PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
LOOP CONTROL STATEMENTS:
Loop control statements are used in C++ to change the normal sequence of execution. These statements offer a way to alter the flow of a program, providing more flexibility and control over how code is executed. C++ supports several loop control statements, including:
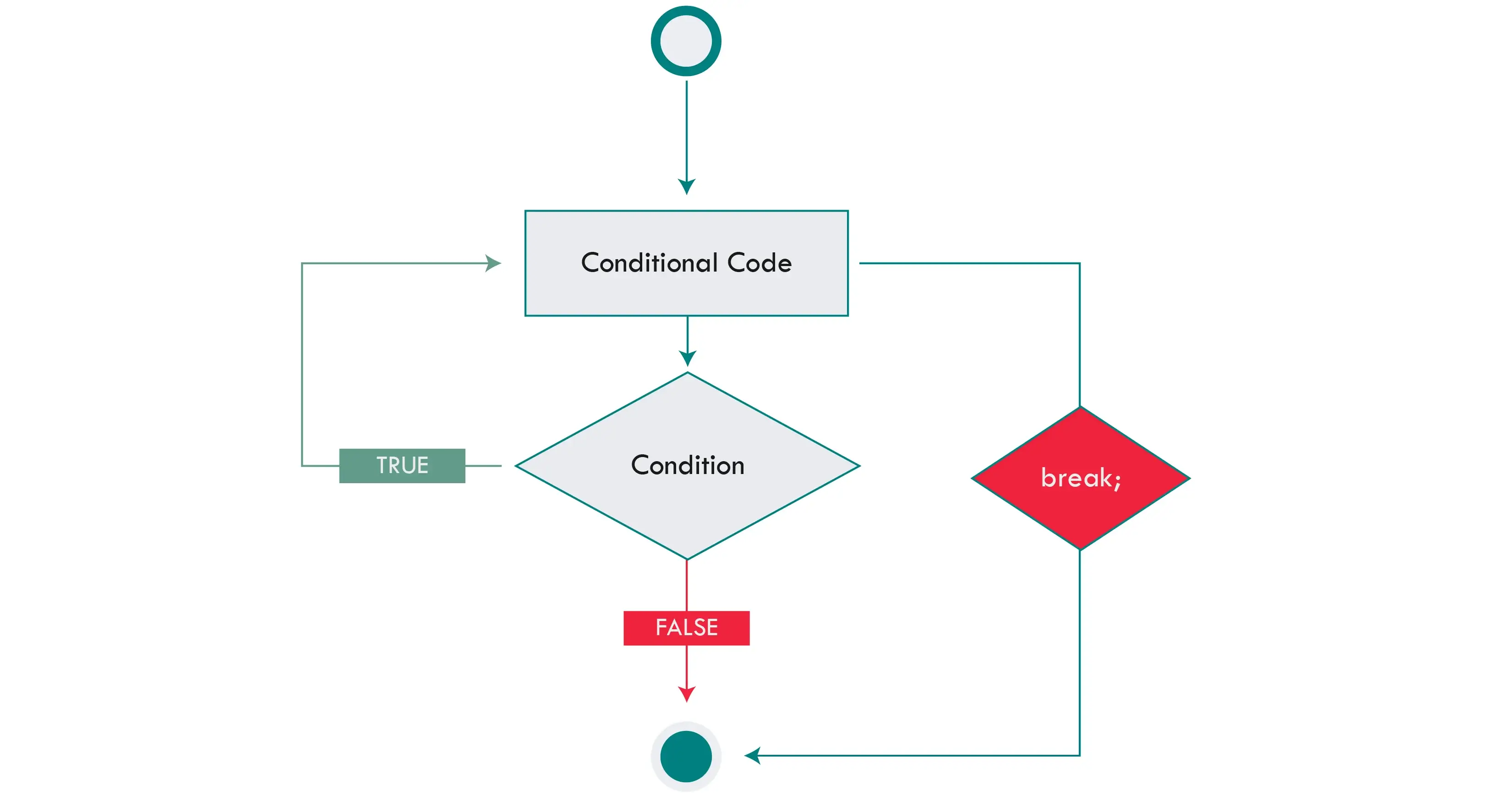
The break statement in C++ allows us to prematurely exit from a loop or switch statement.When encountered, it terminates the current loop iteration or switch case and transfers control to the next statement outside of the loop or switch block.
In a switch statement, without a break statement, the flow of control continues to the next case, executing all subsequent cases until a break statement is encountered. By using break, we can limit the execution to only the desired case.
When used within a loop, the break statement immediately exits the loop, allowing the program to continue executing the next statement after the loop.
break;
Let's illustrate the functionality of the Break statemen
in a C++ program with an example. In this demonstration, we aim to print the first 10 integers (from 0 to 10) using a For Loop.However, we'll employ the 'Break statement' to terminate the loop prematurely when the integer value reaches 5. This can be seen in the following example.
