PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Understanding Operators in C++:
In C++, operators are symbols that instruct the compiler to perform specific mathematical or logical manipulations. These operators take one or more arguments and produce a new value. C++ provides various types of operators to support a wide range of operations:
Arithmetic operators are employed for calculations. C++ supports various arithmetic operators, as demonstrated below:
Operator | Name | Description | Syntax |
---|---|---|---|
+ | Addition | Adds two operands. | x + y |
- | Subtraction | Subtracts second operand from the first. | x - y |
* | Multiplication | Multiplies both Operands. | x * y |
/ | Division | Divides Numerator by de-numerator. | x / y |
% | Modulus | Modulus Operator return the remainder of an integral division. | x % y |
You can explore all arithmetic operators through a comprehensive C++ program. Feel free to copy and run the code to understand the operations involved.
#include <iostream> using namespace std; main() { int x = 10; int y = 5; // endl is used to insert newline. cout << "x + y Will give " << x+y << endl; cout << "x - y Will give " << x-y << endl; cout << "x * y Will give " << x*y << endl; cout << "x / y Will give " << x/y << endl; cout << "x % y Will give " << x%y << endl; return 0; }
Relational Operators define a connection between the values of operands. The C++ programming language supports the following relational operators for this purpose.
Operator | Name | Description | Syntax |
---|---|---|---|
== | Equal to | Verifies whether the values of two operands are equal or not; if true, the condition is satisfied. It is important to note that the single equal sign (=) is utilized in C++ to assign a value to variables. | x==y |
!= | Not Equal to | Examines whether the values of two operands are unequal; if they are not equal, the condition is satisfied. | x !=y |
Greater than | Determines if the value of the left operand is greater than that of the right operand; if true, the condition is satisfied. | x >y | |
< | Less than | Examines whether the value of the left operand is less than that of the right operand; if true, the condition is satisfied. | x < y |
= | Greater than or Equal to. | Checks if the value of the left operand is greater than or equal to the value of the right operand; if true, the condition is satisfied. | x >=y |
<= | less than or Equal to. | Determines if the value of the left operand is less than or equal to the value of the right operand; if true, the condition is satisfied. | x <=y |
Consider an example to grasp the various relational operators present in C++. In this example, we will utilize if and else statements to demonstrate the relationships between operands. Run the code yourself to gain a practical understanding of how these relational operators operate.
#include <iostream> using namespace std; main() { int x = 10; int y = 5; if(x == y) cout << " x is equal to y: "<< endl; else cout << " x is not equal to y: "<< endl; if(x > y) cout << " x is greater then y: "<< endl; else cout << " x is less then y: "<< endl; if(x >= y) cout << " x is greater than or equal to y: "<< endl; else cout << " x is not greater than or equal to y: "<< endl; return 0; }
Learn more about the if and else statementsby reading here:
The logical operator generates a true or false outcome based on the logical association of its arguments. It's important to note that in C and C++, a statement is considered true if it possesses a non-zero value and false if it has a value of zero. When printing a bool, it commonly displays 1
for true and 0
for false. The C++ language supports the following logical operators.
Operator | Name | Description | Syntax |
---|---|---|---|
&& | Logical AND | The condition becomes true if both operands are non-zero. It's important to note that the single ampersand (&) serves another purpose in C++. | x && y |
|| | Logical OR | The condition becomes true if either of the two operands is non-zero. | x || y |
! | Logical NOT | It is employed to invert the logical state of its operands. If a condition is true, the Logical NOT operator will render it false. | !(x && y) |
Here's an example using if and else statements.Feel free to copy and run the code to understand how logical operators work.
#include <iostream> using namespace std; main() { int x = 1; int y = 0; //logical AND if(x && y) cout << "Condition is true: "<< endl; else cout << "Condition is not true: "<< endl; //Logical OR if(x || y) cout << "Condition is true: "<< endl; else cout << " Condition is not true: "<< endl; //Logical NOT if(!(x && y)) cout <<" Condition is true: "<< endl; else cout << " Condition is not true: "<< endl; return 0; }
The Bitwise Operators enable the manipulation of individual bits in a number. It's important to note that bitwise operators work only with integral types ( char
, int
, and long
) since floating-point values use a special internal format. These operators perform Boolean algebra on the corresponding bits in the arguments to generate the result.
