PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
DATA TYPES IN C++:
When writing programs in any programming language, the use of variables is crucial for storing information. Variablesare essentially reserved memory locations that hold values. Creating a variable involves reserving a specific space in memory.
We will learn more about C++ variables in our Variable Tutorial.
We may need to store information of different data types such as characters, integers, floating point numbers, etc. Depending on the data type of a variable, the operating system allocates memory and determines what can be stored in that memory.
In computer programming, information is stored in computer memory using different data types. We need to know what we are storing in computer memory, whether it's a digit, a character, a small number, or a decimal number. Computer memory is organized in bytes, and for variables with varying information, a data type is associated. A byte is the smallest amount of memory in a computer that can store a small amount of data and can be easily managed. Every variable is declared with two entities: its type and its name. C++ provides several data types, including char
, int
, float
, double
, bool
, etc.
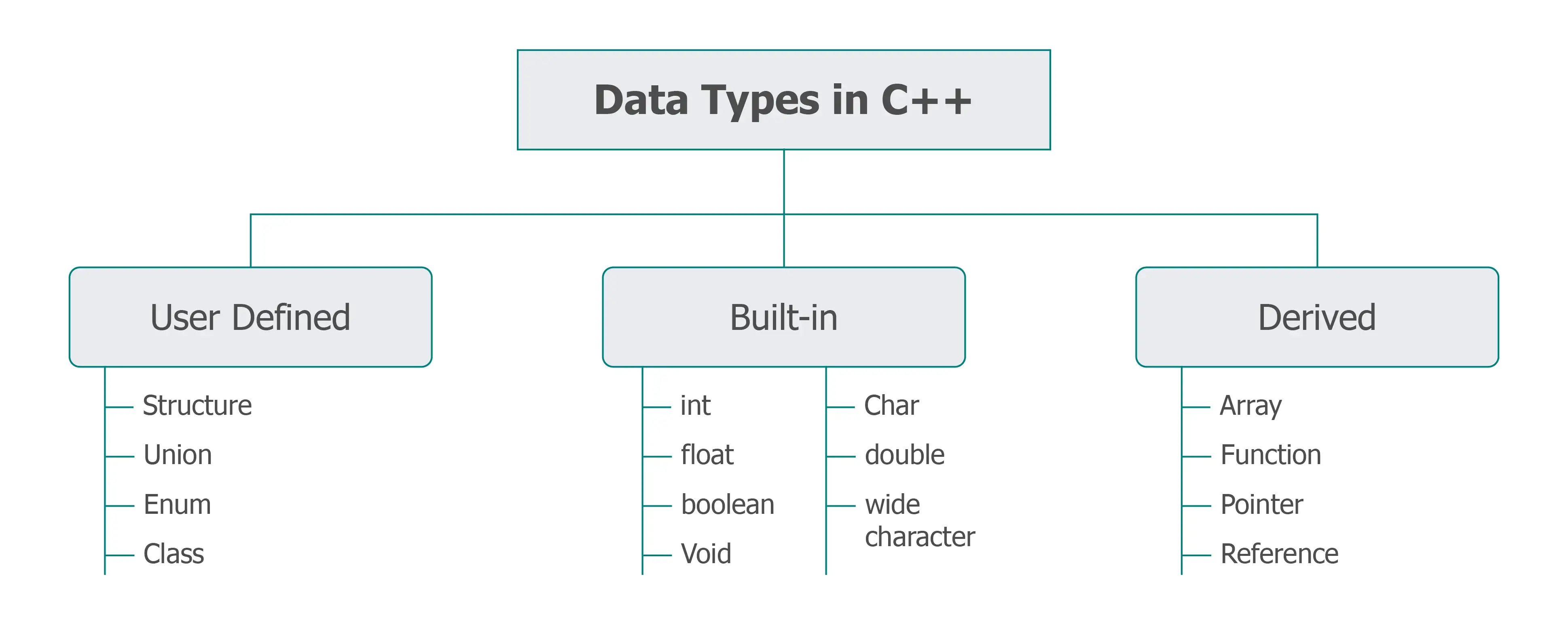
C++ offers various data types, each represented differently in the computer's memory. The data types provided by C++ include:
- Built-in data types.
- Derived data types.
- User-defined data types.
