PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
WHILE LOOP:
A while loop statement repeatedly executes a target statement as long as a given condition is true. In a while loop, a Boolean expression is evaluated. If the expression in the while statement is true, the resulting action, called the Loop Body, executes, and the Boolean expression is tested again. If it is false, the loop is terminated, and program execution continues with the statement that follows the while statement. This cycle of execute-test-execute-test continues as long as the Boolean expression remains true.
The syntax of the while loop is as follows:
while (condition)
{
/* Block of one or more C++ statements to be executed
if the condition is true. */
}
The parentheses ( )
around "condition" are required. As long as "condition" is true, the block of one or more C++ statements executes repeatedly until the condition becomes false. Braces {}
are necessary before and after the body of the while loop unless only one statement is to be executed. Each statement in the body of the while loop requires an ending semicolon.
The placeholder "condition" usually contains relational, and possibly logical, operators. These operators provide the true-false condition checked in condition
. If "condition" becomes false at the beginning of the while loop, the body of the while loop does not execute at all because the first "condition" will be tested. If the condition is true, the loop body will be executed; otherwise, there is nothing to execute in the loop body if the condition becomes false.
If the body of the while loop contains only one statement, the braces surrounding it are not required, but it is good programming practice to enclose all while loop statements in braces.
Let's consider a simple example to understand how a while loop works. In this example, we will create a simple guessing game. We will hide a number and ask our friend to guess the number. This loop will execute repeatedly until your friend guesses the right number.
#include <iostream>
using namespace std;
int main()
{
int number = 55; // Number to be guessed
int guess; // User's guess will be stored here
cout << "Guess the Number: ";
cin >> guess;
// The loop will execute until the guess is not equal to 55
while (guess != number)
{
cout << "Wrong Guess...!!" << endl;
cout << "\nGuess again: ";
cin >> guess;
}
// If the user guesses the right number, this message will be displayed
cout << "Congratulations! You've guessed the right number." << endl;
return 0;
}
Let's consider another useful example: in this example, the program will ask to enter an Upper Limit (any number) and will add all numbers till the Upper Limit and give you the sum. For example, if you enter "5" as the upper limit, then it will add 0+1+2+3+4+5 and give you the sum (i.e., 15). It will start from 0 and will end at the upper limit.
#include<iostream>
using namespace std;
int main()
{
int UpperLimit; // To get Upper Limit from User.
int sum = 0; // Will be used to store the Sum of Numbers.
int Number = 0; // All Numbers less than the upper limit.
cout << "Enter the Upper Limit to get the sum: ";
cin >> UpperLimit;
/* Loop will continue until reaching the Upper Limit Number. */
// Number is less than the Upper Limit.
while (Number < UpperLimit)
{
Number = Number + 1; // Increment number one by one.
// All numbers will be added to sum and will be stored in the sum variable.
sum = sum + Number;
// Example. sum = 0+1=1; sum = 1+1=2 and so on.
}
/* When the condition becomes false, meaning Number
will reach the upper limit. */
cout << "The sum of the first " << UpperLimit << " numbers is: " << sum;
return 0;
}
Be careful while using loops. Using the wrong condition can lead to an infinite loop. Always set a limit for the loop; otherwise, the loop's condition will always be true, resulting in an infinite loop. Here is an example: copy and paste this code into your compiler and observe how an infinite loop works.
In this example, the program will ask to enter the starting number. You can input any number, and the loop will become an infinite loop because the condition is "Number >0." Obviously, all positive numbers are greater than 0, so there is no limit to terminate the program. Therefore, the condition will always be true, and the program will continue indefinitely.
#include<iostream>
using namespace std;
int main()
{
int Number = 0; // To get the starting Number from the user.
cout << "Please enter the starting Number: ";
cin >> Number;
// While Number is greater than zero, meaning there is no limit.
while (Number > 0)
{
cout << Number << ",";
++Number; // Increment Number.
