PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
WHAT IS CONSTANT?
Constants in programming are fixed values that do not change during the execution of a program. They provide a way to store and reference unchanging data. In C++, two common methods for defining constants are using the #define
preprocessor directive and the const
keyword.
A constant, similar to a variable, represents a memory location that stores a value. However, unlike variables, constants retain their initial value throughout the program's execution. An example of this permanence can be seen in fundamental scientific constants such as the speed of light or Avogadro's number. In C++, we can declare constants using the const
keyword, specifying the data type and initializing it immediately.
const double PI = 3.14159;
Once initialized, a constant behaves like a variable in most aspects, except that it cannot be reassigned a new value. Attempting to do so, as illustrated below, results in a compilation error:
PI = 3.41; // Not allowed in C++
This statement would result in a compilation error.
Note:
Constants must be initialized at their point of declaration, and it is forbidden to assign a constant outside of its declaration statement.
There are two straightforward methods in C++ to define constants:
The #define
preprocessor directive is a method inherited from C, although its use is less frequent in modern C++ due to the availability of the const
keyword. Still, it's valuable for compatibility when transitioning from C to C++. The syntax for using #define is as follows:
#define VARIABLE_NAME value
In this syntax, VARIABLE_NAME is a single variable name (usually written in uppercase), and value is the constant's content. The #define directive replaces all occurrences of VARIABLE_NAME in the program with its assigned value.
#define PI 3.14159
Note:
It is important to note that #define is a preprocessor directive and not a C++ command. No semicolon should be added at the end of its expression. Adding a semicolon at the end of its expression will result in an error.
#include <iostream> using namespace std; #define GREETING "Welcome to InfoBrother" #define NAME "Omar" #define AGE 22 #define NEWLINE '\n' // \n is for newline. int main() { cout << GREETING << NEWLINE; cout << "This is " << NAME; cout << " And i am " << AGE << " Year Old:"; return 0; }
The const
keyword in C++ allows us to declare constants, providing both the data type and a semicolon at the end of the statement. While functionally equivalent to #define
, it aligns more with the modern C++ approach and is considered a statement rather than a preprocessor directive.
const double PI = 3.14159;
Note:
Remember to include a semicolon at the end of a const statement, as it is a part of the C++ syntax. Failure to do so will result in a compilation error.
#include <iostream> using namespace std; const string GREETING = "Welcome to InfoBrother"; const string NAME = "Omar"; const int AGE = 22; const char NEWLINE ='\n'; // \n is for newline. int main() { cout << GREETING << NEWLINE; cout << "This is " << NAME; cout << " And i am " << AGE << " Year Old:"; return 0; }
Understanding constants and how to define them in C++ is essential for writing robust and maintainable code. Whether using the #define preprocessor or the const keyword, choosing the appropriate method depends on factors such as code readability, compatibility, and adherence to modern C++ standards.
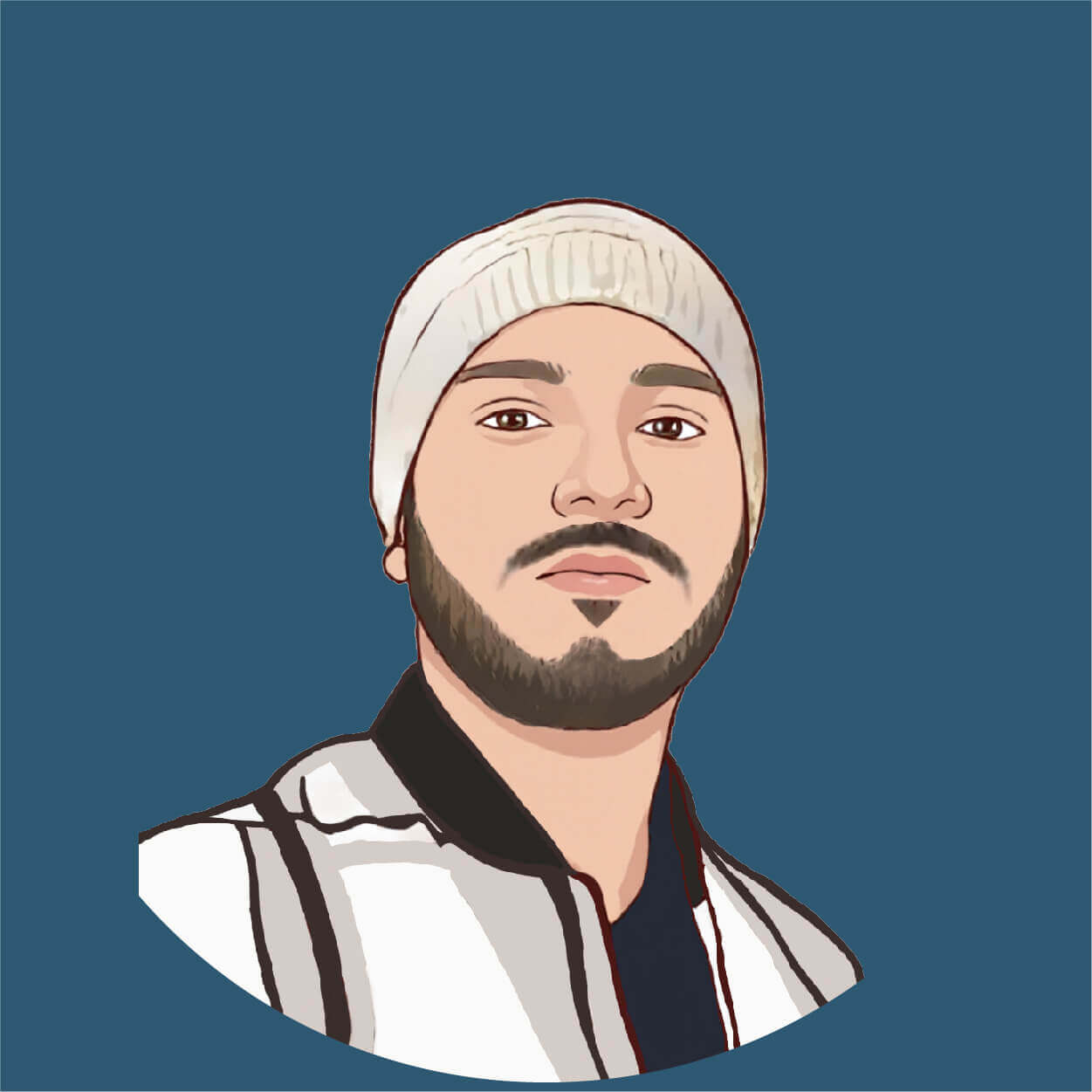
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.