PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
REFERENCES:
A criminal using two names is described as having an alias. In programming, to establish a secondary identifier for a variable, we can create an alias or an alternative name. In C++, a variable serving as an alias for another variable is referred to as a reference variable, or simply a reference.
A reference is essentially another name for an existing variable. Once a reference is initialized with a variable, either the variable's original name or the reference name may be used to access the variable.
In C++, references offer similar capabilities to pointers. While most C++ programmers develop an intuition for when to use references instead of pointers and vice versa, there are still situations where the choice may not be clear. References are often mistaken for pointers, but three significant differences between them are:
- Null references are not allowed; a reference must always be associated with a valid piece of memory.
- Once a reference is initialized to an object, it cannot be reassigned to refer to another object, unlike pointers which can be redirected to point to a different object.
- A reference must be initialized upon creation, whereas pointers can be initialized at any time.
The syntax for declaring a reference closely resembles that of a pointer declaration. However, while a pointer declaration utilizes the *
operator, a reference declaration employs the &
operator. For instance:
int x = 10;
int *ptr = &x;
Here, 'x' is declared as a variable holding a value of 10, and 'ptr' is declared as a pointer initialized with the address of 'x'. Conversely:
int x = 10;
int &ref = x;
In this case, 'ref' is declared as a reference variable of type 'int', referring to 'x'. Although the distinction between pointer and reference declarations is notable, it isn't the primary factor in choosing one over the other. The choice typically depends on the clarity of expressions involving references versus pointers.
While achieving call-by-reference manually using pointer operators is possible, it's often cumbersome. This approach necessitates performing all operations through pointers and remembering to pass addresses rather than values when calling functions.
Fortunately, in C++, it's possible to instruct the compiler to automatically utilize call-by-reference for one or more parameters of a function using reference parameters. With reference parameters, the address (not the value) of an argument is automatically passed to the function. Inside the function, operations on the reference parameter are automatically dereferenced, eliminating the need for pointer operators.
Consider the following example to understand how arguments can be passed by reference to a function:
#include <iostream>
using namespace std;
// Function used to change the value of the actual variable
void increment(int &x) {
++x; // Increment the value
}
int main() {
int x = 10; // Actual variable
int &ptr = x; // Create a reference variable
cout << "Before increment: " << x << endl;
increment(x); // Function calling: call by reference
cout << "After increment: " << x << endl;
return 0;
}
What Happens Inside Call by Reference?
As implied by the term "by reference," we're not passing the value itself but rather a reference or address. To grasp this concept, we can think of variables as names for memory locations. Accessing a variable by its name is essentially accessing the memory location it represents. When we want a called function to modify the value of a variable in the calling function, we must pass the address of that variable to the called function. In summary, when using call-by-reference, we pass memory addresses rather than values.
By using references, we're effectively passing the address of a variable to a function. This approach offers several advantages and disadvantages:
ADVANTAGES:- If a function intends to modify the variable, it alters the original variable rather than a copy, eliminating the need to return values.
- Functions can be designed to modify multiple values, as opposed to returning only a single value.
- Passing variable addresses to functions eliminates the need for copying variables, which saves time.
- The syntax involving '&' and '*' can be cumbersome and more challenging to comprehend than using plain variable names.
- Even if a function doesn't intend to modify a variable, it may inadvertently do so when given access to the variable's address.
- To leverage the benefits of passing addresses to functions while avoiding the drawbacks, reference variables and constant parameters can be used.
In C++, arguments can be passed to functions in three ways:
- Pass by value
- Pass by pointer
- Pass by reference
The default approach is "pass by value." In our upcoming tutorial, we will elaborate on "call by value"and "call by pointer", exploring the intricacies of passing arguments in greater detail.
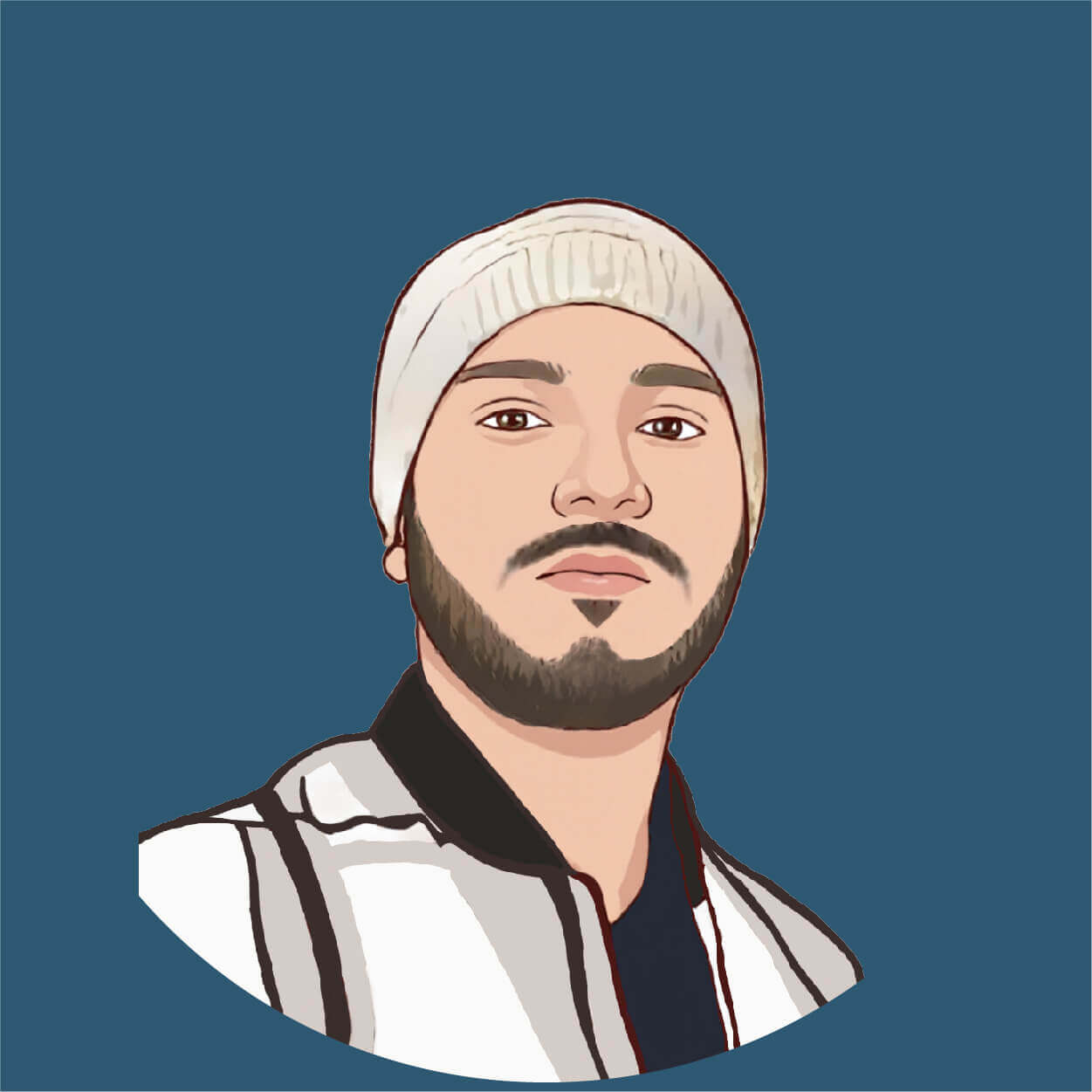
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.