PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
SWITCH STATEMENT IN C++:
The switch statement, commonly referred to as a multiple-choice statement, enables our program to select from various alternatives. This construct allows a variable to undergo equality tests with a list of values. Each of these values is termed a case, and the variable is evaluated against each case within the switch statement.
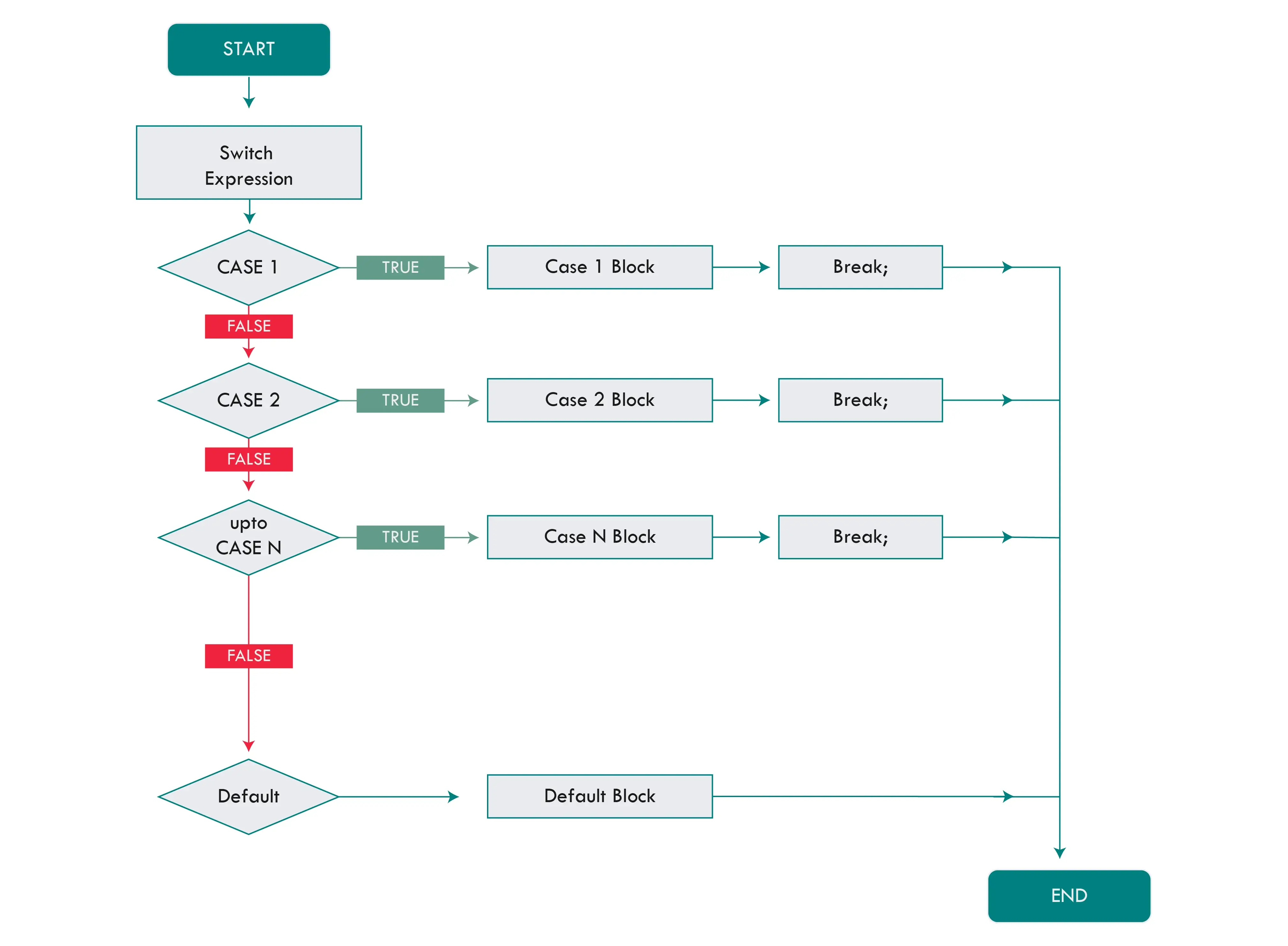
In the illustrated diagram, the Switch Expression is highlighted as an expression generating a case of integralor enumeratedtype. The Switch operation then compares the outcome of the "Switch Expression" with each integral value associated with a case. If a match is identified, the corresponding statement for that case is executed. In the event of no match in the initial case, the switch proceeds sequentially to the subsequent cases. If no matches are found, the default statement is triggered.