The C++ programming language includes the following bitwise operators:
Operator | Name | Description | Syntax |
---|---|---|---|
& | Binary AND | The Binary AND Operator duplicates a bit in the result if it is present in both operands. | x & y |
| | Binary OR | The Binary OR Operator duplicates a bit if it is present in either operand. | x | y |
^ | Binary XOR | The Binary XOR Operator duplicates a bit if it is set in one operand but not in both. | x ^ y |
~ | Binary Ones Complement | The Binary Ones Complement Operator is Unary and results in the "flipping" of bits. | ~x |
<< | Binary Left Shift | The value of the left operand is shifted left by the number of bits specified by the right operand. | x << y |
Binary Right Shift | The value of the left operand is shifted right by the number of bits specified by the right operand. | x >y |
Consider an example to comprehend all the available Bitwise operators in C++. Feel free to replicate and execute the code to gain a practical understanding of the operations performed by these Bitwise operators.
#include <iostream> using namespace std; main() { unsigned int x = 60; unsigned int y = 13; int z = 0; z = x & y; // 12 = 0000 1100 cout << "Using Binary AND Operator the value of z is: " << z << endl ; z = x | y; // 61 = 0011 1101 cout << "Using Binary OR Operator the value of z is: " << z << endl ; z = x ^ y; // 49 = 0011 0001 cout << "Using Binary XOR Operator the value of z is: " << z << endl ; z = ~x; // -61 = 1100 0011 cout << "Using Binary Ones Complement Operator the value of z is: " << z << endl ; z = x << 2; // 240 = 1111 0000 cout << "Using Binary Left shift Operator the value of z is: " << z << endl ; z = x >> 2; // 15 = 0000 1111 cout << "Using Binary Right shift Operator the value of z is: " << z << endl ; return 0; }
Assignment operators are utilized to assign the value of the right-side operand to the left-side operand. The C++ programming language supports the following assignment operators:
Operator | Name | Description | Syntax |
---|---|---|---|
= | Assignment | The Simple Assignment Operator assigns values from the right-side operands to the left-side operand. Assign the sum of x and y to z. | z=x + y |
+= | Add and Assignment | The Add and Assignment Operator adds the right operand to the left operand and assigns the result to the left operand. z +=x is equivalent to z=z + x. | z +=x |
-+ | Subtract and Assignment | he Subtract and Assignment Operator subtracts the right operand from the left operand and assigns the result to the left operand. z -=x is equivalent to z=z - x. | z -=x |
*= | Multiply and Assignment | The Multiply and Assignment Operator multiplies the right operand with the left operand and assigns the result to the left operand. z *=x is equivalent to z=z * x. | z *=x |
/= | Divide and Assignment | The Divide and Assignment Operator divides the left operand by the right operand and assigns the result to the left operand. z /=x is equivalent to z=z / x | z /=x |
%= | Modulus and Assignment | The Modulus and Assignment Operator takes the modulus using two operands and assigns the result to the left operand. z %=x is equivalent to z=z % x | z %=x |
<<= | Left Shift and Assignment | The Left Shift and Assignment Operator. z <<=2 is equivalent to z=z << 2 | z <<=2 |
= | Right shift and Assignment | The Right Shift and Assignment Operator. z >=2 is equivalent to z=z >2 | z >=2 |
&= | Bitwise AND Assignment | The Bitwise AND and Assignment Operator. z &=x is equivalent to z=z & x | z &=x |
^= | Bitwise XOR Assignment | The Bitwise Exclusive XOR and Assignment Operator. z ^=x is equivalent to z=z ^ x | z ^=x |
|= | Bitwise OR Assignment | The Bitwise Exclusive OR and Assignment Operator. z |=x is equivalent to z=z | x | z |=x |
Note:
In C++, double quotes ( " " ) carry a special significance. To include double quotes in a C++ program, a specific approach is required: using a backslash followed by double quotes. \" \"
Take a look at this example to better understand how assignment operators work. You can copy and run the code to see these operators in action and get hands-on experience with them.