#include <iostream> using namespace std; main() { //Loop to print the first 10 integers. for(int i = 0; i < 10; i++) { cout << " Value of i is: " << i << endl; if(i==5) // If the loop reaches 5. break; //Terminate the loop. } return 0; }
It's generally advisable to minimize the use of break statements in loops to maintain code readability and avoid logical errors. However, in switch statements, break statements are necessary after each case to prevent unintended fall-through behavior.
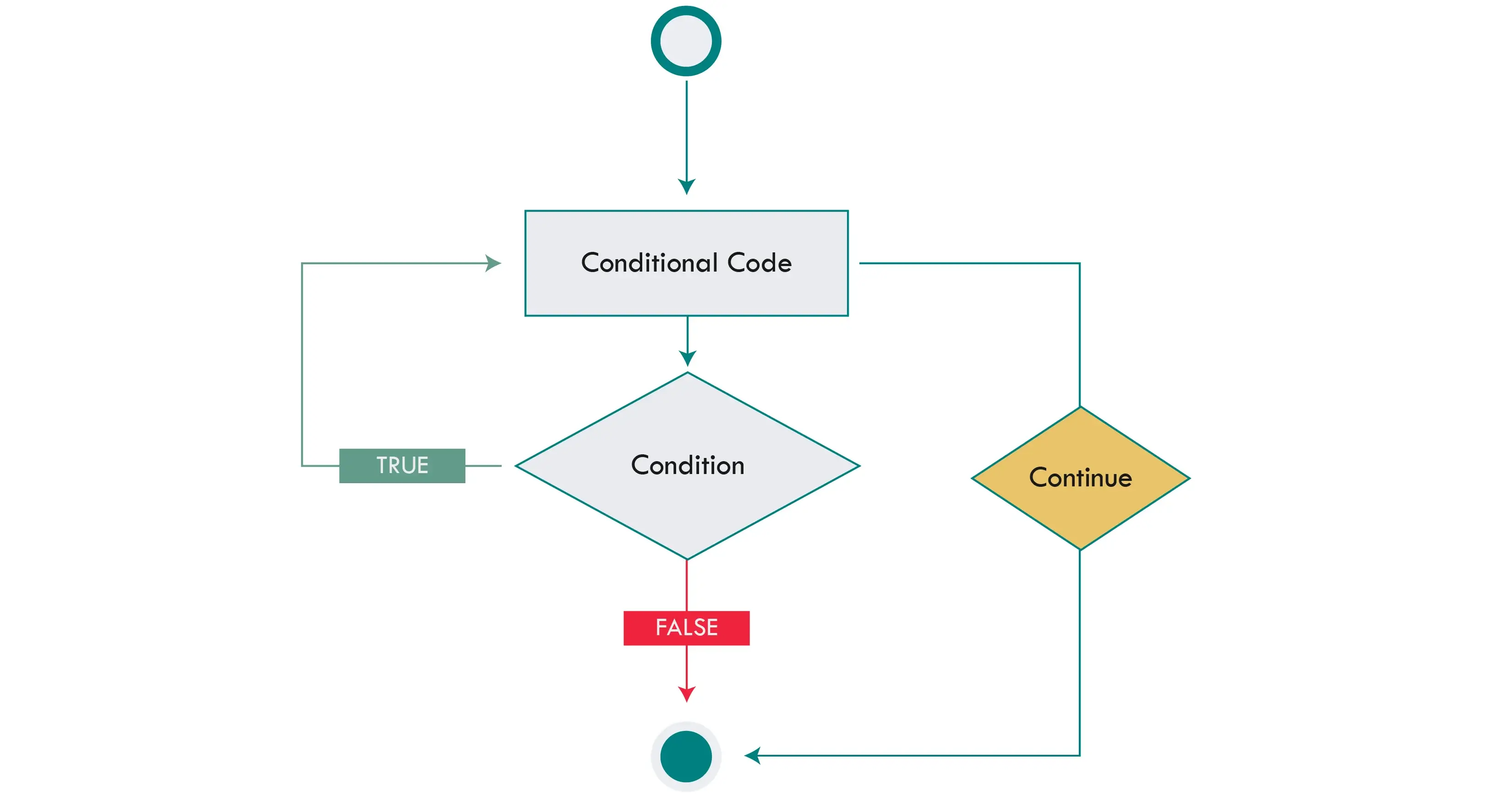
Similar to the break statement, the continue statement alters the flow of a loop in C++. However, instead of terminating the loop, it skips the remaining code in the current iteration and jumps to the next iteration, re-evaluating the loop's condition.