You might have noticed in the picture that every case ends with a Break
. This Break is like a signal that tells the program to stop checking cases and move to the end of the whole switch section. While this Break is commonly used and considered the standard way to write a switch statement, it's not mandatory. If a Break is missing, the program will continue executing the code for the following cases until it encounters a Break. Even though this behavior is usually not desired, experienced programmers might intentionally use it for specific reasons.
Let's delve into the syntax, rules, and examples to gain a comprehensive understanding.
Integral Types:
Integral types in C++ refer to data types that represent whole numbers, both positive and negative, without any fractional or decimal part. These types are used to store integer values. The primary integral types in C++ include: int, char, short, long.
Read MoreEnumerated Types:
Enumerated types (enums) in C++ are user-defined data types that allow you to define a set of named integral constants. Enums make the code more readable and maintainable by providing meaningful names to integral values. Enumerated types are particularly useful when you want to represent a set of related constant values.
Read Moreswitch (expression) { case constant_1: // Statement or group of statements to execute break; // Optional case constant_2: // Statement or group of statements to execute break; // Optional // UPTO N CASES default: // Default statement if no match is found // Statement or group of statements to execute }
Certain guidelines must be adhered to when using a switch statement:
- The expression in the switch statement must be of integralor enumeratedtype, or be of a class type with a single conversion function to an integral or enumerated type.
- Multiple case statements can be used within a switch block.
- The constant expression for each case must match the data type of the switch variable and be a constant or a literal.
- When the switch variable matches a case, the statements following that case will execute until a break statement is reached.
- The break statement terminates the switch, and control jumps to the next line following the switch block.
- Not every case needs to contain a break; if omitted, the control falls through to subsequent cases until a break is encountered.
- A switch statement can have an optional default case, positioned at the end, which executes when none of the cases match. No break is needed in the default case.
To understand the concept of a Switch Statement, let's consider an example. In this example, we'll create a program that prompts students to enter their grades. Once a student enters their grade, the program will display a corresponding comment about that grade. Let's begin.
Given that C++ is a case-sensitive programming language, "A" and "a" are considered distinct grades within a switch statement. If the case constant-expression is "A" and the user enters "a," the switch statement will execute the default statement since there is no match for "a" in the case constant-expression. To prevent such errors and accommodate both capital "A" and small "a," we can include two cases simultaneously, each with the same statement, as demonstrated in the following example:
#include <iostream> using namespace std; int main() { char grade; cout << "Enter Your Grade: (Only A, B, C, and D grades are available): "; cin >> grade; switch (grade) { case 'A': case 'a': cout << "Outstanding! Continue the good work. \n"; break; case 'B': case 'b': cout << "Well done! Pay a bit more attention. \n"; break; case 'C': case 'c': cout << "Fair. Pay attention to your studies. \n"; break; case 'D': case 'd': cout << "Fail. Kindly bring your parents tomorrow. \n"; break; default: cout << "The grading system includes only A, B, C, and D grades. \n"; } return 0; }
The provided example demonstrates the effective utilization of a switch statement to manage various cases according to user input. Feel free to copy and paste this code into your compiler and experiment with it. Try eliminating the Break
statement to understand its functionality. Additionally, input any letter other than A, B, C, and D to observe how the default
case functions.
Consider another practical example of a switch statement: In this case, we'll design a straightforward calculator capable of performing addition (+), multiplication (*), subtraction (-), and division (/). The operator will serve as the case constant-expression, and the switch expression will operate according to the specified operator.
#include<iostream> using namespace std; int main() { float a, b, result; char operation; cout << "Input your question (e.g., 2+3) and press Enter. \n"; cout << "Limited to operations: Multiplication, Addition, Subtraction, and Division." << endl; cin >> a >> operation >> b; switch(operation) { case '+': result = a + b; break; case '-': result = a - b; break; case '*': result = a * b; break; case '/': result = a / b; break; default: cout << "Invalid operation. Please use the correct format. \n"; return -1; } cout << "Result = " << result << endl; return 0; }
In the example above, did you catch that we used return -1
instead of return 0
in the default case? We did this because if someone enters an invalid operation, we want to signal that something went wrong. Choosing "-1" is just a common way to show an error or an unexpected end to the program.
The switch statement is a more efficient and readable choice when dealing with multiple conditions compared to if-else statements. The latter can become computationally expensive and lead to lengthy and less efficient code. The switch statement provides a cleaner and more concise solution, as showcased in the provided examples.
if ( grade == 'A' ) cout <<" Excellent " ; else if (grade == 'B') cout<<" Very Good"; else if ( grade == 'C' ) cout <<" Good " ; else if ( grade == 'D' ) cout << " Poor " ; else if ( grade == 'F' ) cout <<" Fail " ; else { //statements }
Click here to explore and learn more about the if-else statement.
