PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
SEQUENTIAL FILES:
Learn how to work with sequential files in C++ for efficient file handling. This tutorial covers opening, closing, reading, writing, and deleting files, with examples demonstrating the usage of fstream, ifstream, and ofstream objects. Gain insights into file manipulation, including insertion operators, getline functions, and error handling, enhancing your skills in C++ file operations.
A sequential file must be accessed in the same order it was written. Consider the example of cassette tapes: music is played in the order it was recorded. We can quickly fast-forward or rewind to skip songs, but the order dictates how we play them. Inserting data in the middle of a sequential file is difficult and often impossible. The only way to truly add or delete records from the middle of a sequential file is to create a completely new file that combines both old and new records.
With sequential files, we can perform the following operations in the C++ programming language:
In C++, opening, closing, or manipulating files requires some background knowledge to handle the complexity of the operations. However, the C++ library provides a straightforward way to manipulate files using the <iostream>
and <fstream>
libraries.
Ensure you include the <iostream>
and <fstream>
header files in your program to perform file operations.
A file must be opened before it can be read from or written to. We use the <fstream>
object to open a file for both reading and writing.
- The
ifstream
object is used for reading only, - The
ofstream
object is used for writing only.
The standard syntax to open a file is:
fstream myFile;
myFile.open("filename.txt", ios::openMode);
Here, myFile
is an object of the fstream class. The open()
function requires two arguments: the filename and the file opening mode.
A file can be opened in different modes to perform reading and writing operations. C++ supports the following file open modes:
File Mode Parameter | Meaning | Explanation |
---|---|---|
ios::in | Read | Open the file for reading only. If the file doesn't exist, it will generate an error. |
ios::out | Write | Open the file for writing only. If the file already exists, this mode will open the file and format it. If the file doesn't exist, a new file is created. |
ios::app | Appending | Open the file for appending data to the end of the file. This mode allows writing only at the end of the file. |
ios::ate | Appending | Similar to "ios::app". Opens the file and positions the cursor at the end of the file but allows writing anywhere in the file. |
ios::binary | Binary | Open the file in binary mode. |
ios::trunc | Truncate | Open the file and discard all existing data. |
ios::nocreate | Don't Create | Open an existing file. If the file doesn't exist, it will show an error. |
ios::noreplace | Don't Replace | Open a new file. If the file already exists, it will show an error. |
The default value for the fstream
mode parameter is ios::in
| ios::out
meaning the file is opened for both reading and writing.
- For
ofstream
the default mode isios::out
- For
ifstream
the default mode isios::in
We can use more than one file mode using the bitwise operator |
.
A file must be closed after all operations are completed. When a C++ program terminates, it automatically flushes all streams, releases allocated memory, and closes all opened files. However, it is good practice for the programmer to close all opened files before the program terminates.
The standard syntax to close a file is:
file_object.close();
The close()
function is a member function of fstream, ifstream, and ofstream objects. Here is an example of opening and closing a new file:
#include <iostream> // Required for input & output
#include <fstream> // Required for file operations
using namespace std;
int main() {
// Create a new file in write mode
fstream myFile;
cout << "WRITING PURPOSE:" << endl;
myFile.open("InfoBrother.txt", ios::out); // Open file for writing
if (!myFile) { // Check for errors if file didn't open
cout << "Error while opening file." << endl;
} else {
cout << "New file opened successfully for writing." << endl;
}
myFile.close(); // Close the file
if (!myFile.is_open()) { // Check if file is closed
cout << "File has been closed." << endl;
} else {
cout << "File is still open." << endl;
}
// Open an existing file for reading and appending
cout << "\n\nREADING & APPENDING PURPOSE:" << endl;
myFile.open("InfoBrother.txt", ios::in | ios::app);
if (!myFile) {
cout << "Error while opening file." << endl;
} else {
cout << "Existing file opened successfully for reading & appending." << endl;
}
// Let's not close the file and check for errors
if (!myFile.is_open()) {
cout << "File has been closed." << endl;
} else {
cout << "File is still open." << endl;
}
return 0;
}
If you copy and compile the above example, you will find the InfoBrother.txt file in the folder where you are compiling your C++ programs.
In the above example, an object myFile of class fstream
is used to open and close the file "InfoBrother.txt". Error checkers ensure that the file operations are performed correctly. Including error checkers in your program is a good practice to minimize errors.
Fstream Member Functions:
open(): Opens the file and takes two arguments: the filename and the opening mode.
close(): Closes the opened file and doesn't require any arguments.
is_open(): Checks if the file is open. Returns true if the file is open, else returns false.
