PDFVersion
No ads? No problem! Download the PDF book of this tutorial for just $24.99. Your support will help us reach more readers.
Thank You!
TYPES OF INHERITANCE:
There are five types of inheritance based on the ways of inheriting the features of a base class into a derived class:
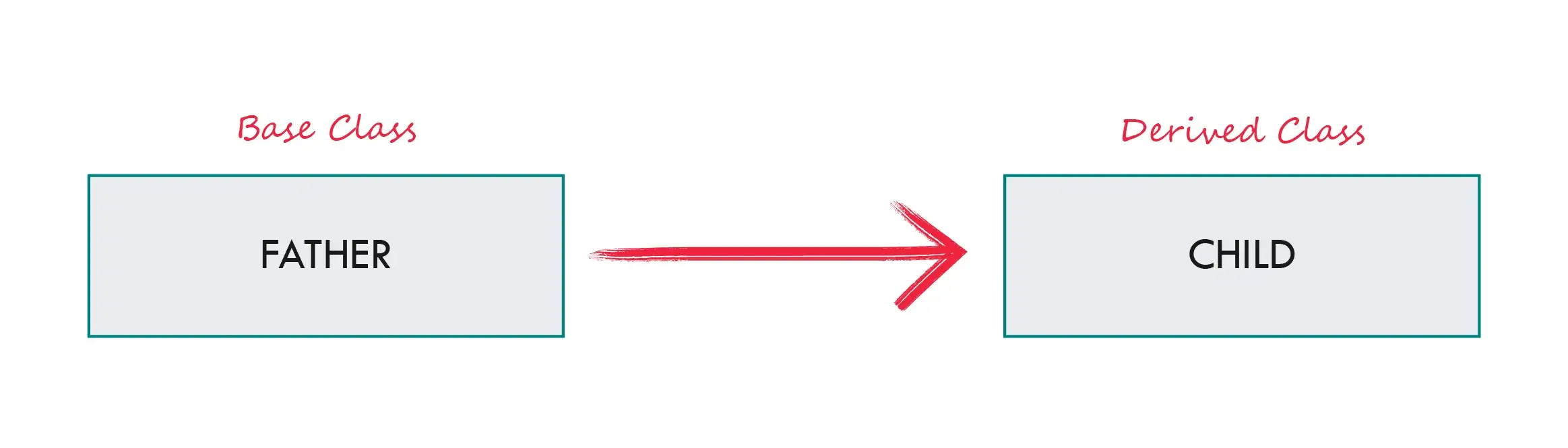
Single Inheritance involves a single base
class and a single derived
class. It allows the derived class to inherit properties and behavior from a single parent class, facilitating code reuse and enabling the addition of new features to existing code. This enhances code elegance and reduces repetition.
class Base_Class
{
// Class Body:
}
class Derived_Class: Access-Specifier Base_Class
{
//Class Body:
}
In the syntax above:
- Access-Specifier defines the scope and visibility of a class.
class
is the keyword to define a class.- Base_Class is the name of the class whose members will be inherited by the derived class.
- Derived_Class is the name of the class which will inherit the members of Base_Class.
- Colon
:
is the special character to establish a relationship between the base and derived class.
