PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
PASSING ARGUMENTS TO A FUNCTION:
The primary aim of passing arguments to a function is message passing, also referred to as communication between two functions - the caller and the called functions. There are three methods through which values can be passed to a function:
C++ supports all these three types of passing values to a function, whereas C supports only the first two types. The arguments used to send values to a function are known as input arguments. The arguments used to return results are known as output arguments, while those used to send and return results are known as input-output arguments. When passing values to a function, certain conditions should be fulfilled:
- The data type and number of actual and formal arguments should be the same in both the caller and called functions.
- Extra arguments are discarded if they are declared. If the formal arguments are more than the actual arguments, the extra arguments appear as garbage.
- Any mismatch in the data type will produce unexpected results.
In this tutorial, we'll use a common example to illustrate the differences between these three methods. We'll take two numbers from the user and swap them, exploring each method: by value, by pointer, and by reference.
The default parameter passing mechanism in C++ is known as Pass by Value. This means the value of the actual parameter is copied to the formal parameter for the function's execution. As it operates on a copy of the actual parameter, the function's execution cannot alter the value of the actual parameter owned by the caller.
Let's illustrate this with a simple example. We have a function that requires two numbers from a user. When the user enters the numbers, this function attempts to swap them and returns the result.
#include<iostream>
using namespace std;
// Function to swap the numbers:
void swap(int i, int j)
{
int temp = j;
j = i;
i = temp;
}
main()
{
int a, b; // Variables to store the numbers
cout << "Enter the value of A: ";
cin >> a;
cout << "\nEnter the value of B: ";
cin >> b;
cout << "\nBefore swapping, the values are: A = " << a << ", B = " << b << endl;
swap(a, b); // Function call by value
cout << "After swapping, the values are: A = " << a << ", B = " << b << endl;
return 0;
}
Execute the provided code yourself and you'll notice that the values of A and B remain the same before and after swapping. This is because the value of the actual parameter is copied to the formal parameter for the function's execution, preventing any alteration to the caller's actual parameter value.
Passing by Pointer, also known as call by pointer, involves copying the address of an argument into the formal parameter. Inside the function, this address is used to access the actual argument used in the call. This means that changes made to the parameter affect the passed argument. To pass the value by pointer, argument pointers are passed to the functions just like any other value. Thus, the function parameters need to be declared as pointer types, which allows for the exchange of values of the two integer variables pointed to by its arguments.
Let's use the same example as before, but this time we'll pass the arguments by pointer.
// Swapping using Call-by-pointer
#include<iostream>
using namespace std;
// Function to swap the numbers
void swap(int *i, int *j)
{
int temp = *j;
*j = *i;
*i = temp;
}
main()
{
int a, b; // Variables to store the numbers
cout << "Enter The value of A: ";
cin >> a;
cout << "\nEnter The value of B: ";
cin >> b;
cout << "\nBefore swapping the value is: A = " << a << " B = " << b << endl;
swap(&a, &b); // Function call-by-pointer
cout << "After swapping the value is A = :" << a << " B = " << b << endl;
return 0;
}
Execute the provided code yourself and you'll notice that the values of A and B change indirectly after swapping. This time, we didn't pass only the values to the function but also passed the address of the variables to the function. The function swap() interestingly takes in the addresses of the local variables A and B. The pointer variables i and j now point to A and B. Therefore, the function has access to the memory that backs those two variables, so when it performs its switch, it writes into the memory of variables A and B.
While it's possible to achieve call-by-reference manually using pointer operators, this approach can be cumbersome. It forces you to perform all operations through pointers and remember to pass addresses (rather than values) of the arguments when calling the function. Fortunately, in C++, you can tell the compiler to automatically use call-by-reference for one or more parameters of a particular function using a reference parameter. When using a reference parameter, the address (not the value) of an argument is automatically passed to the function. Within the function, operations on the reference parameter are automatically dereferenced, eliminating the need for pointer operators.
Let's consider a simple example to understand how arguments can be passed by reference to a function.
// Swapping using Call-by-Reference
#include<iostream>
using namespace std;
// Function to swap the numbers
void swap(int &i, int &j)
{
int temp = j;
j = i;
i = temp;
}
main()
{
int a, b; // Variables to store the numbers
cout << "Enter The value of A: ";
cin >> a;
cout << "\nEnter The value of B: ";
cin >> b;
cout << "\nBefore swapping the value is: A = " << a << " B = " << b << endl;
swap(a, b); // Function call-by-reference
cout << "After swapping the value is A = :" << a << " B = " << b << endl;
return 0;
}
In the above example, the values of A and B change indirectly after swapping, similar to pointers. As apparent from the name "by reference," we are not passing the value itself but some form of reference or address. Thus, by passing the address of the variable to the called function, we convey to the function that the number we should change is located inside this passed memory location. When the calling function regains control after calling the called function, it receives the changed value back in the same memory location.