DATA TYPE (Keywords) | DESCRIPTION | SIZE | TYPICAL RANGE |
---|---|---|---|
int | Integer. | 4 bytes | -2147483648 to 2147483647 |
Signed int | Signed integer. Values may be Negative, positive, or Zero. | 4 bytes | -2147483648 to 2147483647 |
Unsigned int | Unsigned integer. value are always positive or zero. Never Negative value. | 4 bytes | 0 to 4294967295 |
Short | short Integer. | 2 bytes | -32768 to 32767 |
Signed short | Signed short integer. values may be positive, Negative, or zero. | 2 bytes | -32768 to 32767 |
Unsigned short | Unsigned short integer. Values are always positive or zero. Never Negative value. | 2 bytes | 0 to 65535 |
Long | Long integer. | 4 bytes. | -2147483648 to 2147483647 |
Signed long | Signed long integer. values may be Negative, Positive, or zero. | 4 bytes | -2147483648 to 2147483647 |
Unsigned long | Unsigned long integer. values are always positive or zero. Never Negative value. | 4 bytes | 0 to 4,294,967,295 |
DATA TYPE (Keywords) | DESCRIPTION | SIZE | TYPICAL RANGE |
---|---|---|---|
char | Any single character. it may include a letter, a digit, a punctuation mark, or a space. | 1 byte | -128 to 127 or 0 to 255 |
Signed char | Single character. | 1 byte | -128 to 127 |
unsigned char | Unsigned character | 1 byte | 0 to 255 |
wchar-t | Wide character | 2 or 4 bytes | 1 wide character |
DATA TYPE (Keywords) | DESCRIPTION | SIZE | TYPICAL RANGE |
---|---|---|---|
float | Floating point number. there is no fixed number of digits before or after the decimal point. | 4 bytes | +/- 3.4e +/- 38 (~7 Digits) |
Double | Double precision floating point number. More accurate compared to float. | 8 bytes | +/- 1.7e +/- 308 (~15 Digits) |
long double | Long double precision floating point number. | 8 bytes | +/- 1.7e +/- 308 (~15 Digits) |
DATA TYPE (Keywords) | DESCRIPTION | SIZE | TYPICAL RANGE |
---|---|---|---|
bool | Boolean value. it can only take one of two values: true or False. | 1 byte | true or false |
Note that the sizes of the above-mentioned variables may vary on different computers due to the compiler and computer structure. The following example can help you determine the correct size of various data types. Copy and paste the following code into your compiler to learn about your data sizes.
#include<iostream> using namespace std; main() { cout << "Integer data type"<< endl; cout << "size of int is "<< sizeof(int)<<endl; cout << "size of signed int is "<< sizeof(signed int) << endl; cout << "size of unsigned int is "<< sizeof(unsigned int) << endl; cout << "size of short is "<< sizeof(short) << endl; cout << "size of signed short is "<< sizeof(signed short) << endl; cout << "size of unsigned short is "<< sizeof(unsigned short) << endl; cout << "size of long is "<< sizeof(long) << endl; cout << "size of signed long is "<< sizeof(signed long) << endl; cout << "size of unsigned long is "<< sizeof(unsigned long) << endl; cout << "\n\n character data type" << endl; cout << "size of char is "<< sizeof(char) << endl; cout << "size of signed char is "<< sizeof(signed char) << endl; cout << "size of unsigned char is "<< sizeof(unsigned char) << endl; cout << "size of wchar_t is "<< sizeof(wchar_t) << endl; cout << "\n\n Floating point data type:" << endl; cout << "size of float is "<< sizeof(float) << endl; cout << "size of Double is "<< sizeof(double) << endl; cout << "size of long double is "<< sizeof(long double) << endl; return 0; }
In addition to the standard data types, C++ introduces the String data type. Although not inherently part of the C++ programming language, it is defined in the < string >
header file. To use this data type, include the "string" header file. A variable of type String can contain a string like "Hello World" or "InfoBrother." The length of the string can change during the program's execution. String is essentially an array of characters, represented in C++ as "C-Style Character String" and using the String Class.
The void data type is used to specify an empty parameter list for a function or as a return type for a function. When void is used to specify an empty parameter list, it means that the function does not take any arguments. When used as a return type, it means that the function does not return a value. Void does not allocate any memory and therefore cannot store anything. As a result, void cannot be used to declare simple variables, but it can be used to declare generic pointers.
Derived data types are data types that are derived from the built-in data types. Array, Function, References, and Pointers are examples of derived data types provided by C++.
Array:An arrayis a set of elements of the same data type that are referred to by the same name. All the elements in an array are stored in contiguous memory locations, and each element is accessed by a unique address.
Function:
A functionis a self-contained program segment that performs a specific well-defined task. In C++, every program contains one or more functions that can be invoked from other parts of the program if needed.
Reference:
A referenceis an alternative name for a variable. It is an alias for a variable in a program. A variable and its reference can be used interchangeably as they refer to the same memory location. Changes made to either one are reflected in the other.
Pointer:
A pointeris a variable that can store the memory address of another variable. Pointers allow for dynamic memory usage. With the help of pointers, memory can be allocated or deallocated to variables at runtime, making a program more efficient.
User-defined data types in C++ allow the storage of multiple values of the same type, different types, or both. C++ provides various user-defined data types, such as Structures, Unions, Enumerations, and Classes.