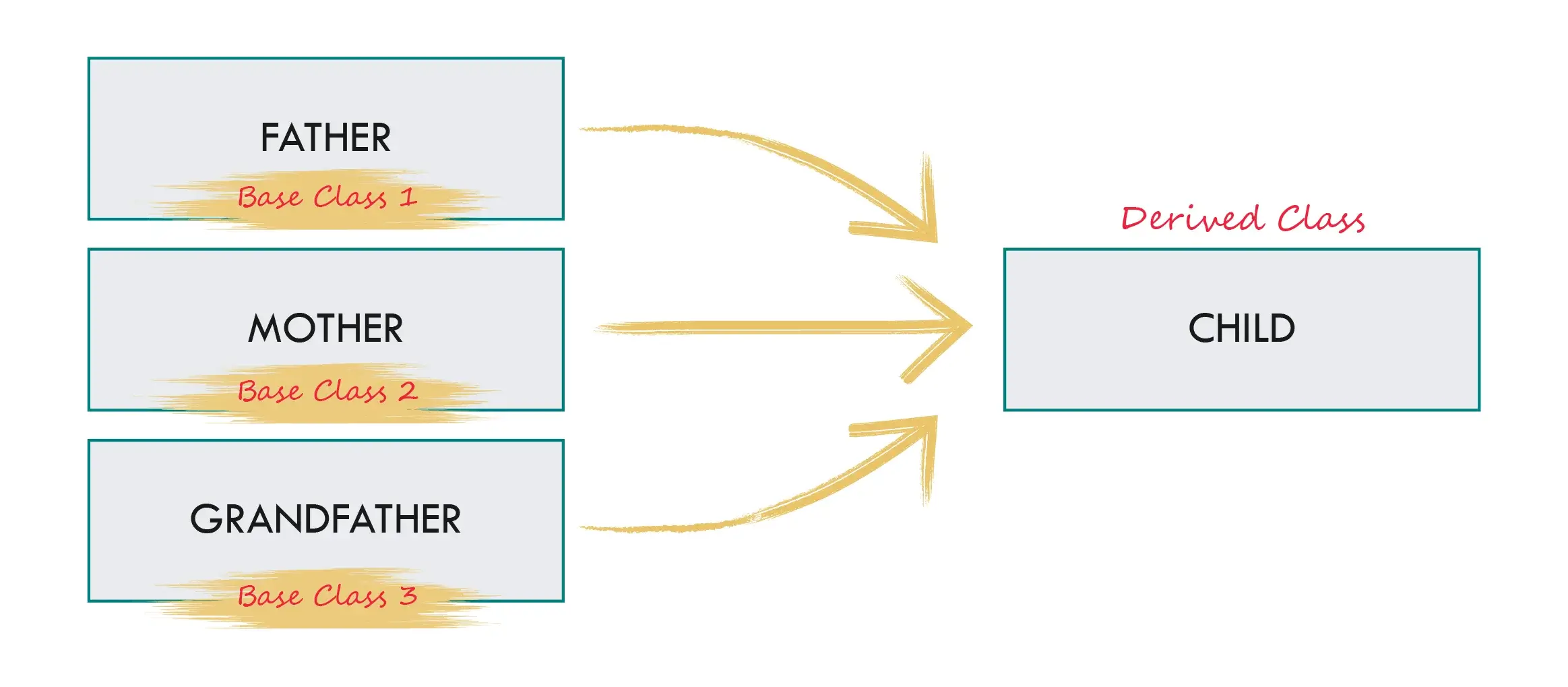
Multiple Inheritance occurs when a derived
class inherits properties from multiple base
classes. It allows a class to inherit from more than one class, as demonstrated in the extended syntax below:
class A
{
// Base Class Body:
}
class B
{
// Base Class Body:
}
class C : Access-Specifier A, Access-Specifier B
{
// Derived Class Body:
}
In the syntax above:
- Access-Specifier defines the scope and visibility of a class.
class
is the keyword to define a class.- Base_Class is the name of the class whose members will be inherited by the derived class.
- Derived_Class is the name of the class which will inherit the members of Base_Class.
- Colon
:
is the special character to establish a relationship between the base and derived class. - Class A is the base class of Class C.
- Class B is the base class of Class C.
- The derived class "C" inherits the properties and methods of both classes A and B using the comma operator.
