PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Dynamic Memory
To become a proficient programmer, it is essential to understand how dynamic memory works. In C++ programs, memory is divided into two parts: the stack and the heap. To understand dynamic memory and its functionality, we need to explore the stack and heap. This tutorial will discuss the stackand heapand in future tutorials, we will implement dynamic memory in C++ using various tools.
A stack is an abstract data type commonly used in most programming languages. It operates on a Last-In-First-Out (LIFO) principle, similar to a stack of plates or cards where elements are added and removed from the top. When an element is added to the stack, it is placed on top, and only the top element can be removed.
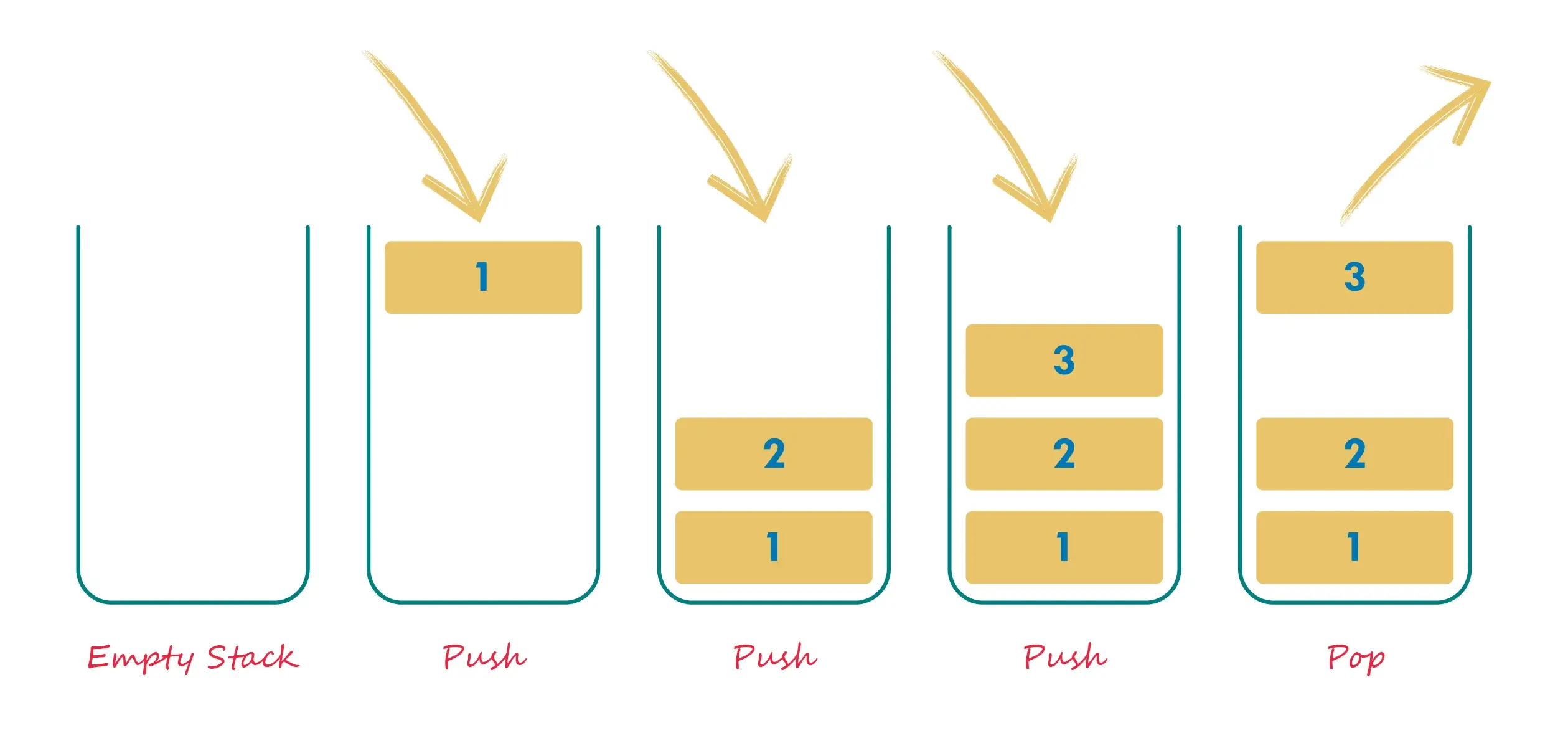
When a variable is declared inside or outside a function, it is stored in the stack. This means all declared variables reside in the stack.
Consider a pile of 10 books. To add another book, you simply place it on top of the pile. If you want the 5th book from the pile, you need to remove the top 4 books to access it. After taking the 5th book, you replace the removed books back on the pile in the same order.
In a stack, adding data (push) and removing data (pop) are operations performed only at the top.
