PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
STRUCTURE VS UNION:
A structure struct
is utilized to define a data type comprising several fields, where each field occupies a distinct storage location.
struct Disk
{
int S-width;
int S-height;
};
On the other hand, a union is akin to a structure but designates a single location that can be labeled with multiple field names.
union Disk
{
int U-width;
int U-height;
};
In a union, such as the one illustrated above, U-Width and U-Height share the same space. Think of a structure as a box partitioned into compartments, each labeled with its own name. Conversely, a union is an undivided box with various labels affixed to the single compartment inside. In a structure, fields operate independently, meaning altering one field doesn't affect others. However, in a union, all fields occupy the same space, permitting only one to be active at any given time. Consequently, assigning a value to one field in a union overrides the value stored in any other field.
The concept behind a union in C/C++ revolves around accessing a memory location through various means. If we've utilized int
and char
interchangeably in our programs, storing a char within an int for instance, can we access a memory location as both an int and a char? For such scenarios, the construct Union is employed. The syntax of a Union is as follows:.
union Union_Tag
{
Data-Type1 Element1;
Data-Type2 Element2;
Data-Type3 Element3;
.
.
Data-TypeN ElementN;
} Union_Object;
It's crucial to include a semicolon ;
after the closing curly brace in Union definitions, as the program will fail to compile without it. Despite being easily overlooked, this semicolon is essential because in other contexts within C++ programs, closing curly braces are seldom followed by a semicolon.
Where Union_Tag serves as a label for the structure type model and the optional parameter Union_Object is a valid identifier for instantiating structure objects. Inside the curly brackets {}
types and their sub-identifiers correspond to the elements composing the structure.
We must name Union_Tag ourselves, adhering to the same naming conventions for variables. By naming a Union_tag, such as intOrChar, we inform C++ that the tag named "intOrChar" resembles a character array, comprising variables with different data types.
Pro-Tip:
No memory is reserved for Union tags. A union is a user-defined data type. C++ only allocates memory for the integer data type when we declare an integer variable, similarly to how it doesn't reserve memory for a structure until we declare a structure variable.
If the Union definition includes the parameter intOrChar, that parameter becomes a valid type name equivalent to the structure, as shown in the following example:
union intOrChar
{
int i;
char c ;
};
The syntax mirrors that of a structure. In structures, different data members each have their memory space. However, in a union, the memory location remains the same, with the first data member denoting one name for that location, while subsequent data members serve as alternate names for the same location. For instance, consider the intOrChar union containing an integer and a character as data members. The size of this union would be equal to the largest data member's size. Therefore, if an int occupies four bytes and a char occupies one byte, the intOrChar union would occupy four bytes.
Similar to structures, we can utilize the dot .
operator to access any member of a union. Once we've declared objects of a particular union, we can operate on the fields that comprise them. To achieve this, we use a dot .
between the object name and the field name. Let's delve into an example to comprehend how a union functions precisely.
Suppose we have an integer value of 123 and aim to append 456 to it, resulting in 123456. To achieve this, we need to shift the integer three decimal places, effectively multiplying the integer by 1000 (i.e., 123000) and then adding 456 to it (i.e., 123456). Consider a union containing four characters and an integer. Given that the size of a char is 1 and an integer is 4, the size of the union would be four. If we assign the character 'a' to the integer and display the characters and integer value, we observe that the char variables of the union contain 'a'.
Take into account the following example: This program utilizes a union of int and char to showcase the memory usage of both data types.
#include <iostream>
using namespace std;
int main()
{
union intOrChar // Declaration of union;
{
char c[4];
int x;
} u1; // Declaration of Union_Object;
u1.x = 'a'; // Assigning ‘a’ to x
// Displaying the char array and integer value
cout << "The value of c = " << u1.c[0] << "," << u1.c[1]
<< "," << u1.c[2] << "," << u1.c[3] << endl;
cout << "The value of x = " << u1.x << endl;
// Shifting the values one byte and adding ‘b’ to the int
u1.x *= 256;
u1.x += 'b';
// Displaying the char array and integer value
cout << "The value of c = " << u1.c[0] << "," << u1.c[1]
<< "," << u1.c[2] << "," << u1.c[3] << endl;
cout << "The value of x = " << u1.x << endl;
// Shifting the values one byte and adding ‘b’ to the int
u1.x *= 256;
u1.x += 'c';
// Displaying the char array and integer value
cout << "The value of c = " << u1.c[0] << "," << u1.c[1]
<< "," << u1.c[2] << "," << u1.c[3] << endl;
cout << "The value of x = " << u1.x << endl;
// Shifting the values one byte and adding ‘b’ to the int
u1.x *= 256;
u1.x += 'd';
// Displaying the char array and integer value
cout << "The value of c = " << u1.c[0] << "," << u1.c[1]
<< "," << u1.c[2] << "," << u1.c[3] << endl;
cout << "The value of x = " << u1.x << endl;
return 0;
}
Unions are infrequently used but prove crucial when aiming for highly efficient programming. It's beneficial to experiment with unions and structures to gain a deeper understanding of their functionality.