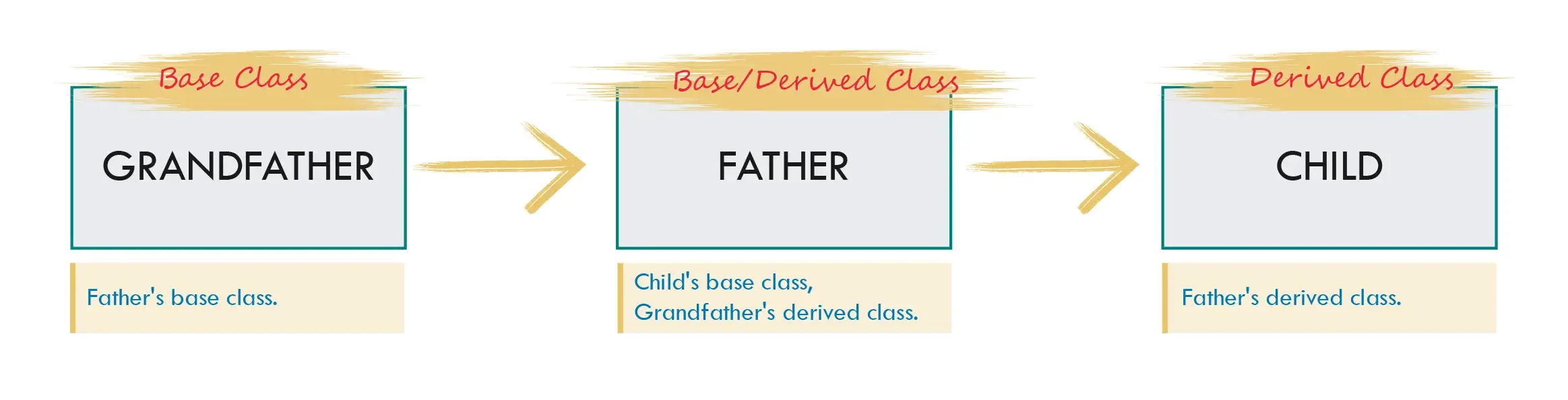
Multilevel Inheritance occurs when a derived
class serves as the base
class for another class. It involves deriving a class from a derived class, as shown in the example where Class Grandfather is the parent of Class Father, and Class Father is the parent of Class Child.
class GrandFather // Base Class
{
// Class Body
};
// Derived Class from GrandFather Class
class Father : Access-Specifier GrandFather
{
// Class Body
};
// Derived Class from Father Class
class Child : Access-Specifier Father
{
// Class Body
};
In the syntax above:
- Access-Specifier defines the scope and visibility of a class.
class
is the keyword to define a class.- GrandFather is the base class for Class Father.
- Father is the derived class from Class GrandFather and the base class of Child.
