PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
POINTER ARITHMETIC:
Pointers, as understood, represent addresses in memory and are treated as numeric values, allowing us to perform arithmetic operations on them just like we would with regular numbers. The fundamental arithmetic operators applicable to pointers are:
- Increment Operator (++):Increases the pointer value.
- Decrement Operator (--):Decreases the pointer value.
- Addition (+):Adds a numeric value to the pointer.
- Subtraction (-):Subtracts a numeric value from the pointer.
To grasp pointer arithmetic, let's consider an example where Ptr
is an integer pointer with a current value of 250, meaning Ptr
points to the address 250 in memory, assuming 32-bit integers. We'll perform the following arithmetic operations:
Ptr++;
After this increment operation, Ptr's contents will become 254, not 251. This behavior is due to each increment shifting the pointer to the next integer's memory location. Similarly, decrements follow the same logic. For instance:
Ptr--;
Following this decrement, Ptr's contents will be reduced to 246.
When a pointer holds the memory address of a simple variable, avoid incrementing or decrementing the pointer. However, for pointers pointing to an array, such operations are meaningful.
Pointers offer advantages over arraysbecause they allow for incrementing. Unlike array names, which remain constant pointers, each pointer increment directs it to the next element of its base type. Consider the following example:.
#include<iostream>
using namespace std;
main()
{
int var[5] = {10,20,30,40,50}; // Array Declaration.
int *ptr; // Pointer pointing to int.
ptr = var; // Storing array address in pointer.
for (int i = 0; i < 5; i++) // Loop to display Address and Value:
{
cout << "\n Address of var[" << i << "] = " << ptr << endl; // Display the Address.
cout << "Value of var[" << i << "] = " << *ptr << endl; // Display the value.
ptr++; // Move to the next location (incrementation).
}
return 0;
}
Decrementing a pointer operates similarly, reducing its value by the number of bytes of its data type. With each decrement, the pointer shifts to the previous element of its base type. Here's an illustrative example:
#include<iostream>
using namespace std;
main()
{
int var[5] = {10,20,30,40,50}; // Array Declaration.
int *ptr; // Pointer pointing to int.
ptr = &var[4]; // Pointer points to the last address of Array.
for (int i = 5; i > 0; i--) // Loop to display Address and Value:
{
cout << "\n Address of var[" << i << "] = " << ptr << endl; // Display the Address.
cout << "Value of var[" << i << "] = " << *ptr << endl; // Display the value.
ptr--; // Move to the previous location.
}
return 0;
}
Pointers can be compared using relational operators like Equal to ==
, Greater than , and Less than
<
. For meaningful comparison outcomes, the pointers should relate to each other, such as pointing to elements within the same array. Null pointer comparison, where a pointer is compared to the null pointer (zero), is another valid comparison.
#include<iostream>
using namespace std;
main()
{
int var[5] = {10,20,30,40,50}; // Array Declaration.
int *ptr; // Pointer pointing to int.
ptr = var; // Pointer points to the first address of Array.
int i=0; // Variable for the while loop.
while(ptr <= &var[4]) // Loop to display Address and Value:
{
for(int i=0; i<5; i++)
{
cout << "\n Address of var[" << i << "] = " << ptr << endl; // Display the Address.
cout << "Value of var[" << i << "] = " << *ptr << endl; // Display the value.
ptr++; // Move to the next location.
}
}
return 0;
}
Pointer arithmetic isn't limited to increment and decrement operations; we can also add or subtract integers from pointers. For instance, ptr=ptr1 + 5; moves Ptr1 to point to the ninth element beyond its current position.
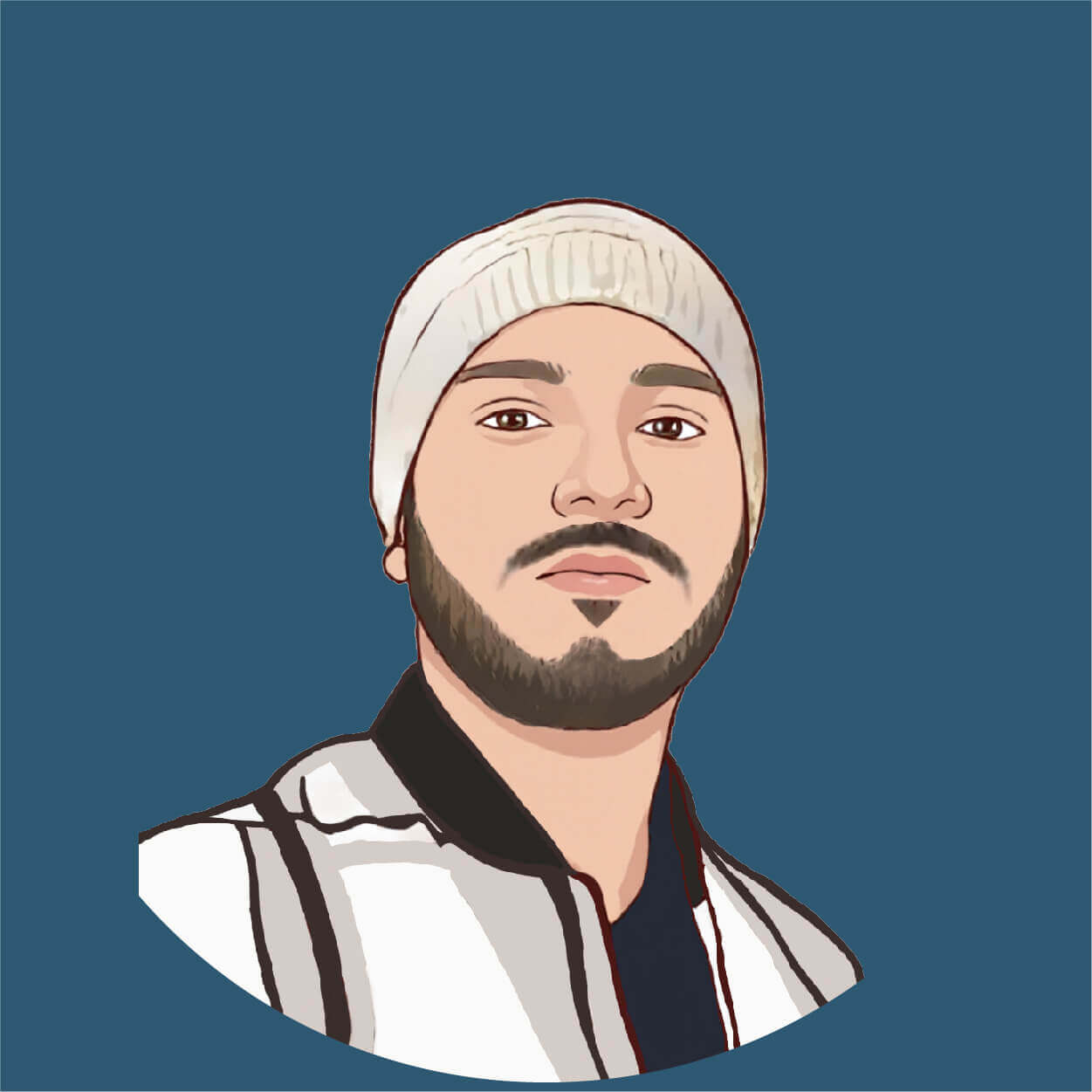
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.