PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Dynamic Memory Allocation with malloc():
In our previous tutorial, New Operator,we discussed dynamic memory allocation using the new operator. In this tutorial, we'll explore dynamic memory allocation using the malloc()
function provided by the old C library <stdlib.h>
.
Similar to the new
operator, the malloc()
function dynamically allocates memory and returns a pointer to the first byte of the allocated memory block from the heap. If there isn't enough space to allocate, this function returns a "NULL" pointer. The basic syntax is as follows:
void * malloc (size_t size);
This function returns a void pointer to the allocated memory. If there's insufficient memory available, it returns NULL. malloc()
requires an argument, which is the size in bytes of the memory area we want to reserve.
If we want the pointer to return a type other than void, we can use a type cast operator on the return value. The syntax is as follows:
type *ptr; // Pointer to Data_type
ptr = (type *)malloc(size);
In the above example, we declare a pointer of the specified type, then malloc()
is used to allocate memory based on the size in bytes using sizeof()operator. Finally, our syntax would be:
int *ptr; // Pointer to int
ptr = (int*)malloc(sizeof(int));
The use of sizeof()operator may resemble a function call, but it's actually an operator, and it doesn't work at compile time.
Let's look at a simple example to understand how this function dynamically allocates memory.
/* Example: Using malloc() Function to Allocate Memory */
#include<iostream>
#include<stdlib.h> // Required for malloc() function
using namespace std;
int main()
{
int *ptr, *ptr2; // ptr is used to allocate memory
// ptr2 will be used to display the result
int num, sum = 0; // num is the number of elements
cout << "Enter the total number of elements to input: ";
cin >> num;
ptr = (int*)malloc(num * sizeof(int)); // Memory allocation
ptr2 = ptr;
cout << "Please enter your " << num << " elements: " << endl;
for (int i = 1; i <= num; i++) // Loop to store data
{
cout << "Element # " << i << ": ";
cin >> *ptr;
sum += *ptr; // Add all numbers
ptr++;
}
cout << "\n\nYou entered: " << endl;
for (int i = 1; i <= num; i++) // Loop to display the result
{
cout << "Element # " << i << ": ";
cout << *ptr2 << endl;
*ptr2++;
}
cout << "The sum of these numbers is: " << sum;
return 0;
}
In the example above, we use the malloc()
function to allocate dynamic memory. This function allocates sufficient memory from the heap, stores some elements, and returns their sum. If malloc()
fails to allocate the requested memory, it returns a NULL pointer, which points to nowhere. Using a NULL pointer can cause the program to crash. Therefore, it's important to include an error checker to ensure malloc()
successfully allocates the memory. After adding an error checker, our program will look like this:
/* Example: malloc() Function with Error Checker */
#include<iostream>
#include<stdlib.h> // Required for malloc() function
using namespace std;
int main()
{
int *ptr, *ptr2; // ptr is used to allocate memory
// ptr2 will be used to display the result
int num, sum = 0; // num is the number of elements
cout << "Enter the total number of elements to input: ";
cin >> num;
ptr = (int*)malloc(num * sizeof(int)); // Memory allocation
ptr2 = ptr;
if (ptr == NULL) { // Error check
cout << "An Error Occurred While Memory Allocation." << endl;
exit(0);
}
else {
cout << "Please enter your " << num << " elements: " << endl;
for (int i = 1; i <= num; i++) // Loop to store data
{
cout << "Element # " << i << ": ";
cin >> *ptr;
sum += *ptr; // Add all numbers
ptr++;
}
cout << "\n\nYou entered: " << endl;
for (int i = 1; i <= num; i++) // Loop to display the result
{
cout << "Element # " << i << ": ";
cout << *ptr2 << endl;
*ptr2++;
}
cout << "The sum of these numbers is: " << sum;
}
return 0;
}
We've provided a simple example demonstrating dynamic memory allocation using malloc()
. This function allocates memory from the heap, stores some elements, and returns their sum. If malloc()
is unable to allocate the requested memory, it returns a NULL pointer, indicating an allocation failure. Therefore, it's advisable to include an error checker to handle such scenarios, ensuring the program's stability.