We've now learned how to employ structures and unions. While these are relatively less used components of the C/C++ language, structures, in particular, are immensely useful. They facilitate a convenient means of grouping data about a single entity. By conceptualizing an object like a car and identifying its properties before grouping them in a structure, we streamline the manipulation of its properties. Rather than manipulating properties individually, consolidating them into a unit offers a superior approach. Try your hand at crafting different programs utilizing structures.
Differences between structures and unions in C/C++ are outlined in the table below. Despite their disparities, structures and unions share conceptual similarities.
Feature | Structure | Union |
---|---|---|
Declaration | The keyword "struct" is used. | The keyword "union" is used. |
Memory Size/th> | Size is greater than or equal to the sum of sizes of its members. Smaller members may result in unused stack bytes. | Size is equal to the size of the largest member. |
Member Access | Each member has a unique storage location. | Memory is shared among individual members. |
Addressing | Addresses of members ascend in order. | All members begin at the same offset. |
Access | Multiple members can be accessed simultaneously. | Only one member can be accessed at a time. |
Initialization | Several members can be initialized at once. | Only the first member can be initialized. |
Side Effects | Manipulating one member does not affect others unless coded to do so. | Manipulating one member can affect others. |
Activity | All data members are active simultaneously. | Only one data member is active at a time. |
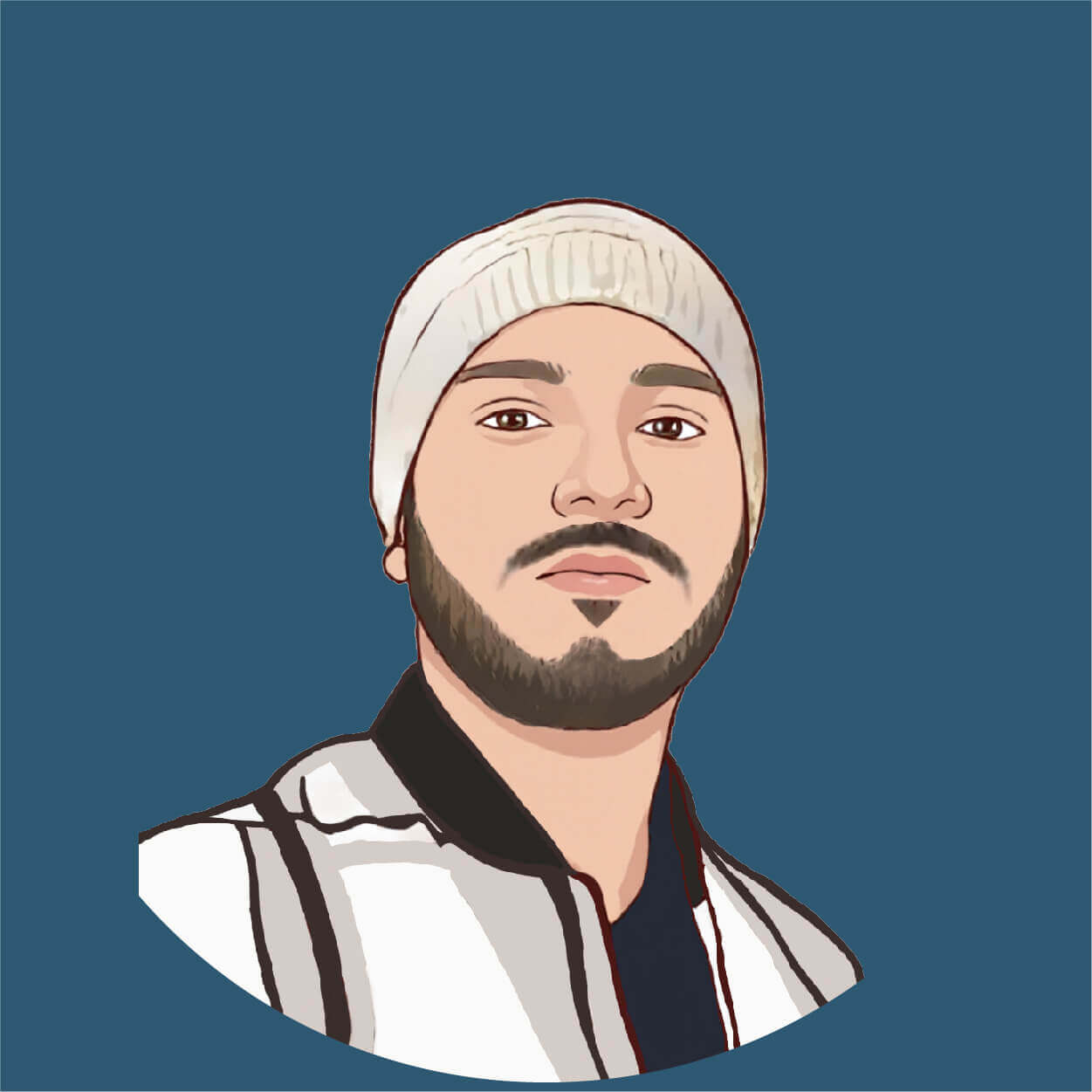
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.