PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Dynamic Memory Allocation Using realloc():
In our previous tutorials, we discussed dynamic memory allocation using the calloc()function. Now, we'll explore how to dynamically allocate memory using the realloc()
function from the C library <stdlib>
.
Let's consider a scenario: we've written a program to store student data. Initially, our program allocates memory for 20 students. But what if we need to add 5 more students? Since our current functions can't allocate memory for additional students, should we terminate the program and start over to allocate enough memory? The answer is no. The C library provides another function, realloc()
, to resize allocated memory.
Reallocation occurs in two ways:
Expanding or contracting the existing area pointed to by the pointer, if possible. The contents of the area remain unchanged up to the lesser of the new and old sizes. If the area is expanded, the contents of the new part of the array are undefined.
Allocating a new memory block of size new_size
bytes, copying the memory area with a size equal to the lesser of the new and old sizes, and freeing the old block.
If there isn't enough memory, the old memory block remains unfreed, and a null pointer is returned.
We can only use this function if memory is dynamically allocated. If memory isn't dynamically allocated, the behavior is undefined.
The basic syntax is:
void* realloc(void *ptr, size_t new_size);
In the above syntax, ptr
is the pointer to the old memory that needs resizing, and new_size
is the new size to be allocated. If realloc()
fails to allocate new memory, the old memory won't be freed, and a null pointer will be returned.
If we need the pointer to return any other data type instead of void, we can use the type cast operator. The syntax is:
cast_type *ptr; // pointer to data type
ptr = (cast_type *)realloc(ptr, size);
For Example:
int *ptr;
ptr = (int *)realloc(ptr, num * sizeof(int));
In the above example, we usesizeof()operator to allocate exact memory in bytes, as realloc() requires the size of elements in bytes.
Now, let's look at an example to understand how to reallocate memory using realloc() function:
/* Example: realloc() function*/
#include<iostream>
#include<stdlib.h> // for realloc and malloc function.
#include<string.h> // for string function.
using namespace std;
main() {
char *ptr;
/* Initial memory allocation */
ptr = (char *) malloc(20);
if(ptr != NULL) {
strcpy(ptr, "InfoBrother");
cout << "The string is: " << ptr << " and the Address is: "
<< &ptr << endl;
}
else
cout << "Error while allocating memory." << endl;
/*********************************************/
/* Reallocating memory */
ptr = (char *) realloc(ptr, 25);
if(ptr != NULL) {
strcat(ptr, ".com");
cout << "String is: " << ptr << " and address is: " << &ptr
<< endl;
}
else
cout << "Error while allocating Memory." << endl;
free(ptr); // free the memory;
}
In the above example, we allocate memory for the string "InfoBrother" using malloc()
function. Later, realizing that ".com" is missing, we reallocate memory for "InfoBrother.com" using realloc()
function. We also include error-checking code in our program and, responsibly, free the allocated space at the end. This example illustrates the concept of the realloc()
function.
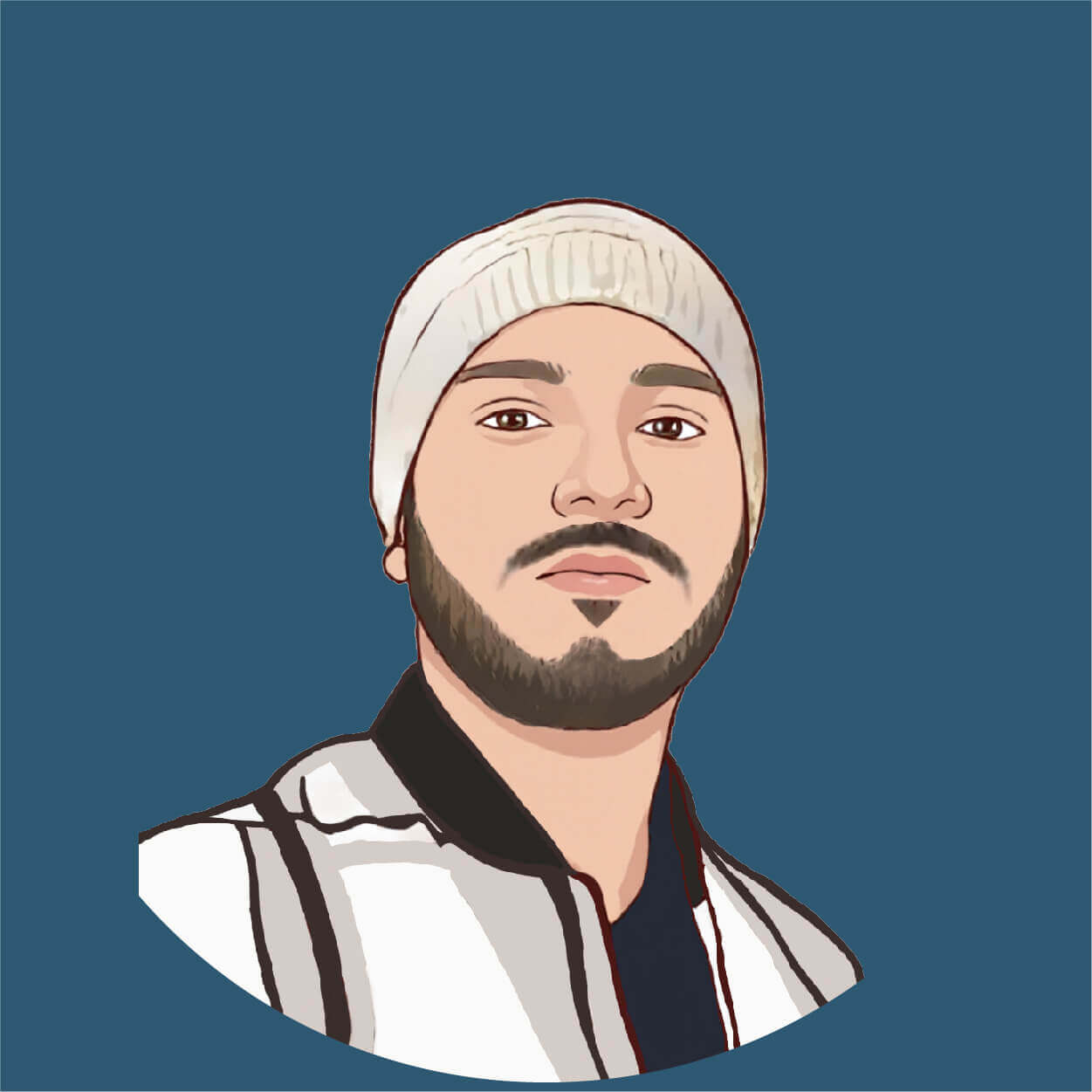
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.