#include <iostream> using namespace std; main() { int x = 12; int z; z = x; cout << " Using \"=\" operator: value of z is : "<< z << endl; z += x; cout << " Using \"+=\" operator: value of z is : "<< z << endl; z -= x; cout << " Using \"-=\" operator: value of z is : "<< z << endl; z *= x; cout << " Using \"*=\" operator: value of z is : "<< z << endl; z /= x; cout << " Using \"/=\" operator: value of z is : "<< z << endl; z %= x; cout << " Using \"%=\" operator: value of z is : "<< z << endl; z <<= 2; cout << " Using \"<<=\" operator: value of z is : "<< z << endl; z >>= 2; cout << " Using \">>=\" operator: value of z is : "<< z << endl; z &= 2; cout << " Using \"&=\" operator: value of z is : "<< z << endl; z ^= 2; cout << " Using \"^=\" operator: value of z is : "<< z << endl; z |= 2; cout << " Using \"|=\" operator: value of z is : "<< z << endl; return 0; }
C++ offers two unique operators for incrementing and decrementing variables. What makes them distinctive is that ++
and --
can be employed either as prefix operators or postfix operators, as illustrated in the table:
Operator | Name | Description |
---|---|---|
Suppose that: int x=10; int y=5; | ||
++ | Increment | The Increment Operator increases the value by one. For instance,x++ will result in 11. |
x++ | Postfix Increment | The Postfix Increment Operator increases the value by one after its current value has been utilized. Ify=x++, then y will be 10. This is because the assignment operator first stores the value of x (i.e., 10) in y and then increments x by 1. |
++x | Prefix Increment | The Prefix Increment Operator increases the value by one before its value is used. If y=++x, then y will be 11. This occurs because x is incremented by 1 first and then stored in y. |
-- | Decrements | The Decrement Operator decreases the integer value by one.x-- will result in 9. |
x-- | Postfix Decrements | The Postfix Decrement Operator decreases the value by one after its current value has been used. If y=x--, then y will be 10. This is because the assignment operator first stores the value of x (i.e., 10) in y and then decrements x by 1. |
--x | Prefix decrements | The Prefix Decrement Operator decreases the value by one before its value is used. If y=--x, then y will be 9. This happens because x is decremented by 1 first and then stored in y. |
In the realm of C++, there are several additional operators that prove to be quite useful, as detailed below. Each operator is designed for a specific purpose, and it's crucial to have a solid grasp of their functions for effective programming.
Operator | Description |
---|---|
Sizeof | Returns the size of a variable. For instance, sizeof(a) will return 4, where "a" is an integer. |
condition ? x:y | This operator works similarly to an if and else statement. If the condition is true, it returns the value of x; otherwise, it returns the value of y. |
Comma (.) | Causes a sequence of operations to be performed. The value of the entire comma expression is the value of the last expression in the comma-separated list. |
Member Operators (dot and arrow) | Used to reference individual members of classes, structures, and unions. |
cast | Convert one data type to another. For example, int(2.2000) would return 2. |
Pointer Operator "&" | Returns the address of a variable. For example, &x will give the actual address of the variable. |
Pointer Operator "*" | Represents a pointer to a variable. For example, *var; will point to a variable named var. |
Operators in C++ have both precedence and associativity. Precedence determines the order of evaluation, while associativity addresses situations with operators of the same precedence.
In expressions, parentheses () are employed to enforce the order of evaluation. Operators within the parentheses are prioritized and evaluated first. In cases of nested parentheses, the innermost set is evaluated initially. For example:
int x = 2 + 3 * 2;
Here, x is assigned the value 8, not 10. This is because multiplication (*) has a higher precedence than addition (+). Therefore, it first multiplies 3 by 2 and then adds 2 to the result. Let's consider another example.
int x = (2 + 3) * 2;
In this case, x is assigned the value 10, not 8. This is because parentheses () have a higher precedence than any other operator. Therefore, addition will be evaluated first, and then the result will be multiplied by 2.
The table below displays operators with the highest precedence at the top and those with the lowest precedence at the bottom. Within an expression, operators with higher precedence will be evaluated first.
CATEGORY | OPERATORS | ASSOCIATIVITY |
---|---|---|
Postfix | () [] ->. ++ -- | Left to Right |
Unary | + - ! ~ ++ -- (type)* & sizeof | Right to Left |
Multiplicative | * / % | Left to Right |
Additive | + - | Left to Right |
Shift | << > | Left to Right |
Relational | < <=>= | Left to Right |
Equality | ==!= | Left to Right |
Bitwise AND | & | Left to Right |
Bitwise XOR | ^ | Left to Right |
Bitwise OR | | | Left to Right |
Logical AND | && | Left to Right |
Logical OR | || | Left to Right |
Conditional | ?: | Right to Left |
Assignment | =+=-=*=/=%=>=<<=&=^=\= | Right to Left |
Comma | , | Left to Right |
Pay attention to detail when programming. Analyze problem statements carefully, consider flow, operator precedence, and associativity. Even a minor mistake can lead to logical errors that are challenging to identify. Be cautious while working with operators..