- Child is the derived class from Father Class.
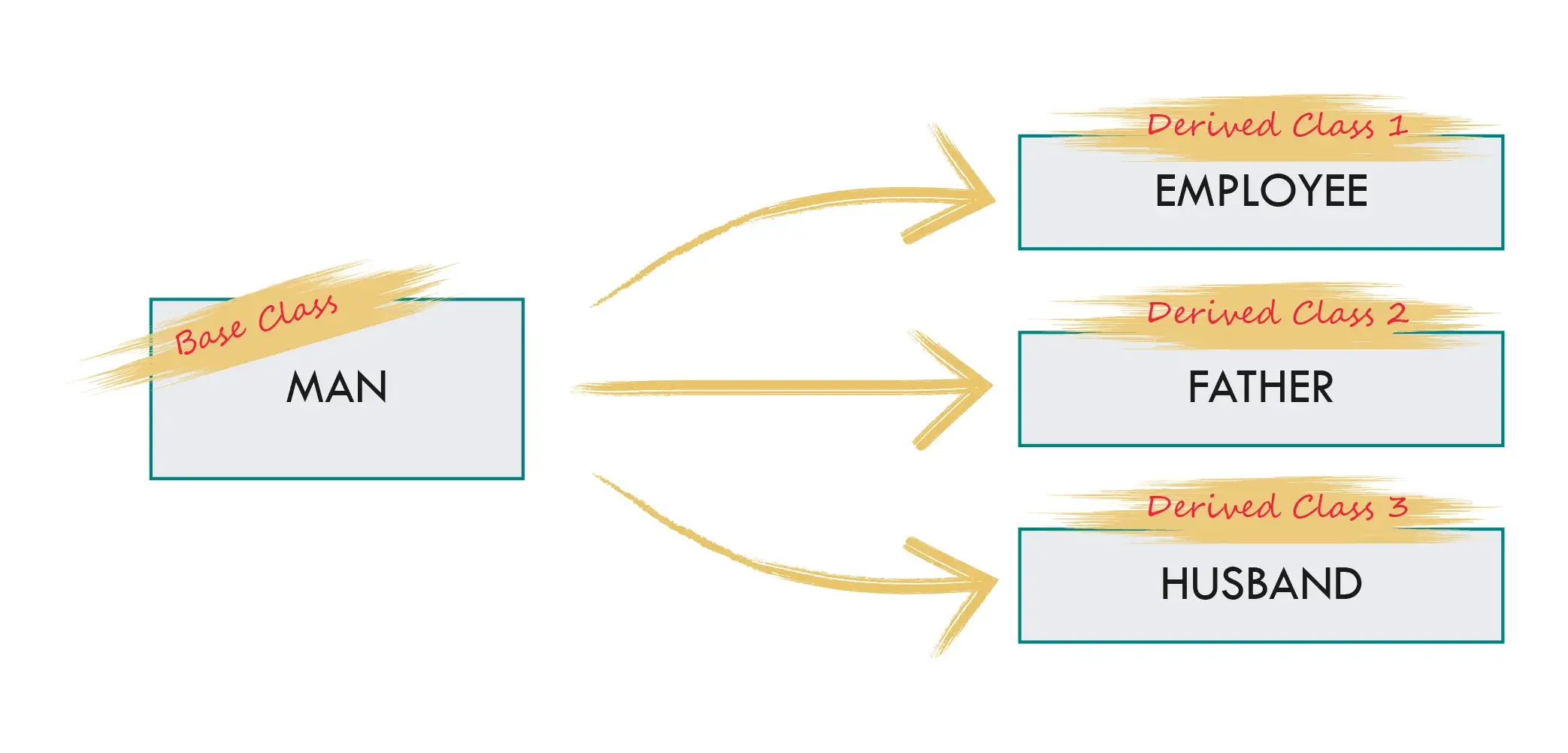
Hierarchical Inheritance involves creating multiple derived
classes that inherit properties from the same base
class. For example, Civil, Computer, Mechanical, and Electrical are derived from Engineer.
class MAN // Base Class
{
// Class Body
};
// Derived Class from MAN
class Employee : Access-Specifier MAN
{
// Class Body
};
// Derived Class from MAN
class Father : Access-Specifier MAN
{
// Class Body
};
// Derived Class from MAN
class Husband : Access-Specifier MAN
{
// Class Body
};
In the syntax above:
- Access-Specifier defines the scope and visibility of a class.
- MAN is the base class for all other classes.
- Employee, Father, and Husband are derived classes of the MAN class.