To perform operations on the stack, we use two functions: PUSH
for insertion and POP
for removal. Every time a function declares a new variable, it is pushed onto the stack. When the function exits, all variables pushed onto the stack by that function are freed or deleted. Once a stack variable is freed, that memory region becomes available for other stack variables, so memory management is automatic and efficient.
Sometimes, the stack is not suitable when memory needs to be passed between different functions or kept alive for longer than a single function's execution. In such cases, we use the heap.
The heap is used to allocate memory dynamically at runtime.
Imagine you are in a library with many books and need a specific book, "Introduction to Heap in C++." You ask the librarian, who quickly fetches the book because it is well-organized and easily accessible, just like a heap. The place from where the book was taken remains empty until you return the book to the librarian, who places it back in its original position.
Stack | Heap |
---|---|
Very fast access | Relatively slower access |
Limited stack size, dependent on OS | No limit on memory size |
Automatic deallocation of variables | Manual memory management required |
Efficient space management by CPU | Potential for fragmented memory over time |
Variables cannot be resized | Variables can be resized using specific functions |
We can use the heap to allocate memory dynamically using one special operator and three different functions:
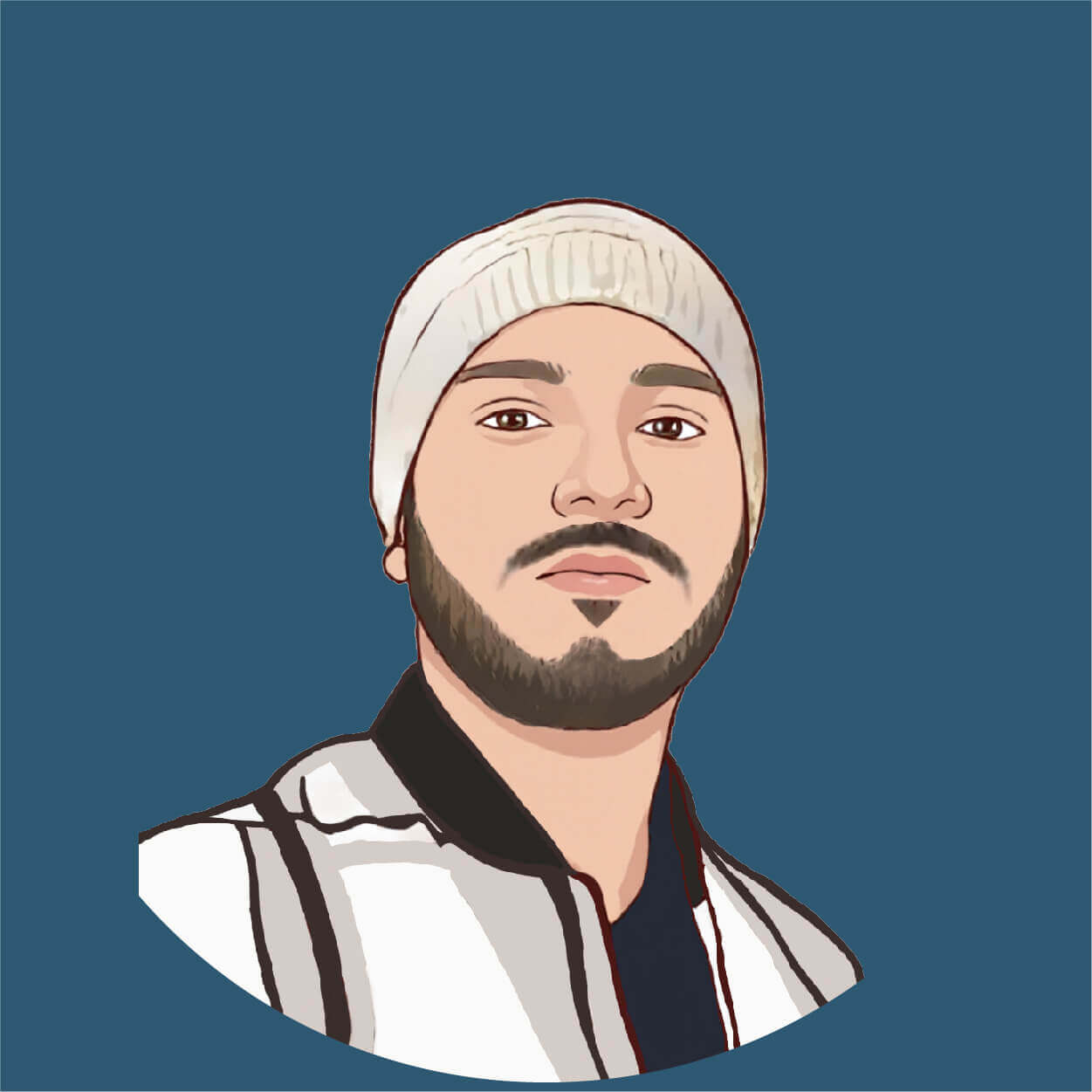
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.