PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Introduction to Object-Oriented Programming (OOP):
Object-Oriented Programming (OOP) is a fundamental paradigm utilized across various programming languages, with C++ being a prominent example. What sets C++ apart from C is its robust support for Object-Oriented Programming, marking a significant shift in the way programs are conceptualized and developed. Widely recognized as one of the most powerful and influential methodologies in computer programming, OOP has garnered widespread adoption. Alongside C++, other languages such as Java, PHP, and VB.NET also embrace OOP principles.
OOP represents a synthesis of the best concepts from structured programming, introducing novel ideas that revolutionize program organization. Traditionally, programs are structured around their code, focusing on sequential execution. However, OOP redefines this approach by organizing programs around data, emphasizing interactions between entities rather than mere code execution.
Object-Oriented Programming (OOP) incorporates all the features of procedural programs, including variables operated on by sequential instructions, selection, and loop statements. However, OOP introduces a paradigm shift, requiring a distinct mindset and introducing novel programming concepts, such as:
- Analysis of objects, encompassing their attributes and associated tasks.
- Sending messages to objects to trigger actions.
- Adaptive message handling across diverse objects.
- Methods capable of handling various data types without multiple names.
- Inheritance, enabling objects to acquire traits from predecessors.
- Enhanced information hiding compared to procedural approaches.
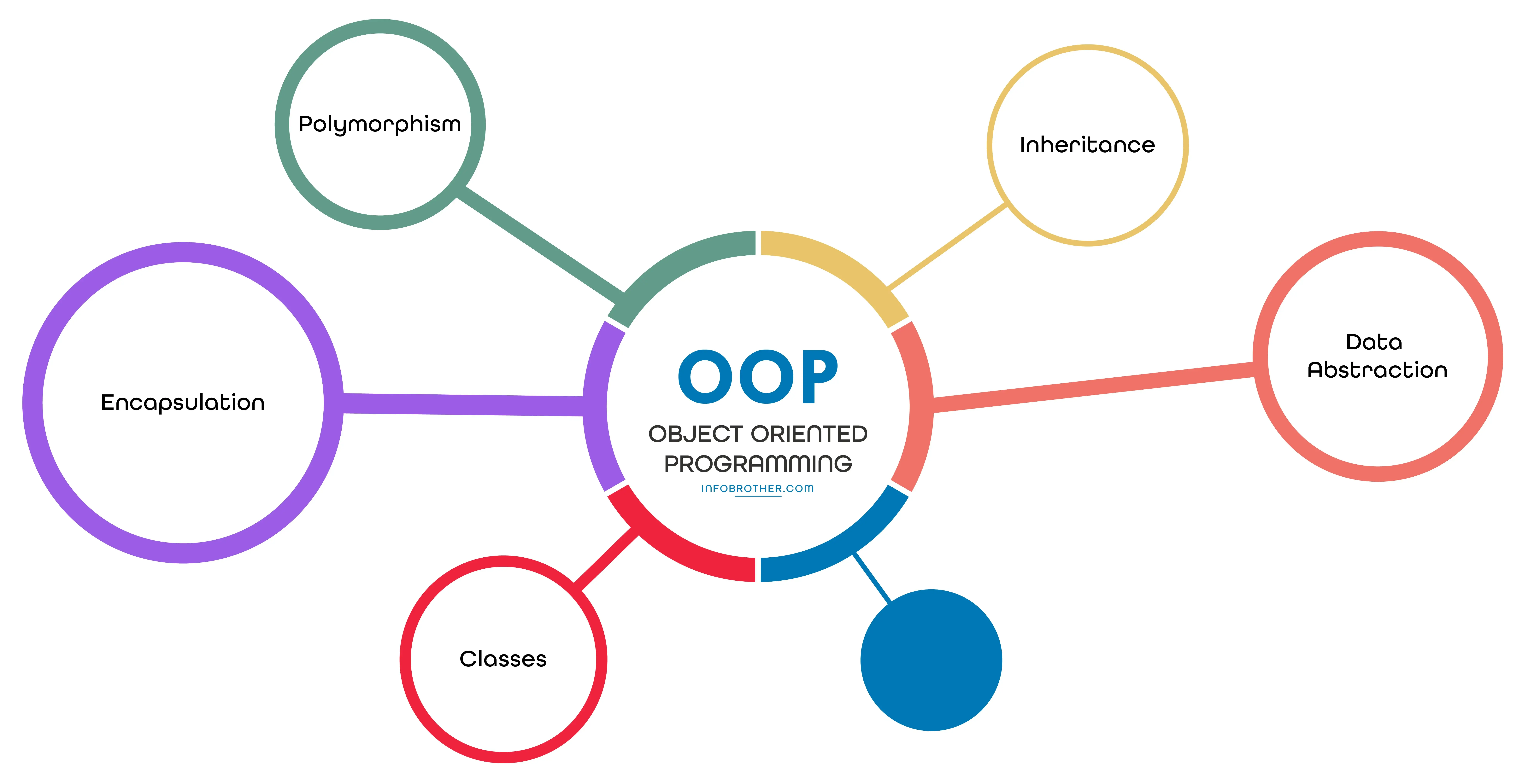
Object-Oriented Programming is structured around the following fundamental concepts:
Classes refers to the concept of building complex objects by combining simpler objects. In C++, classes can have member variables that are objects of other classes. This allows for the creation of more complex relationships between objects, enabling code organization and enhancing code reusability.
Read More...
Abstraction is a fundamental concept in OOP that involves simplifying complex systems by breaking them down into smaller, more manageable components. In C++, abstract classes and interfaces are used to define common attributes and behaviors that derived classes must implement. Abstract classes cannot be instantiated but serve as blueprints for creating derived classes.