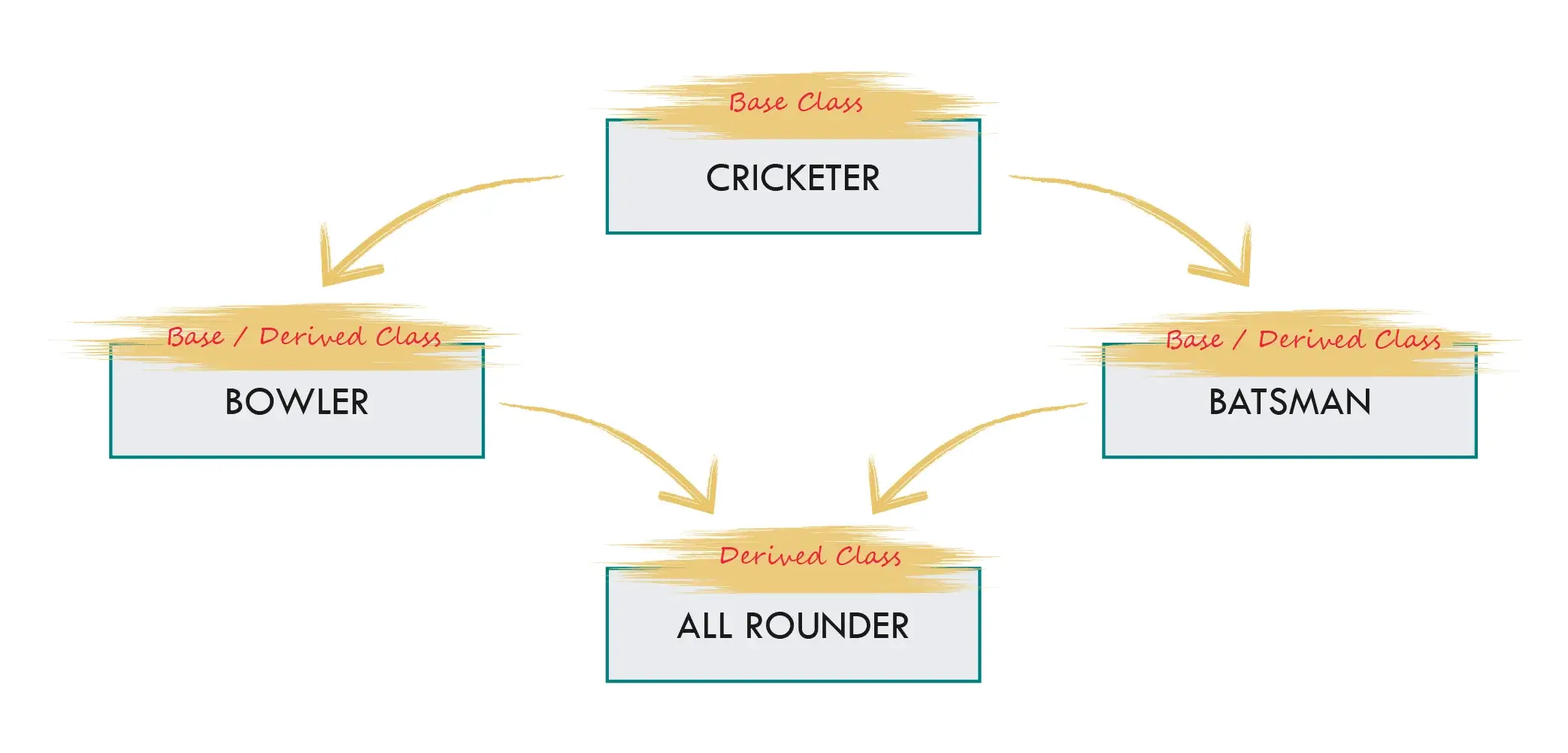
Hybrid Inheritance combines multilevel and hierarchical inheritance. It's also known as Virtual Inheritance.
// Base Class for Batsman and Bowler.
class Cricketer
{
// Class Body
}
// Derived Class of Cricketer and base class for All-rounder.
class Batsman : Access-Specifier Cricketer
{
// Class Body
}
// Derived Class of Cricketer and base class for All-rounder.
class Bowler : Access-Specifier Cricketer
{
// Class Body
}
// Derived Class of Batsman and Bowler.
class All-rounder : Access-Specifier Batsman , Access-Specifier Bowler
{
// Class Body
}
In the syntax above:
- Access-Specifier defines the scope and visibility of a class.
- Cricketer is the base class for Batsman and Bowler.
- Batsman is the derived class of Cricketer and the base class for All-rounder.
- Bowler is the derived class of Cricketer and the base class for All-rounder.
- All-rounder is the derived class of Batsman and Bowler.
When developing an application using inheritance, the following advantages are obtained:
- Faster application development
- Reduced memory consumption
- Faster execution time
- Improved application performance
- Reduction in code repetition, leading to consistent results and lower storage costs.