Switch ( grade ) { case 'A' : cout << " Excellent " ; case 'B' : cout << " Very Good " ; case 'C' : cout << " Good " ; case 'D' : cout << " Poor " ; case 'F' : cout << " Fail " ; }
In the example provided, we made a program that gives feedback about a grade when the user inputs it. We used both If-else and switch statements to achieve the same outcome. However, it's noticeable that the "Switch statement" is more efficient and offers a clearer, easier-to-read code.
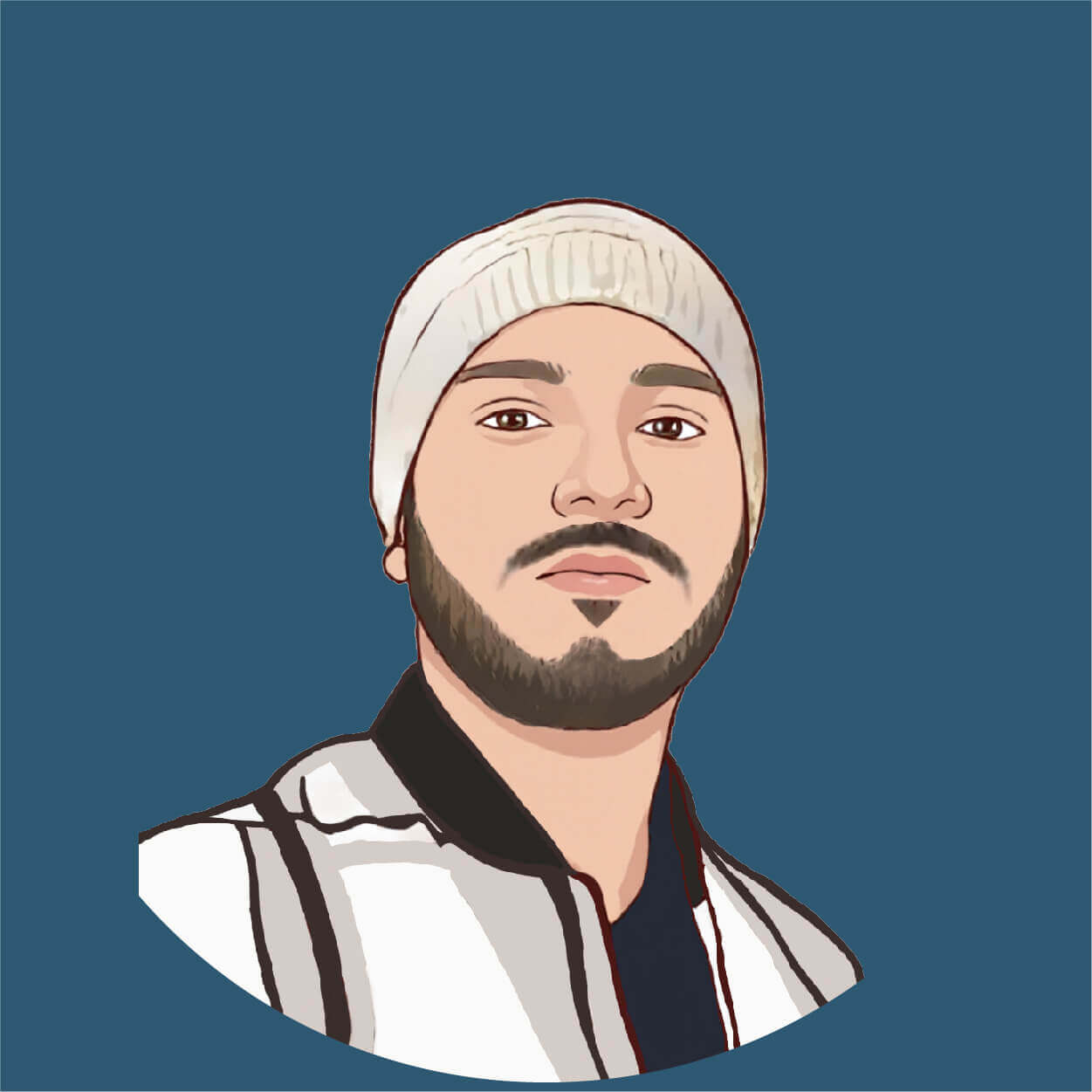
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.