PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Date & Time handling in C++:
The C++ standard library lacks a dedicated data type for handling date and time. Instead, it inherits date and time manipulation features from C through its structs and functions. To utilize these functionalities, including date and time-related structures and functions, we need to include the <ctime>
header file in our C++ programs. This header introduces four essential data types for time representation:
- clock_t
- time_t
- size_t
- tm
These data types primarily represent time as integers, necessitating conversion for commonly used time representations. The tm
structure type encapsulates date and time information within a C structure, comprising elements such as seconds, minutes, hours, day of the month, month, year, days since Sunday, days since January 1st, and the flag for daylight saving time.
struct tm {
int tm_sec; // seconds (0 to 61)
int tm_min; // minutes (0 to 59)
int tm_hour; // hours (0 to 24)
int tm_mday; // day of the month (1 to 31)
int tm_mon; // month (0 to 11)
int tm_year; // year since 1900
int tm_wday; // day of the week (0 to 6, Sunday to Saturday)
int tm_yday; // day in the year (0 to 365)
int tm_isdst; // daylight saving time flag
}
Here are key functions for working with date and time in C or C++:
Function | Purpose |
---|---|
time_t time(time_t *time); | Returns the current system calendar time in seconds elapsed since January 1, 1970. |
char*ctime(consttime_t*time); | Returns a string representing the current time in a specific format. |
structtm *localtime(consttime_t*time); | Returns a pointer to the tm structure representing local time. |
clock_t clock(void); | Approximates the time the program has been running. |
char*asctime(conststructtm *time); | Returns a string representing the time stored in the tm structure. |
structtm *gmtime(consttime_t*time); | Returns the time in a tm structure in Coordinated Universal Time (UTC). |
doubledifftime(time_ttime2, time_ttime1); | Calculates the difference in seconds between two times. |
size_t strftime(); | Formats date and time according to a specified format. |
time_t mktime(struct tm*time); | Converts the time stored in a tm structure to calendar time. |
To learn more about C++ structures,click here.
The tm
structure is crucial for user-friendly time representation, featuring various fields to represent different aspects of time.
Suppose we wish to retrieve the current system date and time, either as local time or as Coordinated Universal Time (UTC). The following example demonstrates how to achieve this:
#include <iostream>
#include <ctime>
using namespace std;
main() {
// current date/time based on current system
time_t now = time(0);
// convert to string form for local time
char* dt = ctime(&now);
cout << "The local date and time is: " << dt << endl;
// convert to tm struct for UTC
tm *gmtm = gmtime(&now);
dt = asctime(gmtm);
cout << "The UTC date and time is: " << dt << endl;
return 0;
}
The tm
structure plays a pivotal role in working with date and time in C or C++. It holds date and time information in a structured format. Below is an example utilizing various date and time-related functions and the tm
structure:
#include <iostream>
#include <ctime>
using namespace std;
main() {
// current date/time based on current system
time_t now = time(0);
cout << "Number of seconds since January 1, 1970: " << now << endl;
// convert to local time representation
tm *ltm = localtime(&now);
// print various components of tm structure
cout << "Year: " << 1900 + ltm->tm_year << endl;
cout << "Month: " << 1 + ltm->tm_mon << endl;
cout << "Day: " << ltm->tm_mday << endl;
cout << "Time: " << 1 + ltm->tm_hour << ":";
cout << 1 + ltm->tm_min << ":";
cout << 1 + ltm->tm_sec << endl;
return 0;
}
Please read the Data Structure in C++tutorial to gain a basic understanding of C structures and how to access structure members using the arrow "->" operator.
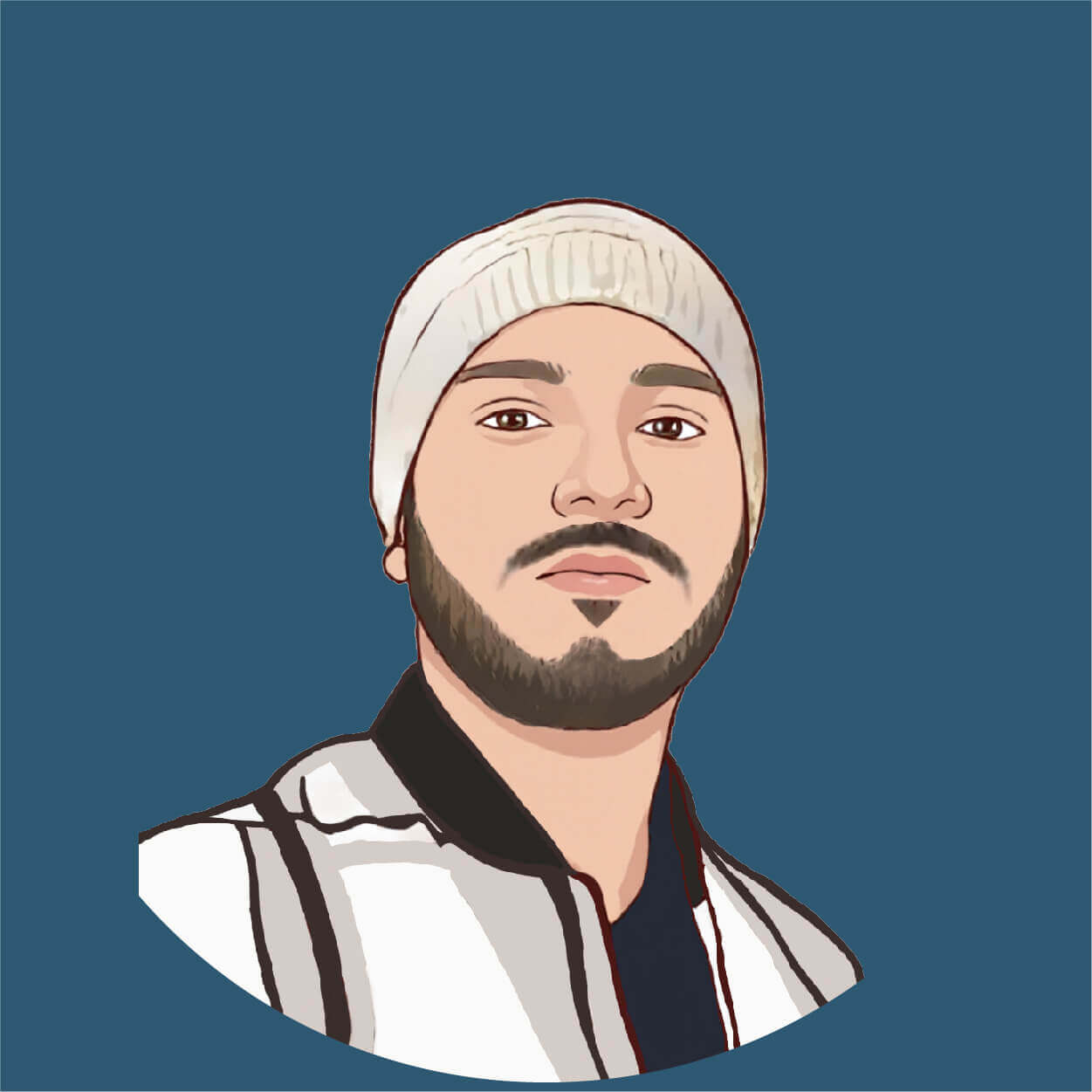
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.