PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Dynamic Memory Allocation Using calloc():
In our previous tutorials, we discussed dynamic memory allocation using the malloc()function. In this tutorial, we will explore dynamic memory allocation using the calloc()
function. The old C library <stdlib.h>
provides the calloc() function for allocating runtime memory.
Similar to the malloc()function, the calloc()
function allocates memory dynamically and returns a pointer to the first byte of the memory block of the specified size from the heap. If the requested space is unavailable, it returns NULL.
The main difference between malloc() and calloc() is that malloc() allocates a single block of memory without initializing it to zero, whereas calloc() allocates multiple blocks of memory and initializes them to zero.
The basic syntax for the calloc()
function is:
void *calloc(size_t num, size_t size);
In this syntax, num
is the number of elements to be allocated, and size
is the size of each element. The function returns a void pointer to the allocated memory. If insufficient memory is available, it returns NULL.
To return a pointer of a specific type instead of a void pointer, we can use a type cast operator on the return value. The syntax is as follows:
cast_type *ptr; // Pointer to the data type
ptr = (cast_type *)calloc(num, size);
//For Example
int *ptr;
ptr = (int *)calloc(num, sizeof(int));
In the above syntax, we declare a pointer to an integer. The calloc() function requires the size in bytes as an argument, so we use the sizeof()operator to allocate the exact size.
Let's look at an example to understand how this function works to allocate memory dynamically.
/* Example: Calloc() function */
#include <iostream>
#include <stdlib.h> // Required for calloc() function
using namespace std;
int main() {
int *ptr, *ptr2; // Ptr to allocate memory and record data, ptr2 to display data
int num, sum = 0; // num: number of elements, sum: total score to display
cout << "Enter the total number of elements to store: " << endl;
cout << "Total elements: ";
cin >> num;
ptr = (int *)calloc(num, sizeof(int));
ptr2 = ptr;
cout << "\nEnter your " << num << " elements to store: " << endl;
for (int i = 0; i < num; i++) {
cout << "Element #" << i << ": ";
cin >> *ptr;
sum += *ptr; // Add all entered numbers
ptr++;
}
cout << "\n\nYour elements are: " << endl;
for (int i = 0; i < num; i++) {
cout << "Element #" << i << ": " << *ptr2 << endl;
ptr2++;
}
cout << "And the sum is: " << sum << endl;
return 0;
}
The above example demonstrates a simple use of the calloc()
function to allocate memory dynamically. This function allocates enough memory from the heap to store some elements and then returns their sum. If the calloc()
function is unable to allocate the requested memory, it returns a NULL pointer, which points to nowhere. Using a NULL pointer can cause the program to crash, so it's important to include an error checker to verify memory allocation. Here's the revised example with an error checker:
/* Example: Calloc() function with error checking */
#include <iostream>
#include <stdlib.h> // Required for calloc() function
using namespace std;
int main() {
int *ptr, *ptr2; // Ptr to allocate memory and record data, ptr2 to display data
int num, sum = 0; // num: number of elements, sum: total score to display
cout << "Enter the total number of elements to store: " << endl;
cout << "Total elements: ";
cin >> num;
ptr = (int *)calloc(num, sizeof(int));
if (ptr == NULL) { // Error Checker
cout << "Error: calloc() is not able to allocate memory." << endl;
return 1; // Exit the program with an error code
}
ptr2 = ptr;
cout << "\nEnter your " << num << " elements to store: " << endl;
for (int i = 0; i < num; i++) {
cout << "Element #" << i << ": ";
cin >> *ptr;
sum += *ptr; // Add all entered numbers
ptr++;
}
cout << "\n\nYour elements are: " << endl;
for (int i = 0; i < num; i++) {
cout << "Element #" << i << ": " << *ptr2 << endl;
ptr2++;
}
cout << "And the sum is: " << sum << endl;
// Free the allocated memory
free(ptr2);
return 0;
}
Remember, memory allocated dynamically using the calloc() function comes from the heap. As the memory is from the heap, we are responsible for releasing it once it is no longer needed. To release the allocated memory, we utilize the free()
function, as demonstrated in the example above.