It's important not to confuse pointers with references. Pointers are variables that store the address of another variable, while references are variables that refer to another variable. References are commonly used in function parameters and return types to define clear interfaces, while pointers are typically used to implement algorithms and data structures.
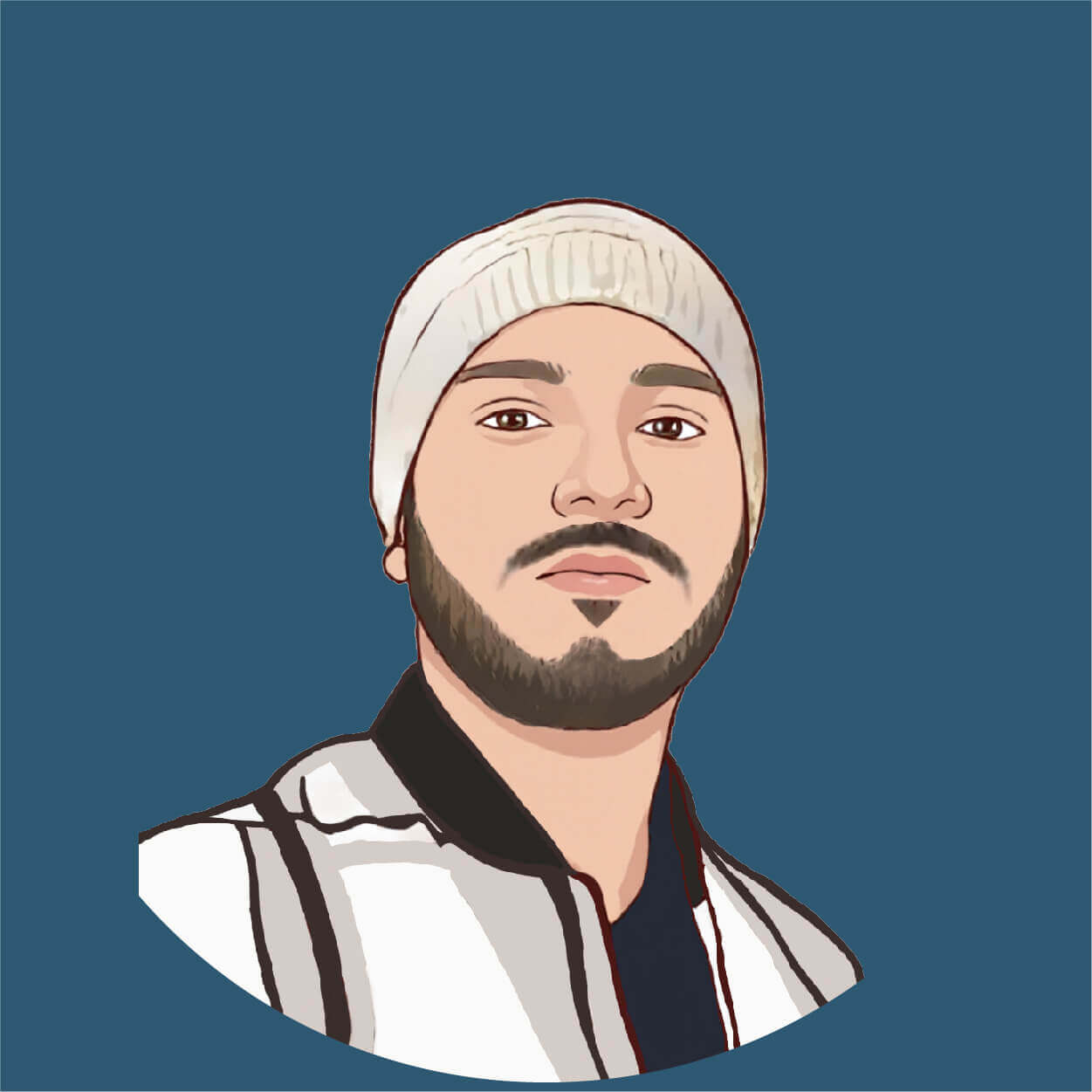
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.