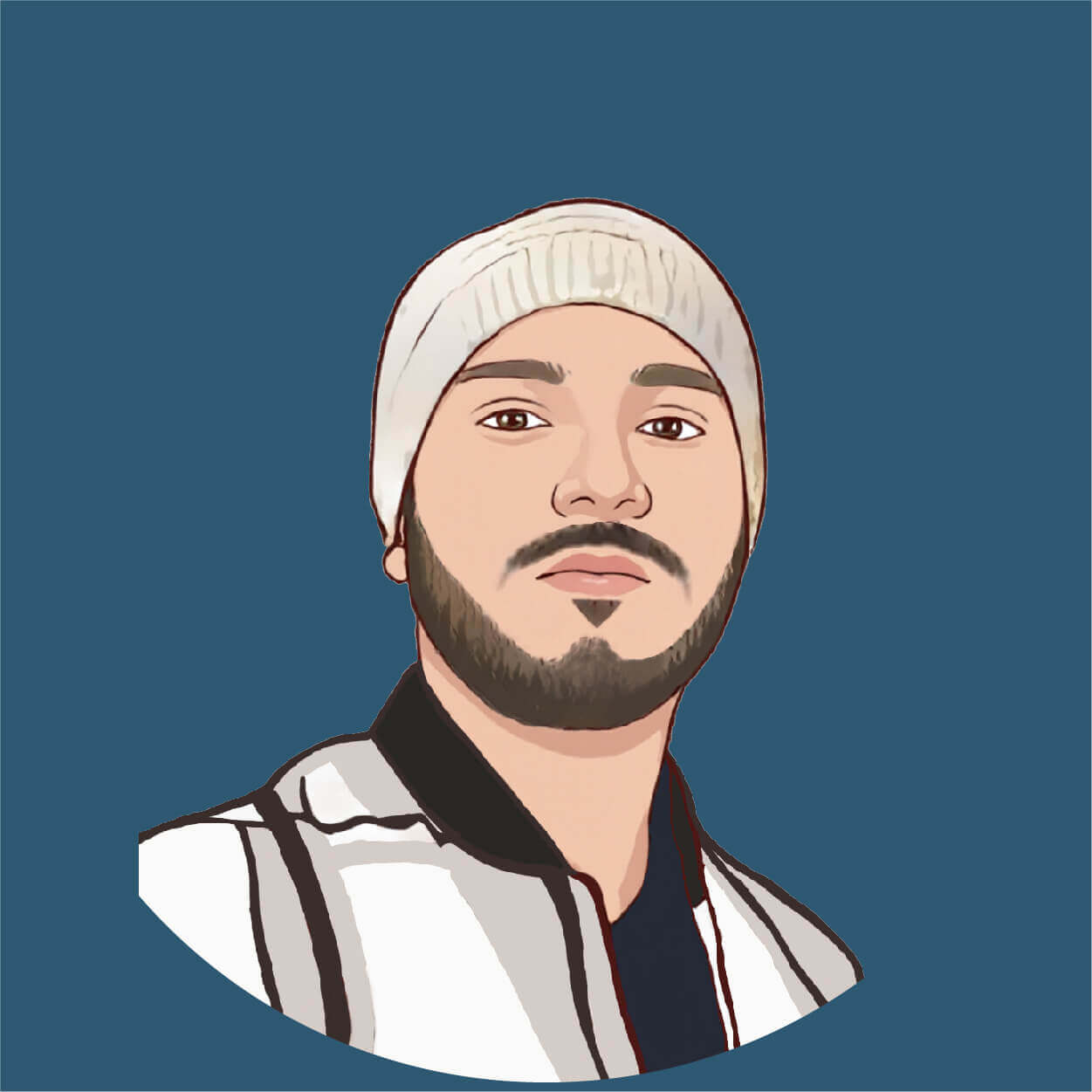
Sardar Omar
I did my hardest to present you with all of the information you need on this subject in a simple and understandable manner. However, if you have any difficulties understanding this concept or have any questions, please do not hesitate to ask. I'll try my best to meet your requirements.