Read More...
Encapsulation is the practice of bundling data and its related operations (methods) within a class. It allows the class to hide its implementation details and only expose the necessary features through a well-defined interface. The idea is to achieve data abstraction, where the internal representation of an object is hidden from the outside world.
Read More...
Inheritance is a mechanism where one class inherits the properties and behaviors of another class. The class being inherited from is called the base class or super class, and the class inheriting from it is called the derived class or sub class. Inheritance allows for code reuse and promotes the concept of hierarchy, where more specialized classes build upon generalized ones.
Read More...
Polymorphism means the ability to take many forms. In the context of OOP, polymorphism allows objects of different classes to be treated as instances of a common base class. Polymorphism is achieved through two main mechanisms: function overloading and function overriding. Function overloading enables a class to have multiple functions with the same name but different parameters, while function overriding allows a derived class to provide its own implementation of a method inherited from a base class.
Read More...
Traditional procedural programming, as seen in languages like C, tends to approach program structuring by decomposing tasks into sequential steps and functions. However, this method becomes unwieldy and convoluted, especially with complex data structures or extensive task sets.
Consider the example of modeling the operation of a laptop. In procedural programming, managing numerous variables related to various laptop components becomes cumbersome, lacking a cohesive structure to encapsulate related functionalities.
To address this complexity, OOP offers a more elegant solution. By encapsulating functionalities within discrete, self-contained units called objects, programmers can conceptualize interactions between objects more abstractly. This leads to modular, maintainable codebases with a focus on interaction dynamics.
It's worth noting that procedural programs follow a linear sequence of steps or procedures, where the programmer dictates the exact conditions, frequency, and termination of each procedure.
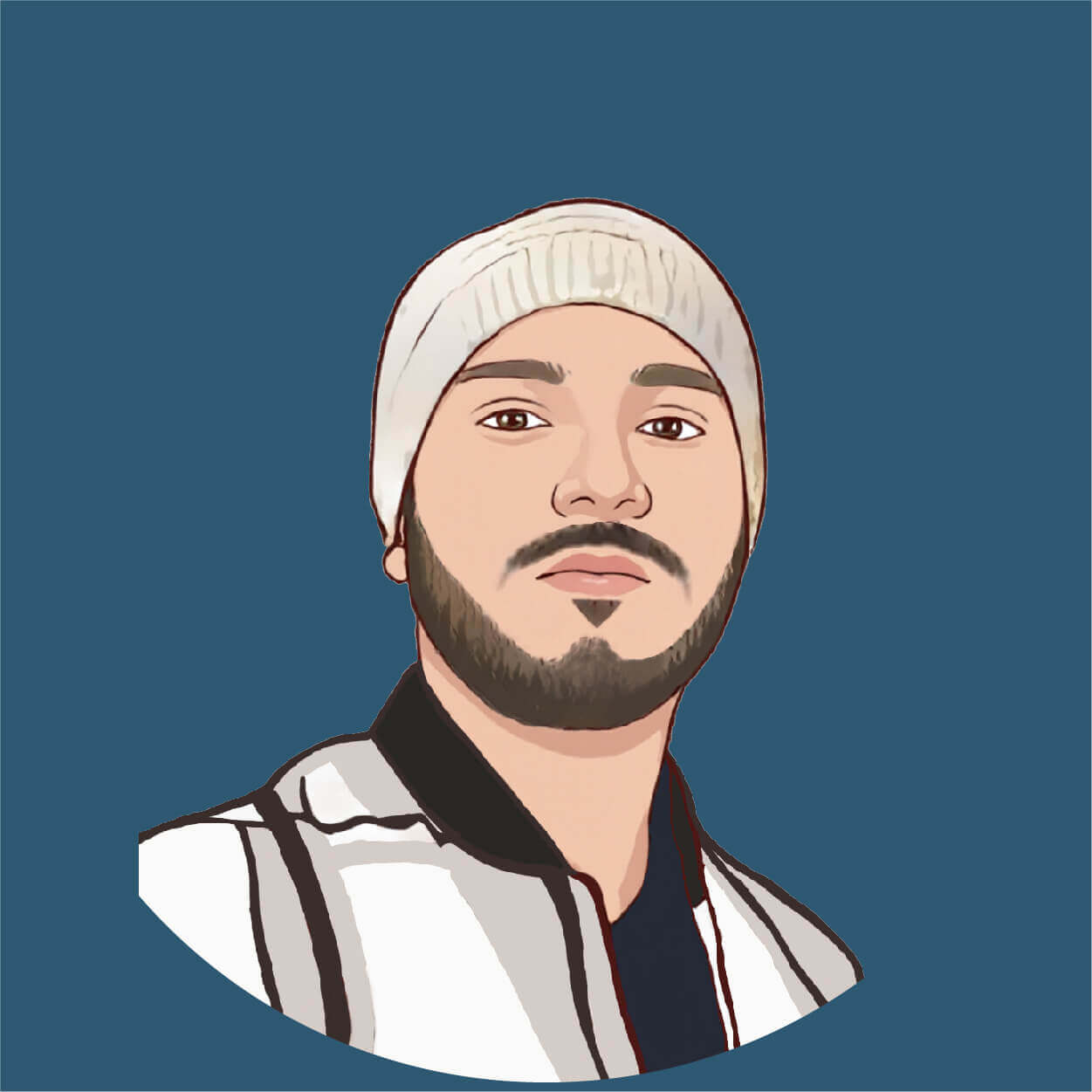
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.