex software systems by breaking them down into manageable and coherent classes, enabling better code structuring and facilitating collaboration among developers.
By understanding and utilizing these OOP concepts effectively, C++ developers can write more modular, extensible, and scalable code.
This tutorial provided an overview of the C++ programming language, covering its basic structure, variables, operators, control flow, functions, arrays, pointers, and object-oriented programming concepts. With this foundation, you can start exploring more advanced features of C++ and build complex and efficient software applications. Remember to practice and experiment with code examples to reinforce your understanding. HAPPY CODING :)
.
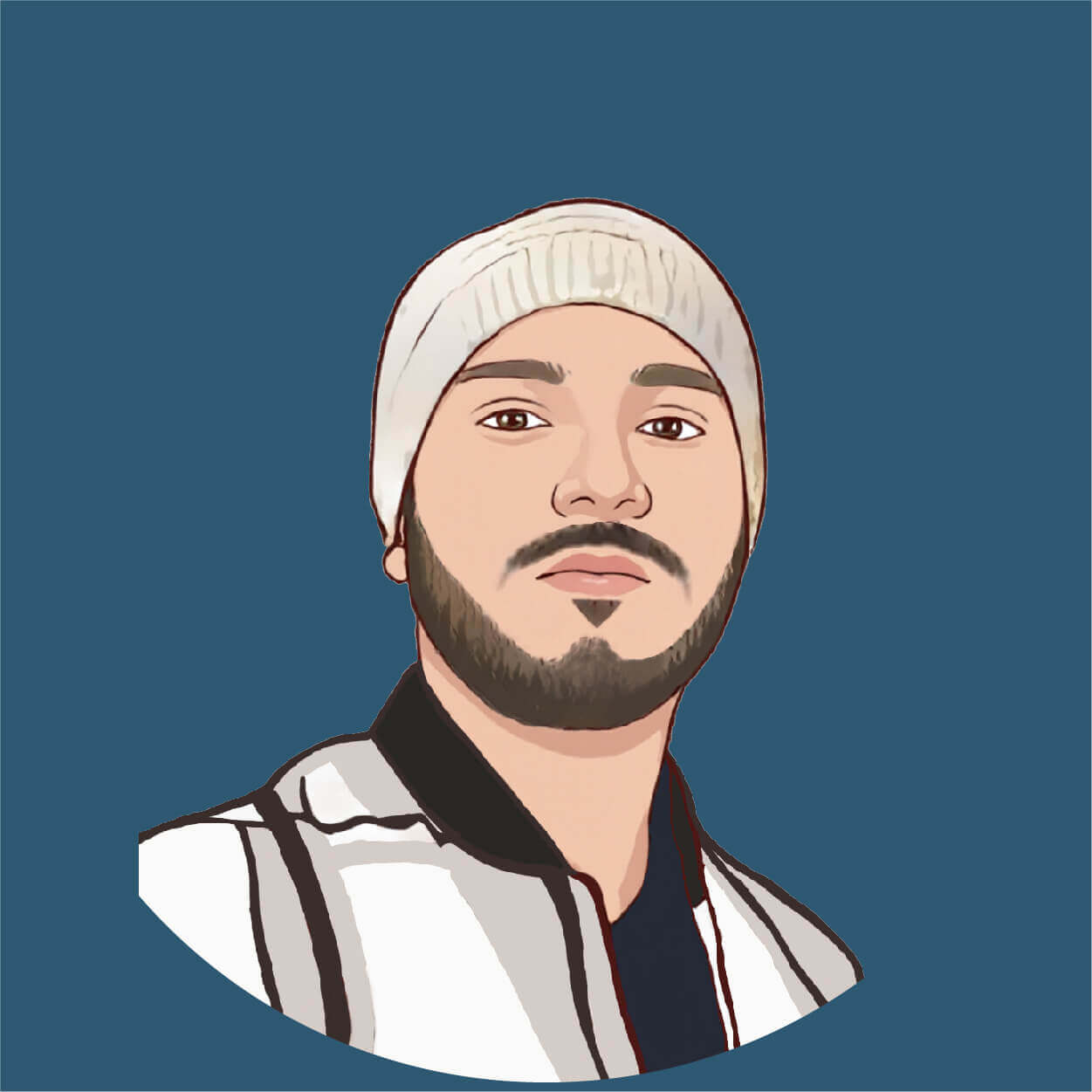
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.