In a For Loop, continue causes the conditional test and increment portions of the loop to execute. In while and do-while loops , control passes directly to the conditional test.
continue;
Let's explore a straightforward example to understand the functionality of the continue statement in a C++ program. In this example, we'll aim to print the first 10 integers (0 to 10) using a do-while loop. However, we'll utilize the continue
statement to skip the integer "4," as demonstrated in the following example:
#include <iostream> using namespace std; main() { int a = 0; do { if (a == 4) // When the loop reaches the integer 4. { a = a + 1; // Skip that iteration (Skip 4) continue; // Then continue with the loop. } cout << "value of a: " << a << endl; a = a + 1; } while (a <= 10); return 0; }
While continue statements offer a way to skip specific iterations within a loop, excessive use may hinder code readability. In some cases, restructuring the loop logic or using conditional statements may be preferable.
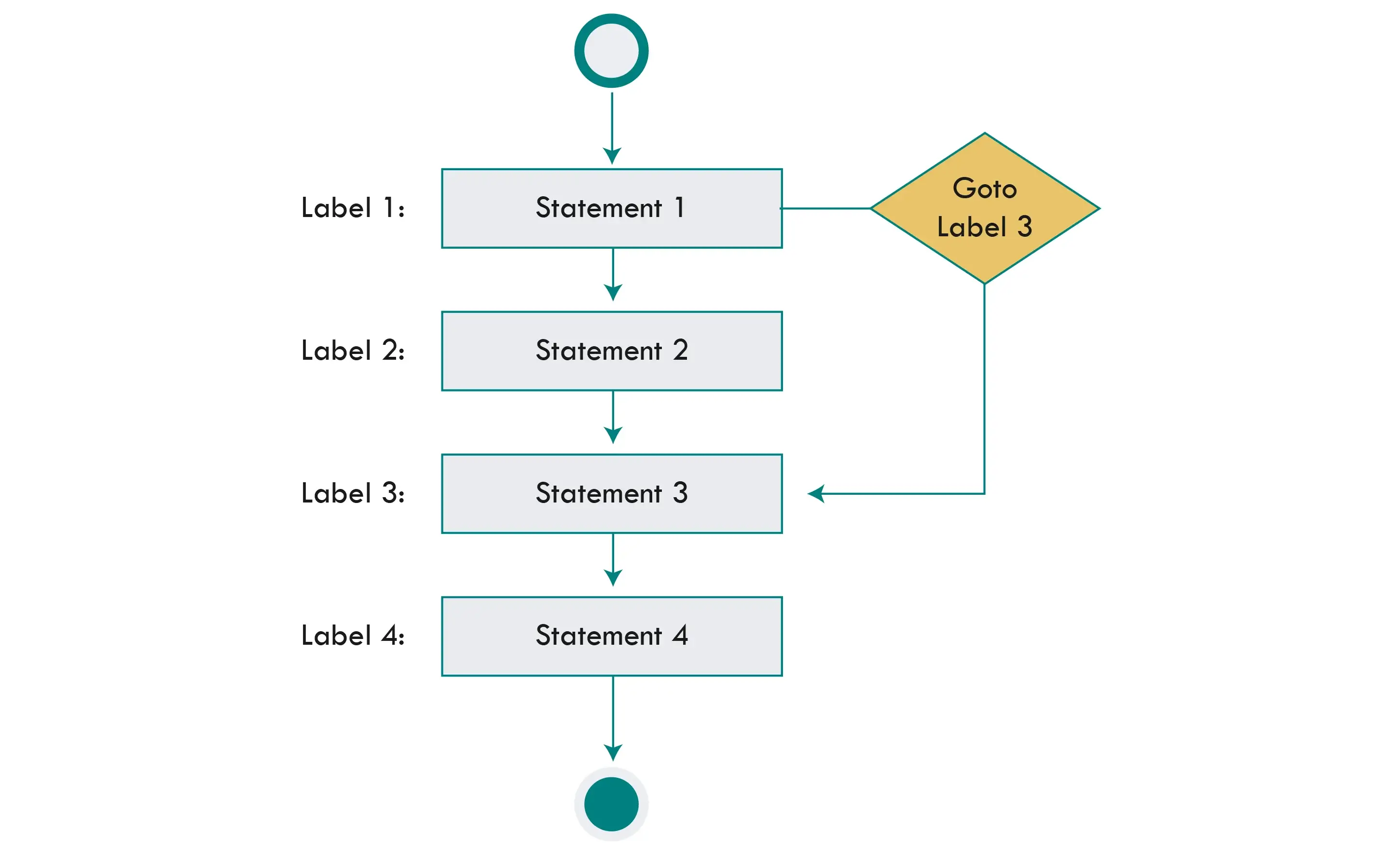
The goto statement in C++ provides an unconditional jump to a labeled statement within the same function. It allows programmers to transfer control to any part of the code, regardless of the normal flow of execution.