Now that we know how to open and close files, let's learn how to write to and read from files in C++. We write information to a file using the stream insertion operator <<
, similar to how we use it to output information to the screen. The difference is that we use an ofstream or fstream object instead of the cout object.
To write to files, we can utilize the following methods:
This function gets input character by character, including spaces, tabs, and newlines, up to a specified size and assigns it to a variable. It is useful for reading a limited number of characters into a specific variable. It needs two arguments: a character array and the size of the array. An optional parameter is a delimiter ('\n' by default).
get(ch, size);
Here's a straightforward example demonstrating how to use the get()
function to obtain input characters in our C++ program.
#include <iostream> // Required for input & output
#include <fstream> // Required for file operations
using namespace std;
int main() {
ofstream myFile; // Object of type ofstream
char name[30];
int age;
cout << "Enter Your Name: ";
cin.get(name, 30); // Use get function to input name
cout << "Enter Your Age: ";
cin >> age;
myFile.open("InfoBrother.txt", ios::out); // Open the file
if (myFile) { // Check if file opened successfully
myFile << name << endl;
myFile << age << endl;
cout << "Data stored successfully." << endl;
} else {
cout << "Error occurred while opening file." << endl;
}
return 0;
}
Similar to get()
, but reads an entire line of text ending with a newline character. It requires two parameters: a character array and the size of the array. An optional parameter is a delimiter ('\n' by default).
getline(array_name, size);
Here's a straightforward example demonstrating how to use the getline()
function to obtain a line of input in our C++ program.
#include <iostream> // Required for input & output
#include <fstream> // Required for file operations
using namespace std;
int main() {
ofstream myFile; // Object of type ofstream
char str[100]; // Array to store input text
cout << "Enter your text here: ";
cin.getline(str, 100); // Use getline() function to read input
myFile.open("InfoBrother.txt", ios::out); // Open the file
if (myFile) { // Check if file opened successfully
myFile << str << endl;
cout << "Data stored successfully." << endl;
} else {
cout << "Error occurred while opening file." << endl;
}
return 0;
}
Similar to cin
, we can use fstream or ofstream objects to get data from the user with the insertion operator.
ofstream file; // 'file' is an object of type ofstream
file << "Text...";
Here's a straightforward example demonstrating how to use the stream insertion operator <<
to input data in our C++ program.
#include <iostream> // Required for input & output
#include <fstream> // Required for file operations
using namespace std;
int main() {
ofstream fp; // Object of type ofstream
fp.open("InfoBrother.txt", ios::out); // Open the file in write mode
if (fp) { // Check if the file opened successfully
fp << "InfoBrother" << endl;
fp << "Sardar Omar" << endl;
fp << "C++ Tutorial" << endl;
fp << "Object Oriented Programming" << endl;
} else {
cout << "Error occurred while opening file." << endl;
}
return 0;
}
Once data is in a file, we must be able to read it. We need to open the file in read access mode ios::in
. There are several ways to read data: character by character or line by line, depending on the format of the data. Files opened for read access must already exist, or C++ will give an error.
Let's create a simple program to open a file and read its contents. We will open the file using ios::in
mode, so the file must already exist.
It's better to place your file in the same folder where you compile your program. Otherwise, you need to provide the complete path to open the file.
#include <iostream> // For input & output
#include <fstream> // For file operations
using namespace std;
int main() {
ifstream myFile; // File input object
char fileName[20]; // Filename storage
char ch; // Character for reading data
cout << "Enter the file name: ";
cin >> fileName;
myFile.open(fileName, ios::in); // Open file for reading
if (!myFile) { // Check if file opened
cout << "Error opening file." << endl;
} else { // File opened successfully
cout << "File opened successfully. Contents:" << endl;
while (!myFile.eof()) { // Read till end of file
myFile.get(ch); // Read character
cout << ch; // Display character
}
}
myFile.close(); // Close the file
return 0;
}
This program reads from the file "InfoBrother.txt". Since newline characters are in the file at the end of each line, the names appear on-screen, one per line. If we attempt to read a file that does not exist, our error checker will show an error.
Let's create another program, a copy program. This program reads from one file and copies its contents to another. It opens two files, one for reading and the other for writing.
In the above example, we use the eof() function to indicate the end-of-file.
#include <fstream> // For file functions
#include <iostream> // For input & output
using namespace std;
int main() {
char File1[20], File2[20], ch; // Variables for filenames and characters
ifstream in_file; // Input file object
ofstream out_file; // Output file object
cout << "Enter the name of the file to be copied: ";
cin >> File1;
cout << "Enter the name of the destination file: ";
cin >> File2;
in_file.open(File1, ios::in); // Open file for reading
if (!in_file) { // Check if file opened
cout << "Error opening source file." << endl;
return 1;
}
out_file.open(File2, ios::app); // Open file for appending
if (!out_file) { // Check if file opened
cout << "Error opening destination file." << endl;
return 1;
}
while (in_file.get(ch)) { // Read and copy data
out_file.put(ch);
}
in_file.close(); // Close files
out_file.close();
cout << "Copy successful." << endl;
return 0;
}
In the above example, we copy from one file and paste it into another. The program reads data character by character and pastes it into a new file character by character.
