PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
Constructor:
A constructor is a special method of a class that is automatically invoked whenever an object of the class is created. Constructors are responsible for initializing objects and allocating memory for the class. If a class is created without a constructor, the compiler automatically generates a default constructor for that class. However, the default constructor does not perform any initialization, so it's necessary to create custom constructors for proper initialization.
When we declare a variable, such as an integer, without initializing it, the variable contains garbage values. This can lead to unexpected behavior in the program. Similarly, when we create an object of a class, if the class properties are not initialized, they may contain garbage values. Constructors help in preventing this by ensuring proper initialization of objects.
A constructor has the same name as the class and does not have a return type. It is automatically invoked when an object is created and does not need to be called explicitly in the main method. Let's look at an example to understand how constructors are defined and how they work.
#include<iostream>
using namespace std;
class Constructor {
public:
void display() {
cout << "Hi, did you call the constructor?";
}
Constructor() {
cout << "You didn't call me, but I'm here." << endl;
cout << "Surprised?" << endl;
}
};
int main() {
Constructor obj;
obj.display();
return 0;
}
In the above example, the constructor is defined but not explicitly called in the main program. However, it executes automatically when an object of the class is created.
If we specify a constructor in our class, the program will exclusively utilize that constructor. Otherwise, the default constructor will automatically function.
In C++, there are four types of constructors:
A default constructor does not have any parameters and initializes the instance of the class without requiring any input, as shown in the example below:
#include<iostream>
using namespace std;
class Def_Constructor {
public:
int x, y;
Def_Constructor() {
x = 15;
y = 10;
}
};
int main() {
Def_Constructor obj;
cout << "The value of X is: " << obj.x << endl;
cout << "The value of Y is: " << obj.y << endl;
return 0;
}
A parameterized constructor accepts at least one parameter and allows initialization of class instances with different values, as demonstrated in the example below:
#include<iostream>
using namespace std;
class Par_Constructor {
public:
int x, y;
Par_Constructor(int a, int b) {
x = a;
y = b;
}
};
int main() {
Par_Constructor obj1(12, 15);
cout << "First Object:" << endl;
cout << "The value of X is: " << obj1.x << endl;
cout << "The value of Y is: " << obj1.y << endl;
Par_Constructor obj2(44, 65);
cout << "\nSecond Object:" << endl;
cout << "The value of X is: " << obj2.x << endl;
cout << "The value of Y is: " << obj2.y << endl;
return 0;
}
A copy constructor initializes an object using another object of the same class. It is useful for creating a new object with the same values as an existing object, as shown below:
#include<iostream>
using namespace std;
class Copy_Constructor {
public:
int x, y;
Copy_Constructor(int a, int b) {
x = a;
y = b;
}
Copy_Constructor(const Copy_Constructor &C_obj) {
x = C_obj.x;
y = C_obj.y;
}
};
int main() {
Copy_Constructor obj1(12, 15);
cout << "Using Normal Constructor:" << endl;
cout << "The value of X is: " << obj1.x << endl;
cout << "The value of Y is: " << obj1.y << endl;
Copy_Constructor obj2(obj1);
cout << "\nUsing Copy Constructor:" << endl;
cout << "The value of X is: " << obj2.x << endl;
cout << "The value of Y is: " << obj2.y << endl;
return 0;
}
A private constructor restricts object creation for other classes and methods, except friend classes. It is useful for scenarios where object instantiation needs to be controlled, as shown below:
#include<iostream>
using namespace std;
class Private_Constructor {
private:
Private_Constructor() {
cout << "This is a private Constructor." << endl;
}
friend class frnd;
};
class frnd {
public:
frnd() {
cout << "Friend Class:" << endl;
}
};
int main() {
frnd obj1;
return 0;
}
A destructor is a special member of a class that is automatically called when an object is destroyed. It is used to release memory resources before the program exits. Unlike constructors, destructors cannot be explicitly invoked.
#include<iostream>
using namespace std;
class House {
public:
House(int price) {
cout << "House Created Successfully:" << endl;
cout << "Price: $" << price << endl;
}
~House() {
cout << "Oops!! House Destroyed:" << endl;
}
};
int main() {
House obj(1030000);
return 0;
}
Destructors automatically release resources when objects go out of scope or when the program exits. They cannot be overloaded, inherited, or used with structs. It's important to use destructors, especially when dealing with pointers and dynamic memory allocation.
Programmers often have fewer tasks to perform in destructors compared to constructors. Generally, code in a destructor is necessary when an object contains a pointer to a member. We use a destructor to ensure we don't retain a pointer pointing to an object that no longer exists.