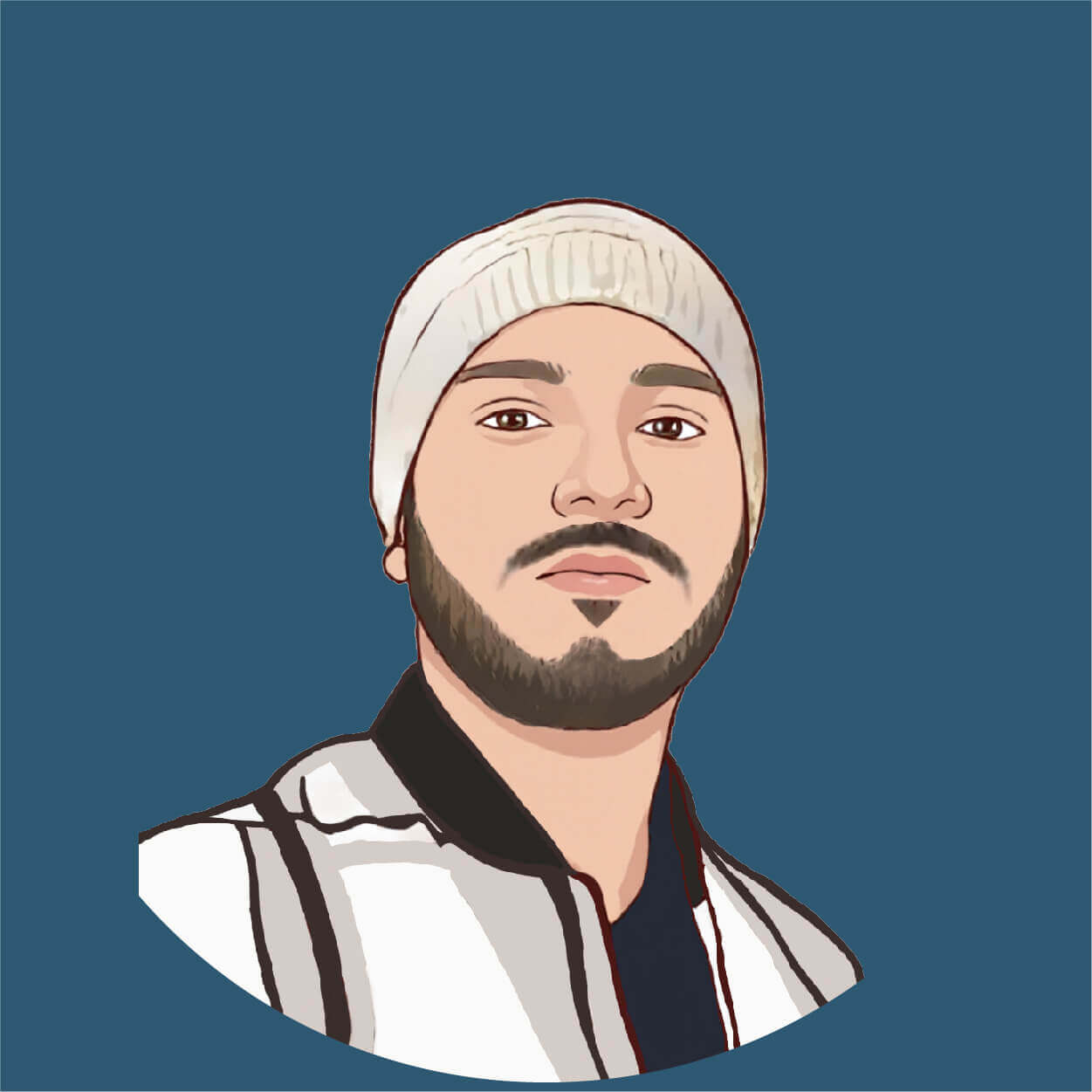
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.