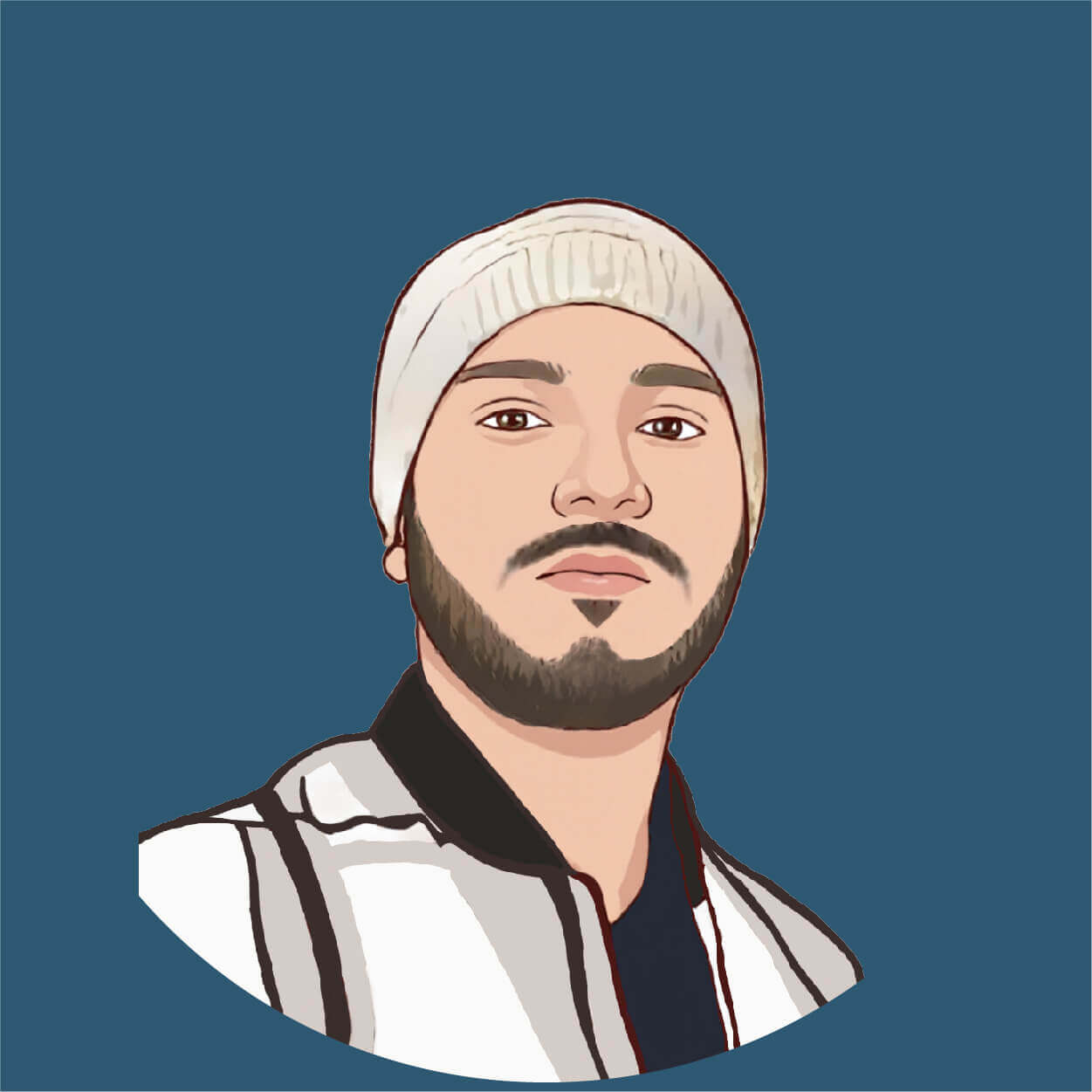
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.