PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
WHAT IS INHERITANCE?
Inheritance is a fundamental concept in Object-Oriented Programming (OOP) that greatly enhances the capability of C++ programming. Mastery of this concept is crucial for effective C++ programming, marking a significant milestone in your journey to becoming an Object-Oriented Programmer. Once you grasp this concept, you'll solidify your understanding of how objects interact. To comprehend inheritance, let's delve into a real-life analogy in our tutorial.
Inheritance draws parallels to why we resemble our parents. Just as individuals inherit physical traits like the shape of their nose or the color of their eyes from their parents, objects in programming inherit characteristics from their parent classes. This transfer of characteristics is at the core of inheritance.
Much like how a building is constructed from bricks, our bodies are composed of cells, guided by DNA. DNA acts as the boss, dictating the instructions for building a human body. As we inherit DNA from both our parents, we receive directives on constructing our bodies from both sides, resulting in a blend of traits from each parent.
While people exhibit unique features, there's a fundamental similarity among us because of our shared ancestry and the inheritance of characteristics. This concept underscores the essence of inheritance. Now that we've explored this analogy, let's transition to the realm of programming.
In C++, inheritance refers to the process of acquiring data members and methods from one class to another. It stands as a cornerstone of object-oriented programming, enabling the extension of existing classes' attributes to new classes. Essentially, inheritance allows for the creation of classes that build upon the foundation laid by others, thus saving development time by leveraging existing structures.
The class from which properties are inherited is termed the Parent, Base, or Super class, while the class inheriting those properties is referred to as the Child, Derived, or Sub class.
Throughout our tutorials, we'll refer to them as Base and Derived classes.
The basic syntax to implement inheritance in C++ is as follows:
class Derived_Class: Access-Specifier Base_class
{
//body of the Derived class.
}
In the above syntax:
Denotes the keyword to declare a class.
Represents the class inheriting properties from another class.
Defines the scope of class members, including variables and methods. In C++, access specifiers can be public, private, or protected.
Refers to the class whose properties are inherited.
Let's elucidate this with a simple example. We'll create a base class named TwoD_Shape
, storing the dimensions of two-dimensional objects, and a derived class named Triangle
, inheriting from TwoD_Shape
. Pay attention to the class declarations in this example.
#include<iostream>
#include<cstring>
using namespace std;
class TwoD_Shape //Base Class:
{
public: //Public Members:
double width;
double height;
//Function to display dimensions:
void showDim()
{
cout << "Width and height are " << width << " and " << height << endl;
}
};
/*Base Class (i.e., TwoD_Shape) is Inherited by
derived class (i.e., Triangle)*/
class Triangle: public TwoD_Shape
{
public:
char style[20];
double area()
{
//Using public members of base class
return width * height / 2;
}
void showStyle()
{
cout << "Triangle is " << style << endl;
}
};
int main()
{
//Create two objects of type Triangle.
Triangle t1, t2;
t1.width = 4.0;
t1.height = 4.0;
strcpy(t1.style, "isosceles");
t2.width = 8.0;
t2.height = 12.0;
strcpy(t2.style, "right");
cout << "Info for t1: " << endl;
t1.showStyle();
t1.showDim();
cout << "Area is " << t1.area() << endl;
cout << "\nInfo for t2: " << endl;
t2.showStyle();
t2.showDim();
cout << "Area is " << t2.area() << endl;
return 0;
}
In this example, we establish TwoD_Shape
as the base class and Triangle
as the derived class inheriting properties from TwoD_Shape
. The declaration class Triangle: Public TwoD_Shape
signifies this inheritance. Since Triangle
incorporates all members of its base class, TwoD_Shape
, it can access width and height within area()
. Similarly, in main()
, objects t1
and t2
can directly access width and height as though they were part of Triangle
.
Despite serving as the base for Triangle, TwoD_Shape remains an independent class, capable of standalone use.
A derived class can access all non-private members of its base class. Therefore, base-class members that shouldn't be accessible to derived class member functions should be declared private in the base class. The table below summarizes the accessibility based on different contexts:
Access | Public | Protected | Private |
---|---|---|---|
Same Class | |||
Derived Class | |||
Outside Class |
A derived class inherits all public methods of the base class, except for:
- Constructors, destructors, and copy constructors of the base class.
