PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Namespace:
Imagine two friends sharing the same name, "Omar," in the same class. To distinguish between them, additional information like their father's name or a nickname is necessary.
Similar situations occur in C++ programs. For instance, if we define a function sort()
in a class and include a library containing another sort()
function with a different implementation, confusion arises. The compiler or linker can't discern which sort()
function to reference within our code, leading to errors.
To resolve this, C++ provides the Namespace Keyword, allowing us to declare our own namespaces.
A namespace is a declarative region that scopes identifiers (names of types, functions, variables, etc.). They organize code logically and prevent name collisions, which commonly occur when multiple libraries are included. Identifiers within a namespace are visible to each other without qualification, while those outside the namespace need full qualification to access members.
Namespace definition starts with the keyword namespace
similar to defining a class.
namespace identifier
{
//namespace body
}
In this syntax:
- namespaceis the keyword to declare a namespace.
- identifiercan be any valid identifier or variable name, following the same naming rules as variables.
- Inside the namespace body, we can define classes, objects, and functions.
For example:
namespace add
{
int a, b;
}
In this example, a
and b
are normal variables within the add
namespace.
Namespace definitions must be at global scope or nested inside another namespace.
Namespace definitions don't terminate with a semicolon, unlike class definitions.
An alias name for a namespace can be used for ease of use. For example:
namespace infoBrotherDotCom
{
void program();
class tutorial{ };
}
// Alias "InfoBrother" for "infoBrotherDotCom"
namespace InfoBrother = infoBrotherDotCom;
Instances of namespaces cannot be created.
There can be unnamed namespaces, unique for each translation unit, acting like named namespaces. For example:
namespace
{
void program();
class tutorial{ };
int x, y;
}
A namespace definition can span multiple files and isn't redefined or overridden.
There are three ways to use a namespace in a program:
Elements in a namespace can be referenced directly within the namespace. Outside the namespace, the namespace must be specified using the scope resolution operator ::
.
For example:
code::x=15; // outside of 'code:'
Consider this example:
/* Namespace using Scope Resolution:*/
#include <string>
#include <iostream>
using namespace std;
namespace MySpace
{
string mess = "Within namespace MySpace";
int count = 0; // Definition: MySpace::count
double f(double); // Prototype: MySpace::f()
}
namespace YourSpace
{
string mess = "Within namespace YourSpace";
void f()
{
mess += '!';
}
}
namespace MySpace
{
int g();
double f(double y)
{
return y / 10.0;
}
}
int MySpace::g()
{
return ++count;
}
int main()
{
cout << "Testing namespaces!\n\n" << MySpace::mess << endl;
MySpace::g();
cout << "\nReturn value g(): " << MySpace::g()
<< "\nReturn value f(): " << MySpace::f(1.2)
<< "\n---------------------" << endl;
YourSpace::f();
cout << YourSpace::mess << endl;
return 0;
}
The using
keyword imports an entire namespace into the program with a global scope. It can be used to import a namespace into another namespace or any program.
For example:
using namespace code;
This statement allows direct referencing of identifiers in the code
namespace. If code
contains another namespace and a using directive, that namespace is also imported.
Consider this example:
/*Namespace using Directive*/
#include <iostream> // Namespace std
void message()
{
std::cout << "Within function ::message()\n";
}
namespace A
{
using namespace std;
void message()
{
cout << "Within function A::message()\n";
}
}
namespace B
{
using std::cout;
void message(void);
}
void B::message(void)
{
cout << "Within function B::message()\n";
}
int main()
{
using namespace std;
using B::message;
cout << "Testing namespaces!\n";
cout << "\nCall of A::message()" << endl;
A::message();
cout << "\nCall of B::message()" << endl;
message();
cout << "\nCall of::message()" << endl;
::message();
return 0;
}
Using Declaration allows importing one specific name at a time into the current scope. Unlike Using Directive, it doesn't import all names in the namespace, limiting their availability to the current scope.
For example:
using code::x;
Consider this example:
/*Namespace using declaration: */
#include <iostream>
using namespace std;
namespace first_num
{
int i;
}
using namespace first_num; //using declaration:
main()
{
i = 2;
cout << "The value is: " << i << endl;
return 0;
}
When using Using Declaration, we don't specify the argument list of a function while importing it. If a namespace has overloaded functions, ambiguity arises.