In sequential files, we can delete a complete file using the remove()
member function. This function takes one argument, which is the name of the file. The basic syntax for deleting a file is:
remove("filename");
The default access mode for file access is text mode, but we can change this default access mode to any extension. However, it's better to use binary modes.
Explore these real-world examples of file handlingto understand how these functions operate and how you can effectively manage files in C++ programming.
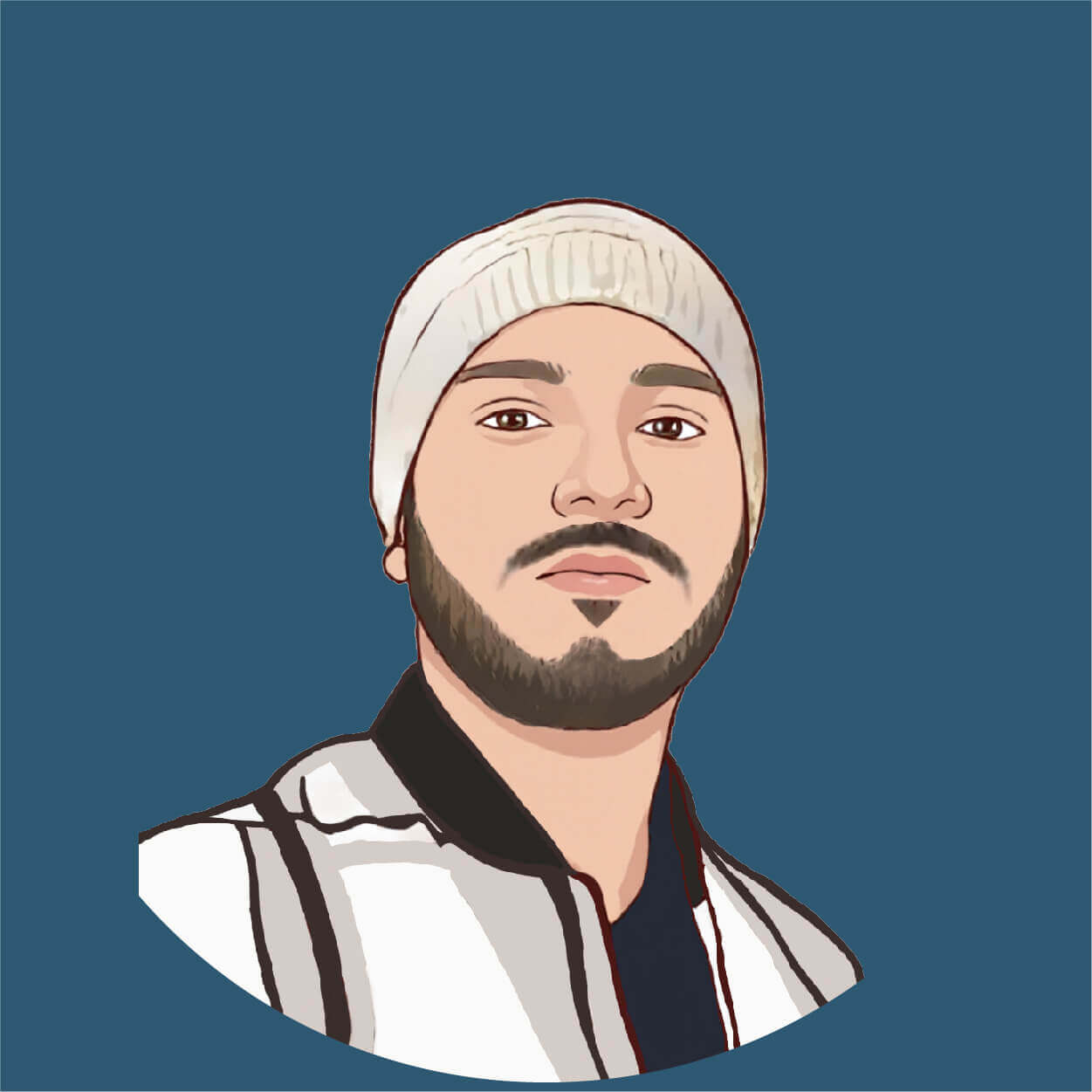
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.