- Overloaded operators of the base class.
- Friend functions of the base class.
Applications developed using inheritance offer several advantages:
- Reduced development time.
- Lower memory footprint.
- Improved execution speed.
- Enhanced application performance.
- Decreased code repetition, leading to consistent results and lower storage costs.
Explore different types of inheritance for further understanding.
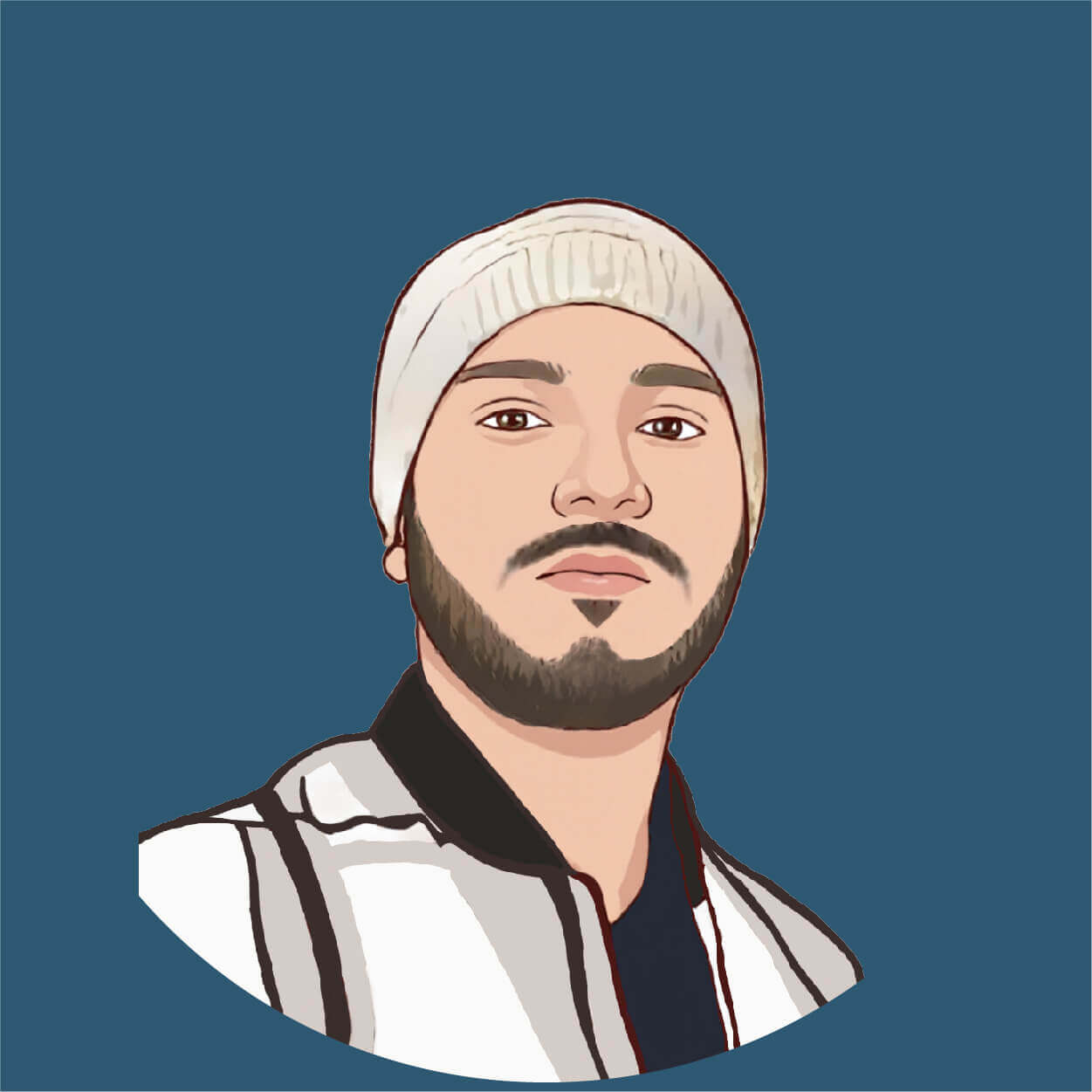
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.