- Destructors are invoked automatically and cannot be invoked explicitly.
- Destructors cannot be overloaded; therefore, a class can have at most one destructor.
- Destructors are not inherited; thus, a class has no destructors other than the one declared in it.
- Destructors cannot be used with structs; they are only used with classes.
- An instance becomes eligible for destruction when it is no longer possible for any code to use the instance.
- Execution of the destructor for the instance may occur at any time after the instance becomes eligible for destruction.
- A destructor does not take modifiers or have parameters.
constructors and destructors play crucial roles in managing object creation, initialization, and memory management in C++ programs. Understanding their usage and implementing them appropriately ensures efficient and reliable program execution.
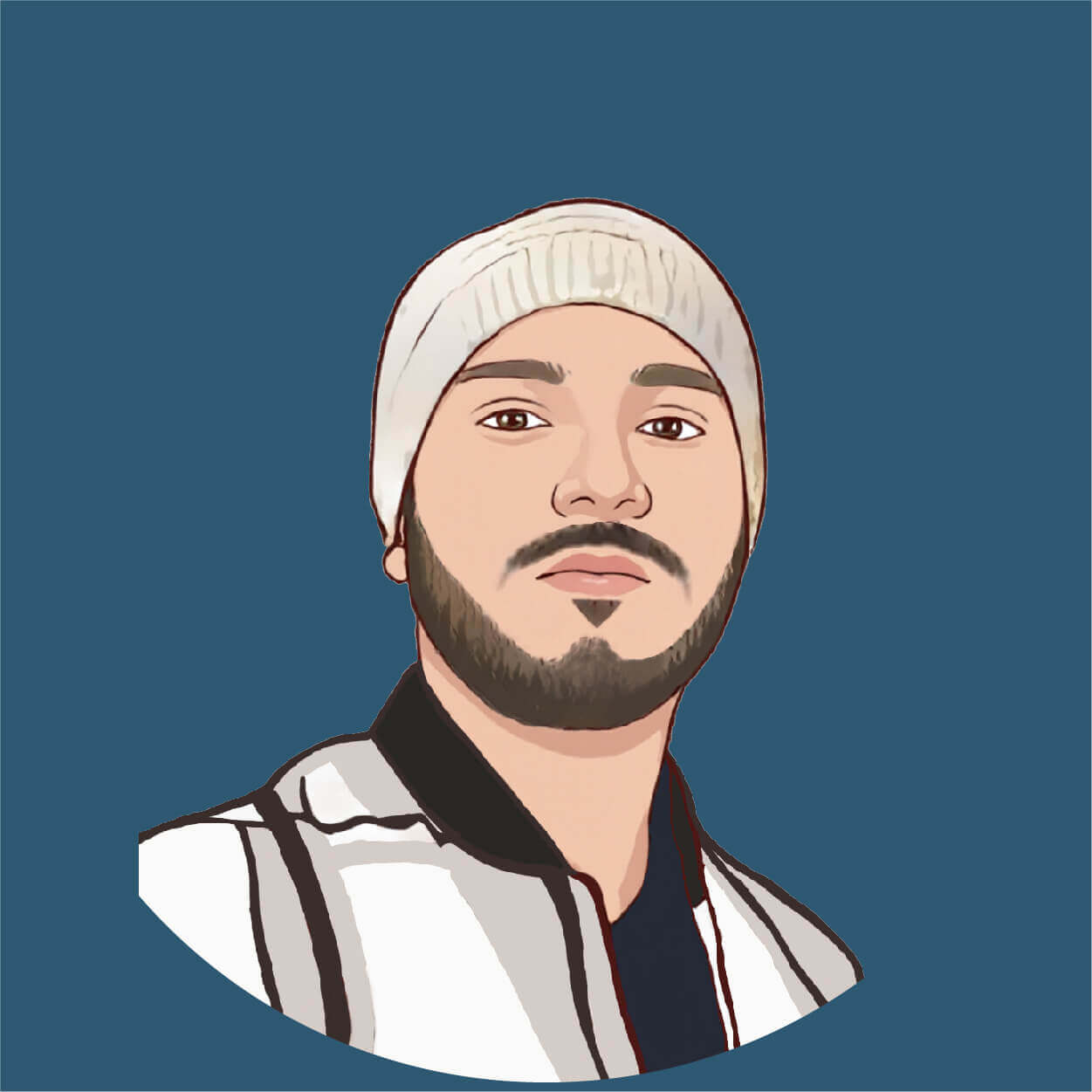
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.