PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Understanding Classes in C++:
A class serves as a user-defined data type reminiscent of a structure.Unlike structures, classes not only include data members but also member functions. These member functions can manipulate data, create and destroy class variables, and even redefine C++ operators to operate on class objects.
Up until now, our programs have largely reflected structured programming rather than Object-Oriented Programming (OOP). To delve into OOP, we need to utilize classes, which are the basic units of encapsulation in C++. Classes facilitate the creation of objects, a fundamental concept in C++ that warrants significant attention throughout this tutorial series.
Consider a simplified example of a class representing a laptop. This class contains properties such as display, hard disk, processor, RAM, color, and operating system (OS), along with methods like power on, power off, processing, video playback, audio playback, and internet access. From this "Laptop" class, we can instantiate multiple objects, each representing a distinct laptop with varying properties but sharing the same methods.
In C++, these properties are referred to as variables, unique to each object, while methods are termed functions, potentially shared across objects.
A class serves as a blueprint defining the structure of an object. It encompasses both code and data, providing instructions on how to construct an object. Notably, a class remains a logical abstraction until an object of that class is instantiated, at which point a physical representation is established in memory.
When defining a class, we specify the data it contains and the operations it can perform. While simple classes may focus solely on code or data, real-world implementations typically combine both. Data is encapsulated within instance variables, while operations are encapsulated within functions, collectively known as class members.
It's worth noting that most C++ compilers come equipped with numerous built-in classes, such as the String classintroduced in a previous tutorial, streamlining string handling within programs.
In essence, a class serves as a blueprint or sketch, outlining properties and methods. Objects, created from these blueprints, share the same methods but possess unique properties, forming the foundation of object-oriented programming.
When defining a class, we create a blueprint for a new, more complex data type. These classes describe objects, detailing their data and associated actions. By grouping related data and functions, classes offer a structured approach to programming. Notably, creating a class automatically generates related fields when objects are instantiated. Moreover, classes facilitate the passage of objects into and out of functions, streamlining data handling. This paradigm allows for intuitive manipulation of objects, akin to real-world entities.
Class definitions start with the keyword class
followed by the class name and enclosed within curly braces {}
. These definitions may contain data members, function members, and access specifiers. Access specifiers determine the visibility of class members and can be public
, private
, or protected
.
The basic syntax for a class is as follows:
class ClassName
{
// Class variables or properties
AccessSpecifier : DataType x;
DataType y;
// Class methods or functions
AccessSpecifier : ReturnType functionName()
{
// Function body
}
};
Determines the visibility of class members. In C++, three types of access specifiers exist:
- Public:Enables access to members from outside the class.
- Private:Restricts access to members outside the class.
- Protected:Limits access to child classes and friend classes.
Keyword initiating class creation, enclosed within curly braces, containing class members.
User-defined identifier for the class.
Specifies variable type (e.g., int, float).
Defines the type of data returned by a method (e.g., int for an integer return type, void for no return).
Following class declaration, class methods and properties are accessed via class objects. Consider the analogy of Facebook: the Facebook class, akin to a blueprint, facilitates the creation of numerous user accounts (objects), each sharing common methods (e.g., login, logout) while possessing distinct properties (e.g., profile picture, status).
class Message
{
// Class members
};
int main()
{
// Creating object
Message msg;
// Accessing class public member using dot operator
msg.display();
return 0;
}
Here, an object msg
of class "Message" is created, and its public member display()
is invoked using the dot .
operator.
Upon class declaration, objects can be instantiated as required. A single object is created as follows:
ClassNameObjectName;
Multiple objects of a single class can be created similarly:
ClassNameObject1, Object2, Object3;
Class methods can be defined within the class definition or externally. Inline definition within the class is achieved by embedding the method within the class definition. Alternatively, external definition mandates declaring the function prototype within the class, followed by defining the function outside the class using the scope resolution operator ::
.
class ClassName
{
public:
// Public method prototype
void display();
};
void ClassName::display()
{
// Function body
}
The scope resolution operator ::
denotes the association between a class member and its class, akin to a family name and a given name.