goto label; ... ... ... label: Statement(s);
Let's explore a basic example to understand the functionality of the goto statement in C++. In this example, we'll attempt to print the first 10 integers (0 to 10) using a do-while loop. However, we'll use the goto
statement to skip the integer "4" and proceed to the next integer, "5", as demonstrated below:
#include <iostream> using namespace std; main() { int a = 0; LOOP: // Label for goto statement do { if (a == 4) // When the loop reaches the integer 4. { a = a + 1; // skip 4. goto LOOP; // And Jump to label "Loop". } cout << "value of a: " << a << endl; a = a + 1; } while (a <= 10); return 0; }
The use of goto statements is discouraged in modern programming practices due to their potential to create complex and difficult-to-follow code paths, often referred to as "spaghetti code." Whenever possible, alternative control structures such as loops and conditional statements should be used to maintain code clarity and ease of maintenance.
Copy and run the provided examples yourself and experiment with them to understand how these statements work. Remember, practice is key to becoming a successful programmer. Enjoy your learning!
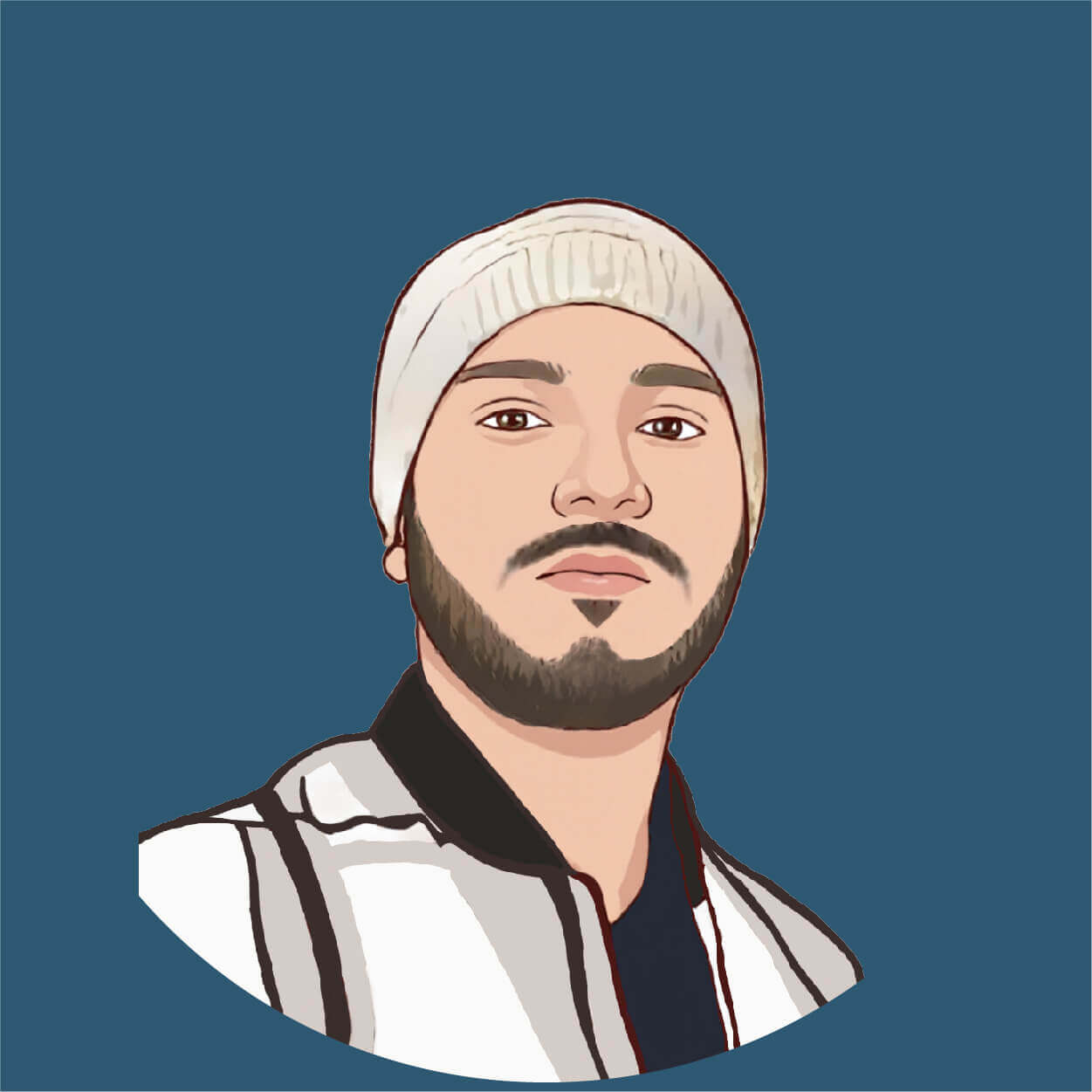
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.