Structure & Union:Structures and Unionsare essential features in C language, providing a way to group similar or dissimilar data types under a single name. C++ extends the concept of structures and unions with additional features to support object-oriented programming.
Class:
C++ introduces a new user-defined data type called a Class, forming the basis of object-oriented programming. A class serves as a template that defines the data functions included in an object
of the class. Classes are declared using the keyword class
and once declared, their objects can be easily created.
Reference:
A referenceis an alternative name for a variable. It is an alias for a variable in a program. A variable and its reference can be used interchangeably as they refer to the same memory location. Changes made to either one are reflected in the other.
Enumeration:
Enumeration is a user-defined data type with a finite set of enumeration constants. The enum
keyword is used to create an enumerated data type.
Pro-Tip:
Understanding and utilizing these data types in C++ is fundamental to effective programming and ensures efficient memory usage.
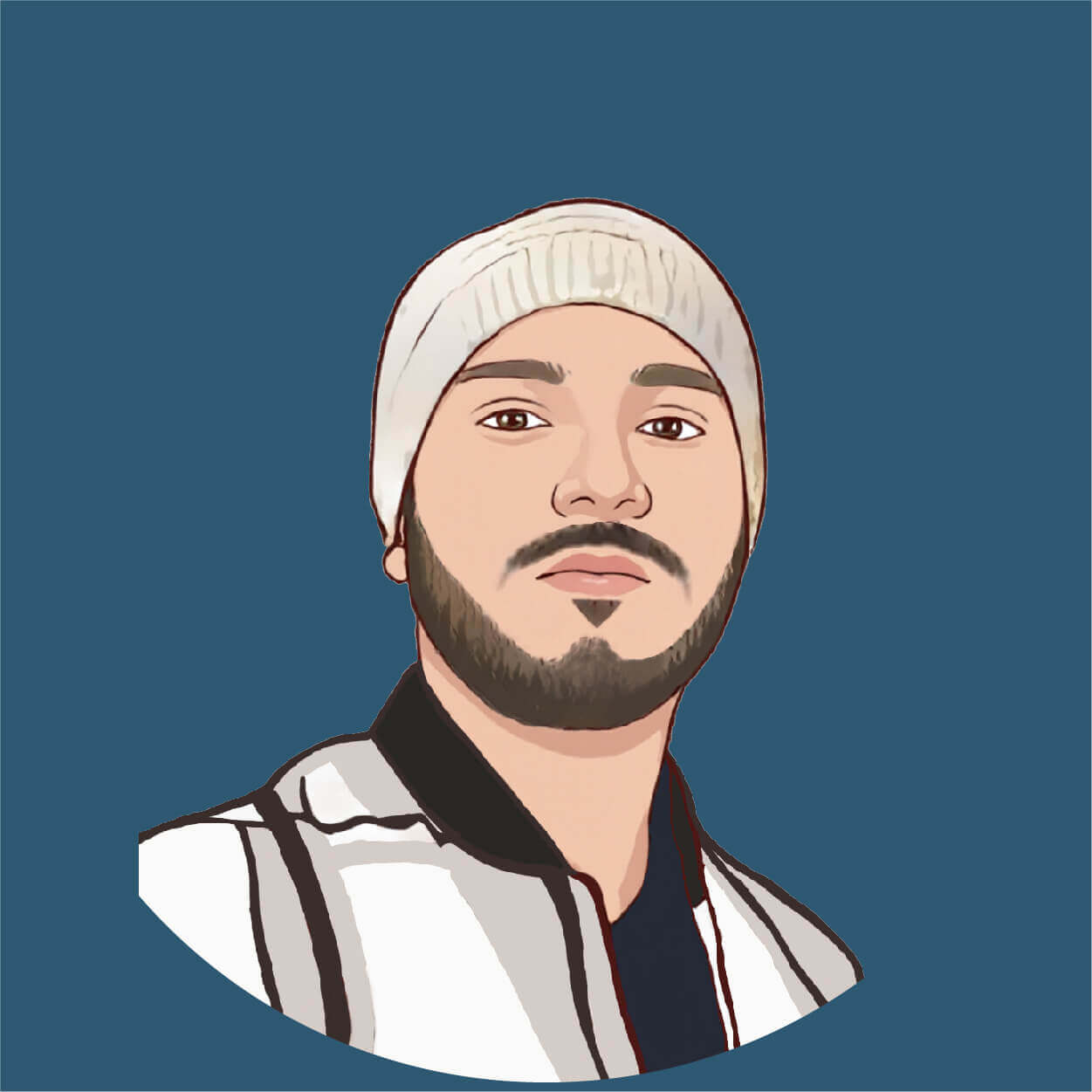
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.