}
return 0;
}
Any C++ expression can be placed inside the required parentheses in the while statement. As with the ifstatement, if the expression evaluates to false or zero, then the loop body is not entered. If the expression within the parentheses evaluates to true or any non-zero value, the loop body executes. With a while statement, when the expression is evaluated as true, the statements that follow execute repeatedly as long as the expression remains true.
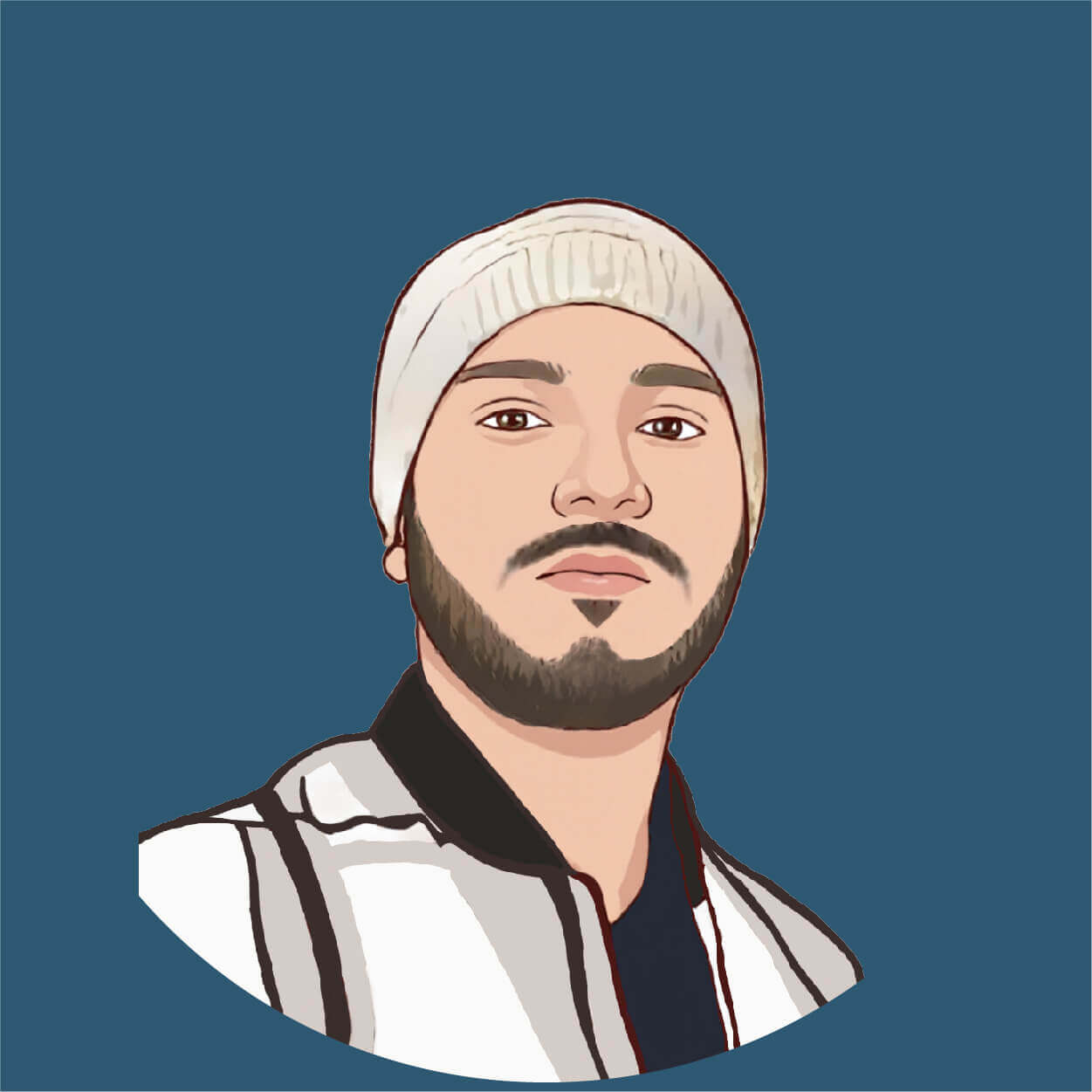
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.