Remember to release dynamically allocated memory using free()
function after it's no longer needed. Unlike new pperator,, which constructs objects, malloc()
simply allocates memory, making new a preferred choice in C++ due to its ability to initialize objects.
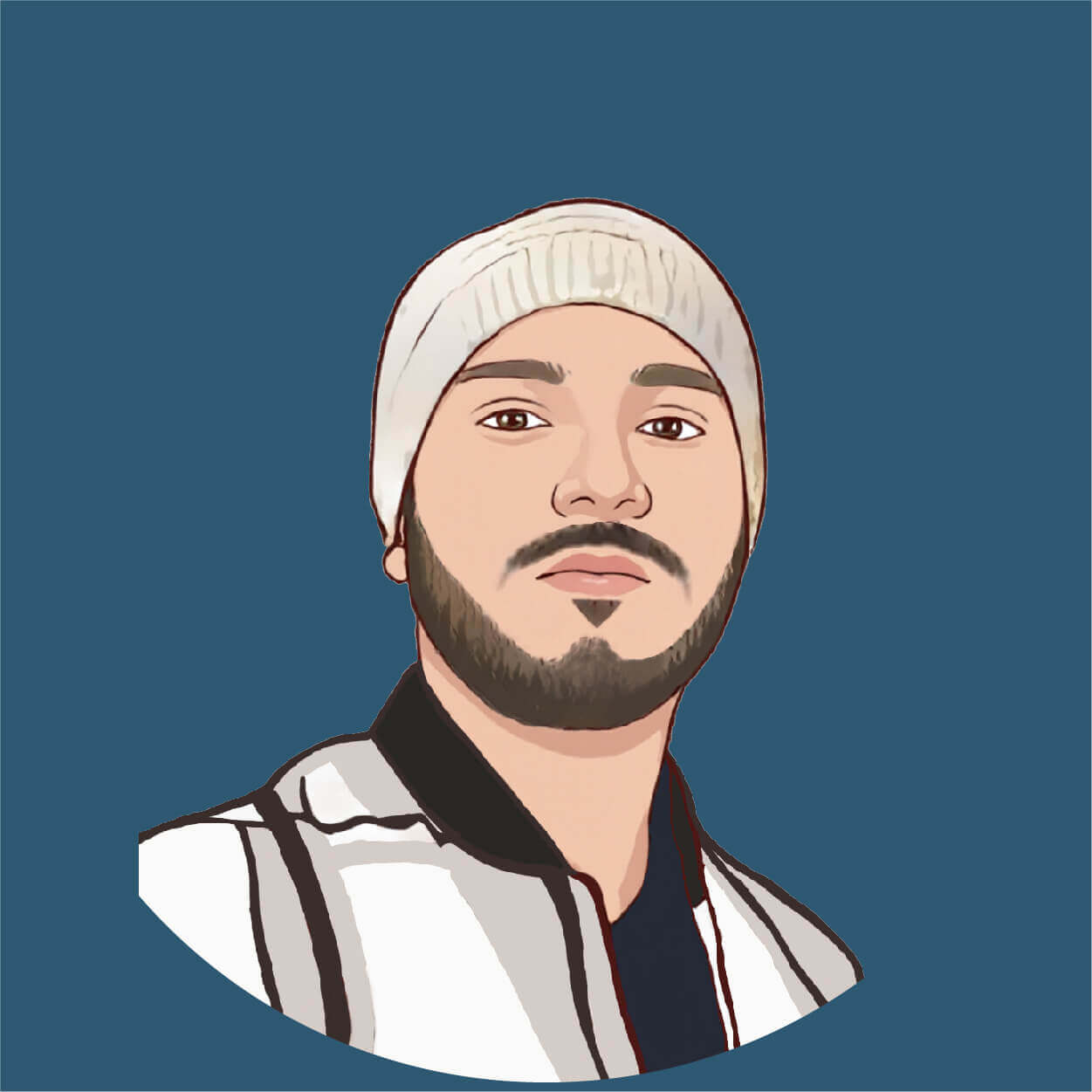
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.