This tutorial elucidates the concept of classes and objects, the fundamental constituents of object-oriented programming. In subsequent tutorials, we delve deeper into class intricacies and their members.
Every C++ class object inherently possesses four special member functions: default constructor, default destructor, default assignment operator, and copy constructor. These functions facilitate object instantiation, destruction, and manipulation.
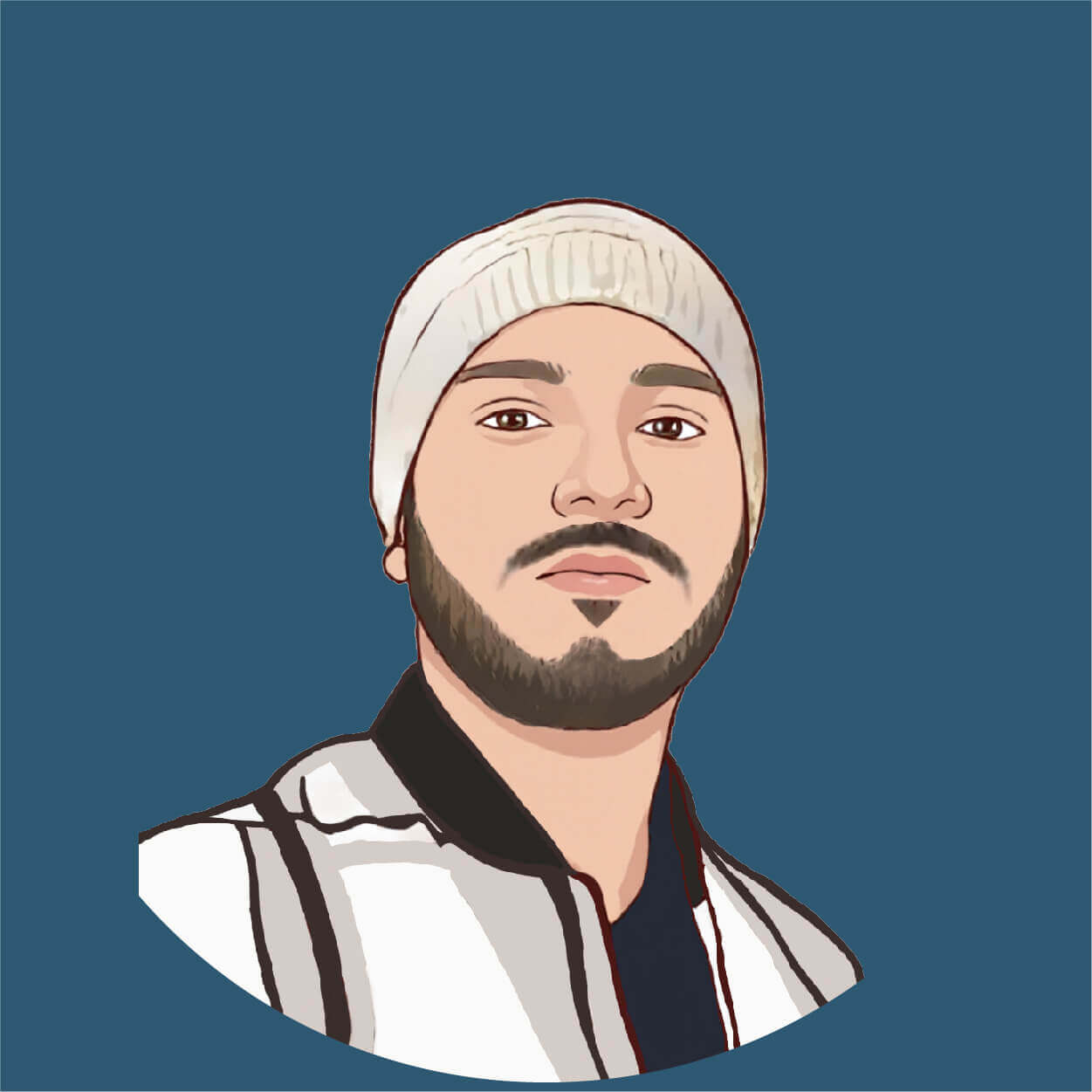
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.