All entities (variables, types, constants, and functions) of the standard C++ Library are declared within the std
namespace. While using this namespace directly can facilitate comprehension and shorten code, many programmers prefer to qualify each element of the standard library used in their programs.
For example, instead of:
cout<< "Welcome to InfoBrother: "<< endl;
It's common to see:
std::cout<< "Welcome to InfoBrother: "<< std::endl;
The choice between using declarations and full qualification doesn't affect program behavior or efficiency; it's largely a matter of style preference. However, for projects mixing libraries, explicit qualification tends to be preferred.
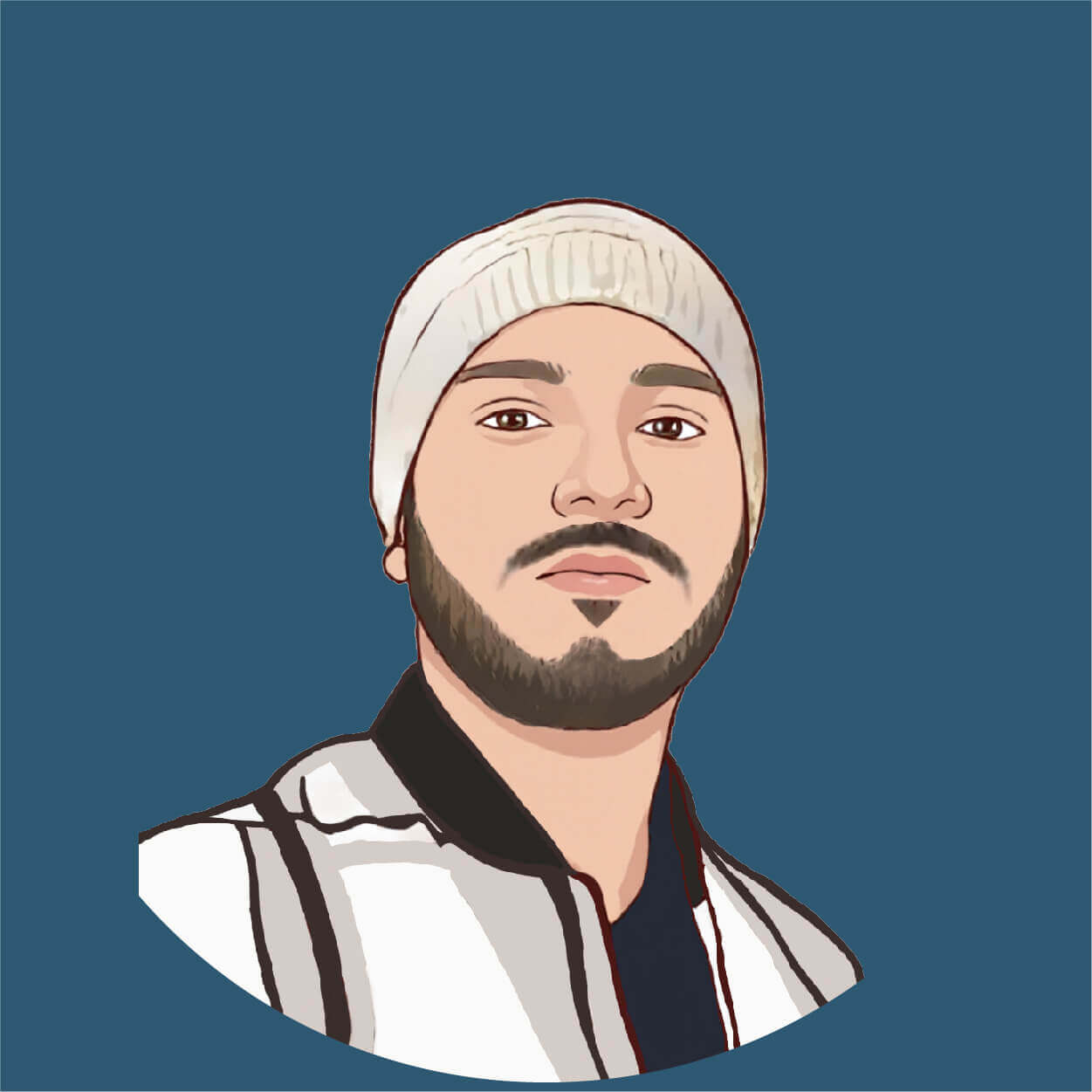
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.