PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Enumeration in C++:
Enumeration, also known as Enumerated type, is a custom data type in C++ that allows the programmer to define a set of named constants. These constants represent the limited values that the enumeration type can hold.
When assigning values to enumerated types, similar to assigning a float value to a character, attempting to assign incompatible values leads to compiler errors. Enumerators, the values within an enumeration, are akin to constants and are assigned integer values by default, starting from 0. These enumerators can be utilized alongside switch statements.
enum season {
spring,
summer,
autumn,
winter
};
In this syntax, "season" is the name of the enumeration, and spring, summer, autumn, and winter are its values.
The final item in the list does not require a comma.
By default, if not specified explicitly, spring is assigned 0, summer is assigned 1, and so forth. You can alter these default values during declaration:
enum season {
spring = 1,
summer = 13,
autumn = 54,
winter = 12
};
Creating an enumeration establishes a blueprint for the variable type. Here's how we declare variables of an enum type:
enum boolean { false, true };
// Inside a function
enum boolean check;
In the above example, a variable check of type enum
boolean is created. Alternatively, the same variable check can be declared using different syntax:
enum boolean
{
false, true
} check;
Let's explore an example where we define a variable named "week" with an enum
type. The expected output of this program is "Day 4". This is because today is Wednesday, equivalent to day 3 when counting from 0. Therefore, adding 1 to today's index should yield 4. To understand how enum types operate, copy and run the code yourself.
#include <iostream>
using namespace std;
enum week { Sunday, Monday, Tuesday,
Wednesday, Thursday, Friday, Saturday
};
int main()
{
week today;
today = Wednesday;
cout << "Day " << today + 1;
return 0;
}
- The
week
enum is defined with values representing each day of the week, starting from 0. - In the
main
function, the variable "today" of type "week" is declared. - It's then assigned the value "Wednesday", which corresponds to an enum value of 3.
- Finally, it prints "Day" followed by today + 1, which results in 4 (since today holds the value Wednesday, which is 3, and adding 1 gives 4).
Enums are frequently employed in switch statementsto assess corresponding values:
Consider the following example where we defines an enumeration Level with constants LOW, MEDIUM, and HIGH. It then declares a variable myVar
of type "Level" and assigns it the value MEDIUM. Finally, it uses a switch statement
to print out the corresponding level based on the value of myVar.
#include <iostream>
using namespace std;
enum Level {
LOW = 1,
MEDIUM,
HIGH
};
int main() {
enum Level myVar = MEDIUM;
switch (myVar) {
case LOW:
cout << "Low Level";
break;
case MEDIUM:
cout << "Medium level";
break;
case HIGH:
cout << "High level";
break;
}
return 0;
}
Why and When to Use Enums?
Enums are beneficial for naming constants, enhancing code readability and maintainability. They are particularly useful for values expected to remain constant, such as month names, days of the week, colors, or a deck of cards.
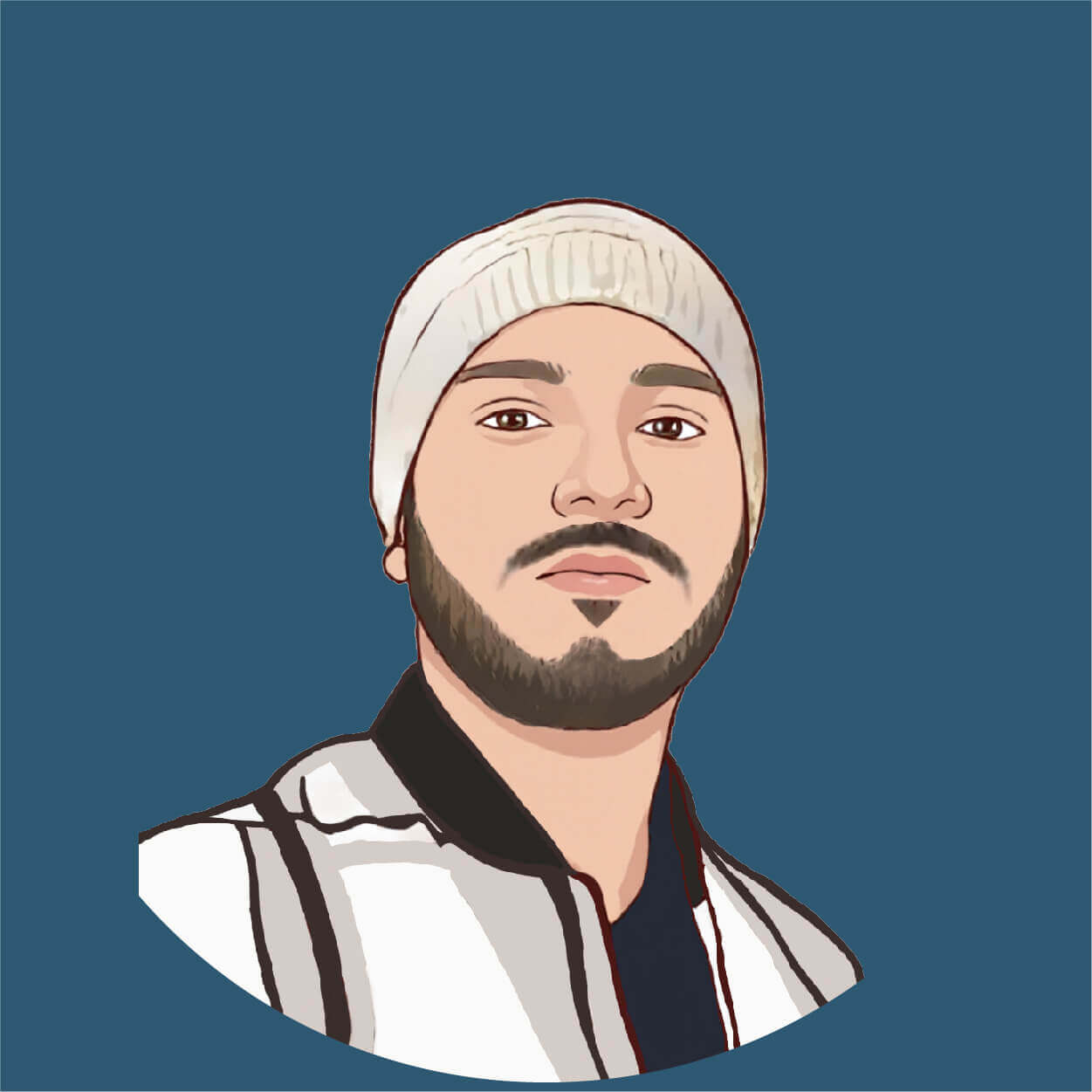
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.